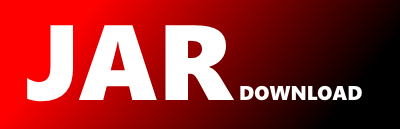
com.pulumi.gcp.compute.kotlin.outputs.RegionInstanceTemplateScheduling.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property automaticRestart Specifies whether the instance should be
* automatically restarted if it is terminated by Compute Engine (not
* terminated by a user). This defaults to true.
* @property instanceTerminationAction Describe the type of termination action for `SPOT` VM. Can be `STOP` or `DELETE`. Read more on [here](https://cloud.google.com/compute/docs/instances/create-use-spot)
* @property localSsdRecoveryTimeouts Specifies the maximum amount of time a Local Ssd Vm should wait while
* recovery of the Local Ssd state is attempted. Its value should be in
* between 0 and 168 hours with hour granularity and the default value being 1
* hour.
* @property maintenanceInterval Specifies the frequency of planned maintenance events. The accepted values are: PERIODIC
* @property maxRunDuration The duration of the instance. Instance will run and be terminated after then, the termination action could be defined in `instance_termination_action`. Only support `DELETE` `instance_termination_action` at this point. Structure is documented below.
* @property minNodeCpus Minimum number of cpus for the instance.
* @property nodeAffinities Specifies node affinities or anti-affinities
* to determine which sole-tenant nodes your instances and managed instance
* groups will use as host systems. Read more on sole-tenant node creation
* [here](https://cloud.google.com/compute/docs/nodes/create-nodes).
* Structure documented below.
* @property onHostMaintenance Defines the maintenance behavior for this
* instance.
* @property preemptible Allows instance to be preempted. This defaults to
* false. Read more on this
* [here](https://cloud.google.com/compute/docs/instances/preemptible).
* @property provisioningModel Describe the type of preemptible VM. This field accepts the value `STANDARD` or `SPOT`. If the value is `STANDARD`, there will be no discount. If this is set to `SPOT`,
* `preemptible` should be `true` and `automatic_restart` should be
* `false`. For more info about
* `SPOT`, read [here](https://cloud.google.com/compute/docs/instances/spot)
*/
public data class RegionInstanceTemplateScheduling(
public val automaticRestart: Boolean? = null,
public val instanceTerminationAction: String? = null,
public val localSsdRecoveryTimeouts: List? = null,
public val maintenanceInterval: String? = null,
public val maxRunDuration: RegionInstanceTemplateSchedulingMaxRunDuration? = null,
public val minNodeCpus: Int? = null,
public val nodeAffinities: List? = null,
public val onHostMaintenance: String? = null,
public val preemptible: Boolean? = null,
public val provisioningModel: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.RegionInstanceTemplateScheduling): RegionInstanceTemplateScheduling = RegionInstanceTemplateScheduling(
automaticRestart = javaType.automaticRestart().map({ args0 -> args0 }).orElse(null),
instanceTerminationAction = javaType.instanceTerminationAction().map({ args0 ->
args0
}).orElse(null),
localSsdRecoveryTimeouts = javaType.localSsdRecoveryTimeouts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.RegionInstanceTemplateSchedulingLocalSsdRecoveryTimeout.Companion.toKotlin(args0)
})
}),
maintenanceInterval = javaType.maintenanceInterval().map({ args0 -> args0 }).orElse(null),
maxRunDuration = javaType.maxRunDuration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.RegionInstanceTemplateSchedulingMaxRunDuration.Companion.toKotlin(args0)
})
}).orElse(null),
minNodeCpus = javaType.minNodeCpus().map({ args0 -> args0 }).orElse(null),
nodeAffinities = javaType.nodeAffinities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.RegionInstanceTemplateSchedulingNodeAffinity.Companion.toKotlin(args0)
})
}),
onHostMaintenance = javaType.onHostMaintenance().map({ args0 -> args0 }).orElse(null),
preemptible = javaType.preemptible().map({ args0 -> args0 }).orElse(null),
provisioningModel = javaType.provisioningModel().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy