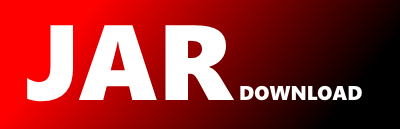
com.pulumi.gcp.compute.kotlin.outputs.URLMapPathMatcherRouteRuleMatchRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property fullPathMatch For satisfying the matchRule condition, the path of the request must exactly
* match the value specified in fullPathMatch after removing any query parameters
* and anchor that may be part of the original URL. FullPathMatch must be between 1
* and 1024 characters. Only one of prefixMatch, fullPathMatch or regexMatch must
* be specified.
* @property headerMatches Specifies a list of header match criteria, all of which must match corresponding
* headers in the request.
* Structure is documented below.
* @property ignoreCase Specifies that prefixMatch and fullPathMatch matches are case sensitive.
* Defaults to false.
* @property metadataFilters Opaque filter criteria used by Loadbalancer to restrict routing configuration to
* a limited set xDS compliant clients. In their xDS requests to Loadbalancer, xDS
* clients present node metadata. If a match takes place, the relevant routing
* configuration is made available to those proxies. For each metadataFilter in
* this list, if its filterMatchCriteria is set to MATCH_ANY, at least one of the
* filterLabels must match the corresponding label provided in the metadata. If its
* filterMatchCriteria is set to MATCH_ALL, then all of its filterLabels must match
* with corresponding labels in the provided metadata. metadataFilters specified
* here can be overrides those specified in ForwardingRule that refers to this
* UrlMap. metadataFilters only applies to Loadbalancers that have their
* loadBalancingScheme set to INTERNAL_SELF_MANAGED.
* Structure is documented below.
* @property pathTemplateMatch For satisfying the matchRule condition, the path of the request
* must match the wildcard pattern specified in pathTemplateMatch
* after removing any query parameters and anchor that may be part
* of the original URL.
* pathTemplateMatch must be between 1 and 255 characters
* (inclusive). The pattern specified by pathTemplateMatch may
* have at most 5 wildcard operators and at most 5 variable
* captures in total.
* @property prefixMatch For satisfying the matchRule condition, the request's path must begin with the
* specified prefixMatch. prefixMatch must begin with a /. The value must be
* between 1 and 1024 characters. Only one of prefixMatch, fullPathMatch or
* regexMatch must be specified.
* @property queryParameterMatches Specifies a list of query parameter match criteria, all of which must match
* corresponding query parameters in the request.
* Structure is documented below.
* @property regexMatch For satisfying the matchRule condition, the path of the request must satisfy the
* regular expression specified in regexMatch after removing any query parameters
* and anchor supplied with the original URL. For regular expression grammar please
* see en.cppreference.com/w/cpp/regex/ecmascript Only one of prefixMatch,
* fullPathMatch or regexMatch must be specified.
*/
public data class URLMapPathMatcherRouteRuleMatchRule(
public val fullPathMatch: String? = null,
public val headerMatches: List? = null,
public val ignoreCase: Boolean? = null,
public val metadataFilters: List? = null,
public val pathTemplateMatch: String? = null,
public val prefixMatch: String? = null,
public val queryParameterMatches: List? =
null,
public val regexMatch: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.URLMapPathMatcherRouteRuleMatchRule): URLMapPathMatcherRouteRuleMatchRule = URLMapPathMatcherRouteRuleMatchRule(
fullPathMatch = javaType.fullPathMatch().map({ args0 -> args0 }).orElse(null),
headerMatches = javaType.headerMatches().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.URLMapPathMatcherRouteRuleMatchRuleHeaderMatch.Companion.toKotlin(args0)
})
}),
ignoreCase = javaType.ignoreCase().map({ args0 -> args0 }).orElse(null),
metadataFilters = javaType.metadataFilters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.URLMapPathMatcherRouteRuleMatchRuleMetadataFilter.Companion.toKotlin(args0)
})
}),
pathTemplateMatch = javaType.pathTemplateMatch().map({ args0 -> args0 }).orElse(null),
prefixMatch = javaType.prefixMatch().map({ args0 -> args0 }).orElse(null),
queryParameterMatches = javaType.queryParameterMatches().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatch.Companion.toKotlin(args0)
})
}),
regexMatch = javaType.regexMatch().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy