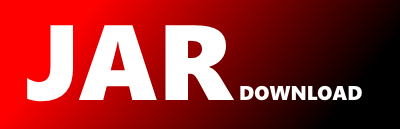
com.pulumi.gcp.container.kotlin.inputs.ClusterAddonsConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.container.inputs.ClusterAddonsConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property cloudrunConfig . Structure is documented below.
* @property configConnectorConfig .
* The status of the ConfigConnector addon. It is disabled by default; Set `enabled = true` to enable.
* @property dnsCacheConfig .
* The status of the NodeLocal DNSCache addon. It is disabled by default.
* Set `enabled = true` to enable.
* **Enabling/Disabling NodeLocal DNSCache in an existing cluster is a disruptive operation.
* All cluster nodes running GKE 1.15 and higher are recreated.**
* @property gcePersistentDiskCsiDriverConfig .
* Whether this cluster should enable the Google Compute Engine Persistent Disk Container Storage Interface (CSI) Driver. Set `enabled = true` to enable.
* **Note:** The Compute Engine persistent disk CSI Driver is enabled by default on newly created clusters for the following versions: Linux clusters: GKE version 1.18.10-gke.2100 or later, or 1.19.3-gke.2100 or later.
* @property gcpFilestoreCsiDriverConfig The status of the Filestore CSI driver addon,
* which allows the usage of filestore instance as volumes.
* It is disabled by default; set `enabled = true` to enable.
* @property gcsFuseCsiDriverConfig The status of the GCSFuse CSI driver addon,
* which allows the usage of a gcs bucket as volumes.
* It is disabled by default for Standard clusters; set `enabled = true` to enable.
* It is enabled by default for Autopilot clusters with version 1.24 or later; set `enabled = true` to enable it explicitly.
* See [Enable the Cloud Storage FUSE CSI driver](https://cloud.google.com/kubernetes-engine/docs/how-to/persistent-volumes/cloud-storage-fuse-csi-driver#enable) for more information.
* @property gkeBackupAgentConfig .
* The status of the Backup for GKE agent addon. It is disabled by default; Set `enabled = true` to enable.
* @property horizontalPodAutoscaling The status of the Horizontal Pod Autoscaling
* addon, which increases or decreases the number of replica pods a replication controller
* has based on the resource usage of the existing pods.
* It is enabled by default;
* set `disabled = true` to disable.
* @property httpLoadBalancing The status of the HTTP (L7) load balancing
* controller addon, which makes it easy to set up HTTP load balancers for services in a
* cluster. It is enabled by default; set `disabled = true` to disable.
* @property istioConfig .
* Structure is documented below.
* @property kalmConfig .
* Configuration for the KALM addon, which manages the lifecycle of k8s. It is disabled by default; Set `enabled = true` to enable.
* @property networkPolicyConfig Whether we should enable the network policy addon
* for the master. This must be enabled in order to enable network policy for the nodes.
* To enable this, you must also define a `network_policy` block,
* otherwise nothing will happen.
* It can only be disabled if the nodes already do not have network policies enabled.
* Defaults to disabled; set `disabled = false` to enable.
* @property statefulHaConfig .
* The status of the Stateful HA addon, which provides automatic configurable failover for stateful applications.
* It is disabled by default for Standard clusters. Set `enabled = true` to enable.
* This example `addons_config` disables two addons:
*/
public data class ClusterAddonsConfigArgs(
public val cloudrunConfig: Output? = null,
public val configConnectorConfig: Output? = null,
public val dnsCacheConfig: Output? = null,
public val gcePersistentDiskCsiDriverConfig: Output? = null,
public val gcpFilestoreCsiDriverConfig: Output? = null,
public val gcsFuseCsiDriverConfig: Output? = null,
public val gkeBackupAgentConfig: Output? = null,
public val horizontalPodAutoscaling: Output? =
null,
public val httpLoadBalancing: Output? = null,
public val istioConfig: Output? = null,
public val kalmConfig: Output? = null,
public val networkPolicyConfig: Output? = null,
public val statefulHaConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.container.inputs.ClusterAddonsConfigArgs =
com.pulumi.gcp.container.inputs.ClusterAddonsConfigArgs.builder()
.cloudrunConfig(cloudrunConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.configConnectorConfig(
configConnectorConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.dnsCacheConfig(dnsCacheConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.gcePersistentDiskCsiDriverConfig(
gcePersistentDiskCsiDriverConfig?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.gcpFilestoreCsiDriverConfig(
gcpFilestoreCsiDriverConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.gcsFuseCsiDriverConfig(
gcsFuseCsiDriverConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.gkeBackupAgentConfig(
gkeBackupAgentConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.horizontalPodAutoscaling(
horizontalPodAutoscaling?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.httpLoadBalancing(httpLoadBalancing?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.istioConfig(istioConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.kalmConfig(kalmConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.networkPolicyConfig(
networkPolicyConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.statefulHaConfig(
statefulHaConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ClusterAddonsConfigArgs].
*/
@PulumiTagMarker
public class ClusterAddonsConfigArgsBuilder internal constructor() {
private var cloudrunConfig: Output? = null
private var configConnectorConfig: Output? = null
private var dnsCacheConfig: Output? = null
private var gcePersistentDiskCsiDriverConfig:
Output? = null
private var gcpFilestoreCsiDriverConfig:
Output? = null
private var gcsFuseCsiDriverConfig: Output? = null
private var gkeBackupAgentConfig: Output? = null
private var horizontalPodAutoscaling: Output? =
null
private var httpLoadBalancing: Output? = null
private var istioConfig: Output? = null
private var kalmConfig: Output? = null
private var networkPolicyConfig: Output? = null
private var statefulHaConfig: Output? = null
/**
* @param value . Structure is documented below.
*/
@JvmName("bhasdumecnyjqcif")
public suspend fun cloudrunConfig(`value`: Output) {
this.cloudrunConfig = value
}
/**
* @param value .
* The status of the ConfigConnector addon. It is disabled by default; Set `enabled = true` to enable.
*/
@JvmName("avdndmmgpfkevnci")
public suspend fun configConnectorConfig(`value`: Output) {
this.configConnectorConfig = value
}
/**
* @param value .
* The status of the NodeLocal DNSCache addon. It is disabled by default.
* Set `enabled = true` to enable.
* **Enabling/Disabling NodeLocal DNSCache in an existing cluster is a disruptive operation.
* All cluster nodes running GKE 1.15 and higher are recreated.**
*/
@JvmName("rjngssepnqhxhuqb")
public suspend fun dnsCacheConfig(`value`: Output) {
this.dnsCacheConfig = value
}
/**
* @param value .
* Whether this cluster should enable the Google Compute Engine Persistent Disk Container Storage Interface (CSI) Driver. Set `enabled = true` to enable.
* **Note:** The Compute Engine persistent disk CSI Driver is enabled by default on newly created clusters for the following versions: Linux clusters: GKE version 1.18.10-gke.2100 or later, or 1.19.3-gke.2100 or later.
*/
@JvmName("uurxnkcnffijshds")
public suspend fun gcePersistentDiskCsiDriverConfig(`value`: Output) {
this.gcePersistentDiskCsiDriverConfig = value
}
/**
* @param value The status of the Filestore CSI driver addon,
* which allows the usage of filestore instance as volumes.
* It is disabled by default; set `enabled = true` to enable.
*/
@JvmName("dgpkrcaxnnvwikeg")
public suspend fun gcpFilestoreCsiDriverConfig(`value`: Output) {
this.gcpFilestoreCsiDriverConfig = value
}
/**
* @param value The status of the GCSFuse CSI driver addon,
* which allows the usage of a gcs bucket as volumes.
* It is disabled by default for Standard clusters; set `enabled = true` to enable.
* It is enabled by default for Autopilot clusters with version 1.24 or later; set `enabled = true` to enable it explicitly.
* See [Enable the Cloud Storage FUSE CSI driver](https://cloud.google.com/kubernetes-engine/docs/how-to/persistent-volumes/cloud-storage-fuse-csi-driver#enable) for more information.
*/
@JvmName("pruhbsoownpecrmr")
public suspend fun gcsFuseCsiDriverConfig(`value`: Output) {
this.gcsFuseCsiDriverConfig = value
}
/**
* @param value .
* The status of the Backup for GKE agent addon. It is disabled by default; Set `enabled = true` to enable.
*/
@JvmName("ehahwatkukqaerkf")
public suspend fun gkeBackupAgentConfig(`value`: Output) {
this.gkeBackupAgentConfig = value
}
/**
* @param value The status of the Horizontal Pod Autoscaling
* addon, which increases or decreases the number of replica pods a replication controller
* has based on the resource usage of the existing pods.
* It is enabled by default;
* set `disabled = true` to disable.
*/
@JvmName("wsxjjhxegojdwmyh")
public suspend fun horizontalPodAutoscaling(`value`: Output) {
this.horizontalPodAutoscaling = value
}
/**
* @param value The status of the HTTP (L7) load balancing
* controller addon, which makes it easy to set up HTTP load balancers for services in a
* cluster. It is enabled by default; set `disabled = true` to disable.
*/
@JvmName("xencsuhqgsywnqcb")
public suspend fun httpLoadBalancing(`value`: Output) {
this.httpLoadBalancing = value
}
/**
* @param value .
* Structure is documented below.
*/
@JvmName("dkqrqfrrsecknboe")
public suspend fun istioConfig(`value`: Output) {
this.istioConfig = value
}
/**
* @param value .
* Configuration for the KALM addon, which manages the lifecycle of k8s. It is disabled by default; Set `enabled = true` to enable.
*/
@JvmName("fiqjofspmsfqywvy")
public suspend fun kalmConfig(`value`: Output) {
this.kalmConfig = value
}
/**
* @param value Whether we should enable the network policy addon
* for the master. This must be enabled in order to enable network policy for the nodes.
* To enable this, you must also define a `network_policy` block,
* otherwise nothing will happen.
* It can only be disabled if the nodes already do not have network policies enabled.
* Defaults to disabled; set `disabled = false` to enable.
*/
@JvmName("vsmustkxrtimgojo")
public suspend fun networkPolicyConfig(`value`: Output) {
this.networkPolicyConfig = value
}
/**
* @param value .
* The status of the Stateful HA addon, which provides automatic configurable failover for stateful applications.
* It is disabled by default for Standard clusters. Set `enabled = true` to enable.
* This example `addons_config` disables two addons:
*/
@JvmName("dacqkqmhncwjeeop")
public suspend fun statefulHaConfig(`value`: Output) {
this.statefulHaConfig = value
}
/**
* @param value . Structure is documented below.
*/
@JvmName("fpnkpsfjltucxdby")
public suspend fun cloudrunConfig(`value`: ClusterAddonsConfigCloudrunConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudrunConfig = mapped
}
/**
* @param argument . Structure is documented below.
*/
@JvmName("etficwpfibtkhmne")
public suspend fun cloudrunConfig(argument: suspend ClusterAddonsConfigCloudrunConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigCloudrunConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cloudrunConfig = mapped
}
/**
* @param value .
* The status of the ConfigConnector addon. It is disabled by default; Set `enabled = true` to enable.
*/
@JvmName("ajvaoyoptoqttrwv")
public suspend fun configConnectorConfig(`value`: ClusterAddonsConfigConfigConnectorConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configConnectorConfig = mapped
}
/**
* @param argument .
* The status of the ConfigConnector addon. It is disabled by default; Set `enabled = true` to enable.
*/
@JvmName("vbadqqbgkshlnybs")
public suspend fun configConnectorConfig(argument: suspend ClusterAddonsConfigConfigConnectorConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigConfigConnectorConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.configConnectorConfig = mapped
}
/**
* @param value .
* The status of the NodeLocal DNSCache addon. It is disabled by default.
* Set `enabled = true` to enable.
* **Enabling/Disabling NodeLocal DNSCache in an existing cluster is a disruptive operation.
* All cluster nodes running GKE 1.15 and higher are recreated.**
*/
@JvmName("mtwtksltfndvfbsw")
public suspend fun dnsCacheConfig(`value`: ClusterAddonsConfigDnsCacheConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dnsCacheConfig = mapped
}
/**
* @param argument .
* The status of the NodeLocal DNSCache addon. It is disabled by default.
* Set `enabled = true` to enable.
* **Enabling/Disabling NodeLocal DNSCache in an existing cluster is a disruptive operation.
* All cluster nodes running GKE 1.15 and higher are recreated.**
*/
@JvmName("psajlagqbifmovmd")
public suspend fun dnsCacheConfig(argument: suspend ClusterAddonsConfigDnsCacheConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigDnsCacheConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dnsCacheConfig = mapped
}
/**
* @param value .
* Whether this cluster should enable the Google Compute Engine Persistent Disk Container Storage Interface (CSI) Driver. Set `enabled = true` to enable.
* **Note:** The Compute Engine persistent disk CSI Driver is enabled by default on newly created clusters for the following versions: Linux clusters: GKE version 1.18.10-gke.2100 or later, or 1.19.3-gke.2100 or later.
*/
@JvmName("qttddbxrtfmdoirp")
public suspend fun gcePersistentDiskCsiDriverConfig(`value`: ClusterAddonsConfigGcePersistentDiskCsiDriverConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcePersistentDiskCsiDriverConfig = mapped
}
/**
* @param argument .
* Whether this cluster should enable the Google Compute Engine Persistent Disk Container Storage Interface (CSI) Driver. Set `enabled = true` to enable.
* **Note:** The Compute Engine persistent disk CSI Driver is enabled by default on newly created clusters for the following versions: Linux clusters: GKE version 1.18.10-gke.2100 or later, or 1.19.3-gke.2100 or later.
*/
@JvmName("ujnemteuexgdubrm")
public suspend fun gcePersistentDiskCsiDriverConfig(argument: suspend ClusterAddonsConfigGcePersistentDiskCsiDriverConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigGcePersistentDiskCsiDriverConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.gcePersistentDiskCsiDriverConfig = mapped
}
/**
* @param value The status of the Filestore CSI driver addon,
* which allows the usage of filestore instance as volumes.
* It is disabled by default; set `enabled = true` to enable.
*/
@JvmName("tioorisgdlqrcarb")
public suspend fun gcpFilestoreCsiDriverConfig(`value`: ClusterAddonsConfigGcpFilestoreCsiDriverConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcpFilestoreCsiDriverConfig = mapped
}
/**
* @param argument The status of the Filestore CSI driver addon,
* which allows the usage of filestore instance as volumes.
* It is disabled by default; set `enabled = true` to enable.
*/
@JvmName("wrcmmsgmvgjrklfv")
public suspend fun gcpFilestoreCsiDriverConfig(argument: suspend ClusterAddonsConfigGcpFilestoreCsiDriverConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigGcpFilestoreCsiDriverConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.gcpFilestoreCsiDriverConfig = mapped
}
/**
* @param value The status of the GCSFuse CSI driver addon,
* which allows the usage of a gcs bucket as volumes.
* It is disabled by default for Standard clusters; set `enabled = true` to enable.
* It is enabled by default for Autopilot clusters with version 1.24 or later; set `enabled = true` to enable it explicitly.
* See [Enable the Cloud Storage FUSE CSI driver](https://cloud.google.com/kubernetes-engine/docs/how-to/persistent-volumes/cloud-storage-fuse-csi-driver#enable) for more information.
*/
@JvmName("hdyhlvtrtmbjybur")
public suspend fun gcsFuseCsiDriverConfig(`value`: ClusterAddonsConfigGcsFuseCsiDriverConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcsFuseCsiDriverConfig = mapped
}
/**
* @param argument The status of the GCSFuse CSI driver addon,
* which allows the usage of a gcs bucket as volumes.
* It is disabled by default for Standard clusters; set `enabled = true` to enable.
* It is enabled by default for Autopilot clusters with version 1.24 or later; set `enabled = true` to enable it explicitly.
* See [Enable the Cloud Storage FUSE CSI driver](https://cloud.google.com/kubernetes-engine/docs/how-to/persistent-volumes/cloud-storage-fuse-csi-driver#enable) for more information.
*/
@JvmName("otsgjprckrrioqdm")
public suspend fun gcsFuseCsiDriverConfig(argument: suspend ClusterAddonsConfigGcsFuseCsiDriverConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigGcsFuseCsiDriverConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.gcsFuseCsiDriverConfig = mapped
}
/**
* @param value .
* The status of the Backup for GKE agent addon. It is disabled by default; Set `enabled = true` to enable.
*/
@JvmName("fsbxrefubyacbkda")
public suspend fun gkeBackupAgentConfig(`value`: ClusterAddonsConfigGkeBackupAgentConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gkeBackupAgentConfig = mapped
}
/**
* @param argument .
* The status of the Backup for GKE agent addon. It is disabled by default; Set `enabled = true` to enable.
*/
@JvmName("vwnrdoavmfbhcddx")
public suspend fun gkeBackupAgentConfig(argument: suspend ClusterAddonsConfigGkeBackupAgentConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigGkeBackupAgentConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.gkeBackupAgentConfig = mapped
}
/**
* @param value The status of the Horizontal Pod Autoscaling
* addon, which increases or decreases the number of replica pods a replication controller
* has based on the resource usage of the existing pods.
* It is enabled by default;
* set `disabled = true` to disable.
*/
@JvmName("wtgbohoedbrpsaiw")
public suspend fun horizontalPodAutoscaling(`value`: ClusterAddonsConfigHorizontalPodAutoscalingArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.horizontalPodAutoscaling = mapped
}
/**
* @param argument The status of the Horizontal Pod Autoscaling
* addon, which increases or decreases the number of replica pods a replication controller
* has based on the resource usage of the existing pods.
* It is enabled by default;
* set `disabled = true` to disable.
*/
@JvmName("bdxdsccwcmxfvnvd")
public suspend fun horizontalPodAutoscaling(argument: suspend ClusterAddonsConfigHorizontalPodAutoscalingArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigHorizontalPodAutoscalingArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.horizontalPodAutoscaling = mapped
}
/**
* @param value The status of the HTTP (L7) load balancing
* controller addon, which makes it easy to set up HTTP load balancers for services in a
* cluster. It is enabled by default; set `disabled = true` to disable.
*/
@JvmName("rqqeafnkatctaqsq")
public suspend fun httpLoadBalancing(`value`: ClusterAddonsConfigHttpLoadBalancingArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpLoadBalancing = mapped
}
/**
* @param argument The status of the HTTP (L7) load balancing
* controller addon, which makes it easy to set up HTTP load balancers for services in a
* cluster. It is enabled by default; set `disabled = true` to disable.
*/
@JvmName("gapxnuuistwvdjbh")
public suspend fun httpLoadBalancing(argument: suspend ClusterAddonsConfigHttpLoadBalancingArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigHttpLoadBalancingArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.httpLoadBalancing = mapped
}
/**
* @param value .
* Structure is documented below.
*/
@JvmName("kvbjskywscxdykiq")
public suspend fun istioConfig(`value`: ClusterAddonsConfigIstioConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.istioConfig = mapped
}
/**
* @param argument .
* Structure is documented below.
*/
@JvmName("kfadheucgekmfjqe")
public suspend fun istioConfig(argument: suspend ClusterAddonsConfigIstioConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigIstioConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.istioConfig = mapped
}
/**
* @param value .
* Configuration for the KALM addon, which manages the lifecycle of k8s. It is disabled by default; Set `enabled = true` to enable.
*/
@JvmName("esbqlciewvvwgfyw")
public suspend fun kalmConfig(`value`: ClusterAddonsConfigKalmConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kalmConfig = mapped
}
/**
* @param argument .
* Configuration for the KALM addon, which manages the lifecycle of k8s. It is disabled by default; Set `enabled = true` to enable.
*/
@JvmName("rurpgglhagxxvgeu")
public suspend fun kalmConfig(argument: suspend ClusterAddonsConfigKalmConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigKalmConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.kalmConfig = mapped
}
/**
* @param value Whether we should enable the network policy addon
* for the master. This must be enabled in order to enable network policy for the nodes.
* To enable this, you must also define a `network_policy` block,
* otherwise nothing will happen.
* It can only be disabled if the nodes already do not have network policies enabled.
* Defaults to disabled; set `disabled = false` to enable.
*/
@JvmName("tcdiryftnkfbgaju")
public suspend fun networkPolicyConfig(`value`: ClusterAddonsConfigNetworkPolicyConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.networkPolicyConfig = mapped
}
/**
* @param argument Whether we should enable the network policy addon
* for the master. This must be enabled in order to enable network policy for the nodes.
* To enable this, you must also define a `network_policy` block,
* otherwise nothing will happen.
* It can only be disabled if the nodes already do not have network policies enabled.
* Defaults to disabled; set `disabled = false` to enable.
*/
@JvmName("lobdhtlsmbnedbkc")
public suspend fun networkPolicyConfig(argument: suspend ClusterAddonsConfigNetworkPolicyConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigNetworkPolicyConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.networkPolicyConfig = mapped
}
/**
* @param value .
* The status of the Stateful HA addon, which provides automatic configurable failover for stateful applications.
* It is disabled by default for Standard clusters. Set `enabled = true` to enable.
* This example `addons_config` disables two addons:
*/
@JvmName("vnpaqfcgfqorwkkd")
public suspend fun statefulHaConfig(`value`: ClusterAddonsConfigStatefulHaConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.statefulHaConfig = mapped
}
/**
* @param argument .
* The status of the Stateful HA addon, which provides automatic configurable failover for stateful applications.
* It is disabled by default for Standard clusters. Set `enabled = true` to enable.
* This example `addons_config` disables two addons:
*/
@JvmName("ifubmbwniakvnwct")
public suspend fun statefulHaConfig(argument: suspend ClusterAddonsConfigStatefulHaConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterAddonsConfigStatefulHaConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.statefulHaConfig = mapped
}
internal fun build(): ClusterAddonsConfigArgs = ClusterAddonsConfigArgs(
cloudrunConfig = cloudrunConfig,
configConnectorConfig = configConnectorConfig,
dnsCacheConfig = dnsCacheConfig,
gcePersistentDiskCsiDriverConfig = gcePersistentDiskCsiDriverConfig,
gcpFilestoreCsiDriverConfig = gcpFilestoreCsiDriverConfig,
gcsFuseCsiDriverConfig = gcsFuseCsiDriverConfig,
gkeBackupAgentConfig = gkeBackupAgentConfig,
horizontalPodAutoscaling = horizontalPodAutoscaling,
httpLoadBalancing = httpLoadBalancing,
istioConfig = istioConfig,
kalmConfig = kalmConfig,
networkPolicyConfig = networkPolicyConfig,
statefulHaConfig = statefulHaConfig,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy