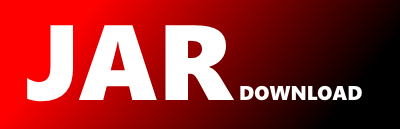
com.pulumi.gcp.container.kotlin.inputs.ClusterIpAllocationPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.container.inputs.ClusterIpAllocationPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property additionalPodRangesConfig The configuration for additional pod secondary ranges at
* the cluster level. Used for Autopilot clusters and Standard clusters with which control of the
* secondary Pod IP address assignment to node pools isn't needed. Structure is documented below.
* @property clusterIpv4CidrBlock The IP address range for the cluster pod IPs.
* Set to blank to have a range chosen with the default size. Set to /netmask (e.g. /14)
* to have a range chosen with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14)
* from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to
* pick a specific range to use.
* @property clusterSecondaryRangeName The name of the existing secondary
* range in the cluster's subnetwork to use for pod IP addresses. Alternatively,
* `cluster_ipv4_cidr_block` can be used to automatically create a GKE-managed one.
* @property podCidrOverprovisionConfig Configuration for cluster level pod cidr overprovision. Default is disabled=false.
* @property servicesIpv4CidrBlock The IP address range of the services IPs in this cluster.
* Set to blank to have a range chosen with the default size. Set to /netmask (e.g. /14)
* to have a range chosen with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14)
* from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to
* pick a specific range to use.
* @property servicesSecondaryRangeName The name of the existing
* secondary range in the cluster's subnetwork to use for service `ClusterIP`s.
* Alternatively, `services_ipv4_cidr_block` can be used to automatically create a
* GKE-managed one.
* @property stackType The IP Stack Type of the cluster.
* Default value is `IPV4`.
* Possible values are `IPV4` and `IPV4_IPV6`.
*/
public data class ClusterIpAllocationPolicyArgs(
public val additionalPodRangesConfig: Output? = null,
public val clusterIpv4CidrBlock: Output? = null,
public val clusterSecondaryRangeName: Output? = null,
public val podCidrOverprovisionConfig: Output? = null,
public val servicesIpv4CidrBlock: Output? = null,
public val servicesSecondaryRangeName: Output? = null,
public val stackType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.container.inputs.ClusterIpAllocationPolicyArgs =
com.pulumi.gcp.container.inputs.ClusterIpAllocationPolicyArgs.builder()
.additionalPodRangesConfig(
additionalPodRangesConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.clusterIpv4CidrBlock(clusterIpv4CidrBlock?.applyValue({ args0 -> args0 }))
.clusterSecondaryRangeName(clusterSecondaryRangeName?.applyValue({ args0 -> args0 }))
.podCidrOverprovisionConfig(
podCidrOverprovisionConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.servicesIpv4CidrBlock(servicesIpv4CidrBlock?.applyValue({ args0 -> args0 }))
.servicesSecondaryRangeName(servicesSecondaryRangeName?.applyValue({ args0 -> args0 }))
.stackType(stackType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterIpAllocationPolicyArgs].
*/
@PulumiTagMarker
public class ClusterIpAllocationPolicyArgsBuilder internal constructor() {
private var additionalPodRangesConfig:
Output? = null
private var clusterIpv4CidrBlock: Output? = null
private var clusterSecondaryRangeName: Output? = null
private var podCidrOverprovisionConfig:
Output? = null
private var servicesIpv4CidrBlock: Output? = null
private var servicesSecondaryRangeName: Output? = null
private var stackType: Output? = null
/**
* @param value The configuration for additional pod secondary ranges at
* the cluster level. Used for Autopilot clusters and Standard clusters with which control of the
* secondary Pod IP address assignment to node pools isn't needed. Structure is documented below.
*/
@JvmName("xwlgkpqsvsuaeswv")
public suspend fun additionalPodRangesConfig(`value`: Output) {
this.additionalPodRangesConfig = value
}
/**
* @param value The IP address range for the cluster pod IPs.
* Set to blank to have a range chosen with the default size. Set to /netmask (e.g. /14)
* to have a range chosen with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14)
* from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to
* pick a specific range to use.
*/
@JvmName("gnolkjtodjtyqcjf")
public suspend fun clusterIpv4CidrBlock(`value`: Output) {
this.clusterIpv4CidrBlock = value
}
/**
* @param value The name of the existing secondary
* range in the cluster's subnetwork to use for pod IP addresses. Alternatively,
* `cluster_ipv4_cidr_block` can be used to automatically create a GKE-managed one.
*/
@JvmName("xcmflitlkqxcpden")
public suspend fun clusterSecondaryRangeName(`value`: Output) {
this.clusterSecondaryRangeName = value
}
/**
* @param value Configuration for cluster level pod cidr overprovision. Default is disabled=false.
*/
@JvmName("vdtxidpicnhrrgbp")
public suspend fun podCidrOverprovisionConfig(`value`: Output) {
this.podCidrOverprovisionConfig = value
}
/**
* @param value The IP address range of the services IPs in this cluster.
* Set to blank to have a range chosen with the default size. Set to /netmask (e.g. /14)
* to have a range chosen with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14)
* from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to
* pick a specific range to use.
*/
@JvmName("omolhslvjucafrfp")
public suspend fun servicesIpv4CidrBlock(`value`: Output) {
this.servicesIpv4CidrBlock = value
}
/**
* @param value The name of the existing
* secondary range in the cluster's subnetwork to use for service `ClusterIP`s.
* Alternatively, `services_ipv4_cidr_block` can be used to automatically create a
* GKE-managed one.
*/
@JvmName("jkrrvtlcudctfgxr")
public suspend fun servicesSecondaryRangeName(`value`: Output) {
this.servicesSecondaryRangeName = value
}
/**
* @param value The IP Stack Type of the cluster.
* Default value is `IPV4`.
* Possible values are `IPV4` and `IPV4_IPV6`.
*/
@JvmName("rgdhywwnyxugjqsm")
public suspend fun stackType(`value`: Output) {
this.stackType = value
}
/**
* @param value The configuration for additional pod secondary ranges at
* the cluster level. Used for Autopilot clusters and Standard clusters with which control of the
* secondary Pod IP address assignment to node pools isn't needed. Structure is documented below.
*/
@JvmName("nwugsbhahuskoqrw")
public suspend fun additionalPodRangesConfig(`value`: ClusterIpAllocationPolicyAdditionalPodRangesConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.additionalPodRangesConfig = mapped
}
/**
* @param argument The configuration for additional pod secondary ranges at
* the cluster level. Used for Autopilot clusters and Standard clusters with which control of the
* secondary Pod IP address assignment to node pools isn't needed. Structure is documented below.
*/
@JvmName("vkuxuhkphegskffl")
public suspend fun additionalPodRangesConfig(argument: suspend ClusterIpAllocationPolicyAdditionalPodRangesConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterIpAllocationPolicyAdditionalPodRangesConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.additionalPodRangesConfig = mapped
}
/**
* @param value The IP address range for the cluster pod IPs.
* Set to blank to have a range chosen with the default size. Set to /netmask (e.g. /14)
* to have a range chosen with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14)
* from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to
* pick a specific range to use.
*/
@JvmName("yikdacajrbfuwngg")
public suspend fun clusterIpv4CidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterIpv4CidrBlock = mapped
}
/**
* @param value The name of the existing secondary
* range in the cluster's subnetwork to use for pod IP addresses. Alternatively,
* `cluster_ipv4_cidr_block` can be used to automatically create a GKE-managed one.
*/
@JvmName("opstppmrwyrldwmt")
public suspend fun clusterSecondaryRangeName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterSecondaryRangeName = mapped
}
/**
* @param value Configuration for cluster level pod cidr overprovision. Default is disabled=false.
*/
@JvmName("oojjqjfbqkhalukd")
public suspend fun podCidrOverprovisionConfig(`value`: ClusterIpAllocationPolicyPodCidrOverprovisionConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.podCidrOverprovisionConfig = mapped
}
/**
* @param argument Configuration for cluster level pod cidr overprovision. Default is disabled=false.
*/
@JvmName("fpgukujgcsgifnxw")
public suspend fun podCidrOverprovisionConfig(argument: suspend ClusterIpAllocationPolicyPodCidrOverprovisionConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterIpAllocationPolicyPodCidrOverprovisionConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.podCidrOverprovisionConfig = mapped
}
/**
* @param value The IP address range of the services IPs in this cluster.
* Set to blank to have a range chosen with the default size. Set to /netmask (e.g. /14)
* to have a range chosen with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14)
* from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to
* pick a specific range to use.
*/
@JvmName("cpaklnmpmtlsvpoi")
public suspend fun servicesIpv4CidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.servicesIpv4CidrBlock = mapped
}
/**
* @param value The name of the existing
* secondary range in the cluster's subnetwork to use for service `ClusterIP`s.
* Alternatively, `services_ipv4_cidr_block` can be used to automatically create a
* GKE-managed one.
*/
@JvmName("frhpdnqdojtnmeqg")
public suspend fun servicesSecondaryRangeName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.servicesSecondaryRangeName = mapped
}
/**
* @param value The IP Stack Type of the cluster.
* Default value is `IPV4`.
* Possible values are `IPV4` and `IPV4_IPV6`.
*/
@JvmName("qenauunvefmvpfpd")
public suspend fun stackType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stackType = mapped
}
internal fun build(): ClusterIpAllocationPolicyArgs = ClusterIpAllocationPolicyArgs(
additionalPodRangesConfig = additionalPodRangesConfig,
clusterIpv4CidrBlock = clusterIpv4CidrBlock,
clusterSecondaryRangeName = clusterSecondaryRangeName,
podCidrOverprovisionConfig = podCidrOverprovisionConfig,
servicesIpv4CidrBlock = servicesIpv4CidrBlock,
servicesSecondaryRangeName = servicesSecondaryRangeName,
stackType = stackType,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy