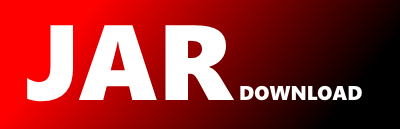
com.pulumi.gcp.container.kotlin.inputs.ClusterClusterAutoscalingAutoProvisioningDefaultsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.container.inputs.ClusterClusterAutoscalingAutoProvisioningDefaultsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property bootDiskKmsKey The Customer Managed Encryption Key used to encrypt the boot disk attached to each node in the node pool. This should be of the form projects/[KEY_PROJECT_ID]/locations/[LOCATION]/keyRings/[RING_NAME]/cryptoKeys/[KEY_NAME]. For more information about protecting resources with Cloud KMS Keys please see: https://cloud.google.com/compute/docs/disks/customer-managed-encryption
* @property diskSize Size of the disk attached to each node, specified in GB. The smallest allowed disk size is 10GB. Defaults to `100`
* @property diskType Type of the disk attached to each node (e.g. 'pd-standard', 'pd-ssd' or 'pd-balanced'). Defaults to `pd-standard`
* @property imageType The default image type used by NAP once a new node pool is being created. Please note that according to the [official documentation](https://cloud.google.com/kubernetes-engine/docs/how-to/node-auto-provisioning#default-image-type) the value must be one of the [COS_CONTAINERD, COS, UBUNTU_CONTAINERD, UBUNTU]. __NOTE__ : COS AND UBUNTU are deprecated as of `GKE 1.24`
* @property management NodeManagement configuration for this NodePool. Structure is documented below.
* @property minCpuPlatform Minimum CPU platform to be used for NAP created node pools. The instance may be scheduled on the
* specified or newer CPU platform. Applicable values are the friendly names of CPU platforms, such
* as "Intel Haswell" or "Intel Sandy Bridge".
* @property oauthScopes Scopes that are used by NAP and GKE Autopilot when creating node pools. Use the "https://www.googleapis.com/auth/cloud-platform" scope to grant access to all APIs. It is recommended that you set `service_account` to a non-default service account and grant IAM roles to that service account for only the resources that it needs.
* > `monitoring.write` is always enabled regardless of user input. `monitoring` and `logging.write` may also be enabled depending on the values for `monitoring_service` and `logging_service`.
* @property serviceAccount The Google Cloud Platform Service Account to be used by the node VMs created by GKE Autopilot or NAP.
* @property shieldedInstanceConfig Shielded Instance options. Structure is documented below.
* @property upgradeSettings Specifies the upgrade settings for NAP created node pools
*/
public data class ClusterClusterAutoscalingAutoProvisioningDefaultsArgs(
public val bootDiskKmsKey: Output? = null,
public val diskSize: Output? = null,
public val diskType: Output? = null,
public val imageType: Output? = null,
public val management: Output? =
null,
public val minCpuPlatform: Output? = null,
public val oauthScopes: Output>? = null,
public val serviceAccount: Output? = null,
public val shieldedInstanceConfig: Output? = null,
public val upgradeSettings: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.container.inputs.ClusterClusterAutoscalingAutoProvisioningDefaultsArgs =
com.pulumi.gcp.container.inputs.ClusterClusterAutoscalingAutoProvisioningDefaultsArgs.builder()
.bootDiskKmsKey(bootDiskKmsKey?.applyValue({ args0 -> args0 }))
.diskSize(diskSize?.applyValue({ args0 -> args0 }))
.diskType(diskType?.applyValue({ args0 -> args0 }))
.imageType(imageType?.applyValue({ args0 -> args0 }))
.management(management?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.minCpuPlatform(minCpuPlatform?.applyValue({ args0 -> args0 }))
.oauthScopes(oauthScopes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.serviceAccount(serviceAccount?.applyValue({ args0 -> args0 }))
.shieldedInstanceConfig(
shieldedInstanceConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.upgradeSettings(
upgradeSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ClusterClusterAutoscalingAutoProvisioningDefaultsArgs].
*/
@PulumiTagMarker
public class ClusterClusterAutoscalingAutoProvisioningDefaultsArgsBuilder internal constructor() {
private var bootDiskKmsKey: Output? = null
private var diskSize: Output? = null
private var diskType: Output? = null
private var imageType: Output? = null
private var management: Output? =
null
private var minCpuPlatform: Output? = null
private var oauthScopes: Output>? = null
private var serviceAccount: Output? = null
private var shieldedInstanceConfig:
Output? = null
private var upgradeSettings:
Output? = null
/**
* @param value The Customer Managed Encryption Key used to encrypt the boot disk attached to each node in the node pool. This should be of the form projects/[KEY_PROJECT_ID]/locations/[LOCATION]/keyRings/[RING_NAME]/cryptoKeys/[KEY_NAME]. For more information about protecting resources with Cloud KMS Keys please see: https://cloud.google.com/compute/docs/disks/customer-managed-encryption
*/
@JvmName("foubtqjnpoxanrhx")
public suspend fun bootDiskKmsKey(`value`: Output) {
this.bootDiskKmsKey = value
}
/**
* @param value Size of the disk attached to each node, specified in GB. The smallest allowed disk size is 10GB. Defaults to `100`
*/
@JvmName("lxaruocjjdscjruv")
public suspend fun diskSize(`value`: Output) {
this.diskSize = value
}
/**
* @param value Type of the disk attached to each node (e.g. 'pd-standard', 'pd-ssd' or 'pd-balanced'). Defaults to `pd-standard`
*/
@JvmName("mdoacjseikkpitvp")
public suspend fun diskType(`value`: Output) {
this.diskType = value
}
/**
* @param value The default image type used by NAP once a new node pool is being created. Please note that according to the [official documentation](https://cloud.google.com/kubernetes-engine/docs/how-to/node-auto-provisioning#default-image-type) the value must be one of the [COS_CONTAINERD, COS, UBUNTU_CONTAINERD, UBUNTU]. __NOTE__ : COS AND UBUNTU are deprecated as of `GKE 1.24`
*/
@JvmName("yyfsuymomlbhetri")
public suspend fun imageType(`value`: Output) {
this.imageType = value
}
/**
* @param value NodeManagement configuration for this NodePool. Structure is documented below.
*/
@JvmName("whwltdtmivdqbmfq")
public suspend fun management(`value`: Output) {
this.management = value
}
/**
* @param value Minimum CPU platform to be used for NAP created node pools. The instance may be scheduled on the
* specified or newer CPU platform. Applicable values are the friendly names of CPU platforms, such
* as "Intel Haswell" or "Intel Sandy Bridge".
*/
@JvmName("jurgdokxvbaxnuwx")
public suspend fun minCpuPlatform(`value`: Output) {
this.minCpuPlatform = value
}
/**
* @param value Scopes that are used by NAP and GKE Autopilot when creating node pools. Use the "https://www.googleapis.com/auth/cloud-platform" scope to grant access to all APIs. It is recommended that you set `service_account` to a non-default service account and grant IAM roles to that service account for only the resources that it needs.
* > `monitoring.write` is always enabled regardless of user input. `monitoring` and `logging.write` may also be enabled depending on the values for `monitoring_service` and `logging_service`.
*/
@JvmName("eelmfngfbxqjcdqa")
public suspend fun oauthScopes(`value`: Output>) {
this.oauthScopes = value
}
@JvmName("hfwwrahqenvmrtlx")
public suspend fun oauthScopes(vararg values: Output) {
this.oauthScopes = Output.all(values.asList())
}
/**
* @param values Scopes that are used by NAP and GKE Autopilot when creating node pools. Use the "https://www.googleapis.com/auth/cloud-platform" scope to grant access to all APIs. It is recommended that you set `service_account` to a non-default service account and grant IAM roles to that service account for only the resources that it needs.
* > `monitoring.write` is always enabled regardless of user input. `monitoring` and `logging.write` may also be enabled depending on the values for `monitoring_service` and `logging_service`.
*/
@JvmName("qjiuxrpwwxkgnvmt")
public suspend fun oauthScopes(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy