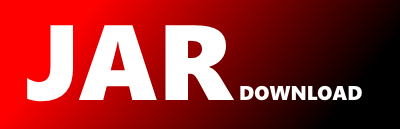
com.pulumi.gcp.container.kotlin.inputs.ClusterDnsConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.container.inputs.ClusterDnsConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property additiveVpcScopeDnsDomain This will enable Cloud DNS additive VPC scope. Must provide a domain name that is unique within the VPC. For this to work `cluster_dns = "CLOUD_DNS"` and `cluster_dns_scope = "CLUSTER_SCOPE"` must both be set as well.
* @property clusterDns Which in-cluster DNS provider should be used. `PROVIDER_UNSPECIFIED` (default) or `PLATFORM_DEFAULT` or `CLOUD_DNS`.
* @property clusterDnsDomain The suffix used for all cluster service records.
* @property clusterDnsScope The scope of access to cluster DNS records. `DNS_SCOPE_UNSPECIFIED` (default) or `CLUSTER_SCOPE` or `VPC_SCOPE`.
*/
public data class ClusterDnsConfigArgs(
public val additiveVpcScopeDnsDomain: Output? = null,
public val clusterDns: Output? = null,
public val clusterDnsDomain: Output? = null,
public val clusterDnsScope: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.container.inputs.ClusterDnsConfigArgs =
com.pulumi.gcp.container.inputs.ClusterDnsConfigArgs.builder()
.additiveVpcScopeDnsDomain(additiveVpcScopeDnsDomain?.applyValue({ args0 -> args0 }))
.clusterDns(clusterDns?.applyValue({ args0 -> args0 }))
.clusterDnsDomain(clusterDnsDomain?.applyValue({ args0 -> args0 }))
.clusterDnsScope(clusterDnsScope?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterDnsConfigArgs].
*/
@PulumiTagMarker
public class ClusterDnsConfigArgsBuilder internal constructor() {
private var additiveVpcScopeDnsDomain: Output? = null
private var clusterDns: Output? = null
private var clusterDnsDomain: Output? = null
private var clusterDnsScope: Output? = null
/**
* @param value This will enable Cloud DNS additive VPC scope. Must provide a domain name that is unique within the VPC. For this to work `cluster_dns = "CLOUD_DNS"` and `cluster_dns_scope = "CLUSTER_SCOPE"` must both be set as well.
*/
@JvmName("oaqdciothtveurnx")
public suspend fun additiveVpcScopeDnsDomain(`value`: Output) {
this.additiveVpcScopeDnsDomain = value
}
/**
* @param value Which in-cluster DNS provider should be used. `PROVIDER_UNSPECIFIED` (default) or `PLATFORM_DEFAULT` or `CLOUD_DNS`.
*/
@JvmName("thvmxjfukitkhnjd")
public suspend fun clusterDns(`value`: Output) {
this.clusterDns = value
}
/**
* @param value The suffix used for all cluster service records.
*/
@JvmName("ldugoojsybcgnwuj")
public suspend fun clusterDnsDomain(`value`: Output) {
this.clusterDnsDomain = value
}
/**
* @param value The scope of access to cluster DNS records. `DNS_SCOPE_UNSPECIFIED` (default) or `CLUSTER_SCOPE` or `VPC_SCOPE`.
*/
@JvmName("ywwjlqjthcpjkssa")
public suspend fun clusterDnsScope(`value`: Output) {
this.clusterDnsScope = value
}
/**
* @param value This will enable Cloud DNS additive VPC scope. Must provide a domain name that is unique within the VPC. For this to work `cluster_dns = "CLOUD_DNS"` and `cluster_dns_scope = "CLUSTER_SCOPE"` must both be set as well.
*/
@JvmName("ndrjsmdbhwsjcjri")
public suspend fun additiveVpcScopeDnsDomain(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.additiveVpcScopeDnsDomain = mapped
}
/**
* @param value Which in-cluster DNS provider should be used. `PROVIDER_UNSPECIFIED` (default) or `PLATFORM_DEFAULT` or `CLOUD_DNS`.
*/
@JvmName("vijqknyyiehjyshm")
public suspend fun clusterDns(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterDns = mapped
}
/**
* @param value The suffix used for all cluster service records.
*/
@JvmName("mnfrqxbtpytmxvur")
public suspend fun clusterDnsDomain(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterDnsDomain = mapped
}
/**
* @param value The scope of access to cluster DNS records. `DNS_SCOPE_UNSPECIFIED` (default) or `CLUSTER_SCOPE` or `VPC_SCOPE`.
*/
@JvmName("daenilvoqprqfdgu")
public suspend fun clusterDnsScope(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterDnsScope = mapped
}
internal fun build(): ClusterDnsConfigArgs = ClusterDnsConfigArgs(
additiveVpcScopeDnsDomain = additiveVpcScopeDnsDomain,
clusterDns = clusterDns,
clusterDnsDomain = clusterDnsDomain,
clusterDnsScope = clusterDnsScope,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy