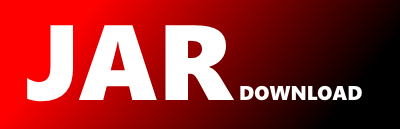
com.pulumi.gcp.container.kotlin.inputs.ClusterMaintenancePolicyMaintenanceExclusionExclusionOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.container.inputs.ClusterMaintenancePolicyMaintenanceExclusionExclusionOptionsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property scope The scope of automatic upgrades to restrict in the exclusion window. One of: **NO_UPGRADES | NO_MINOR_UPGRADES | NO_MINOR_OR_NODE_UPGRADES**
* Specify `start_time` and `end_time` in [RFC3339](https://www.ietf.org/rfc/rfc3339.txt) "Zulu" date format. The start time's date is
* the initial date that the window starts, and the end time is used for calculating duration.Specify `recurrence` in
* [RFC5545](https://tools.ietf.org/html/rfc5545#section-3.8.5.3) RRULE format, to specify when this recurs.
* Note that GKE may accept other formats, but will return values in UTC, causing a permanent diff.
* Examples:
* ```
* maintenance_policy {
* recurring_window {
* start_time = "2019-01-01T00:00:00Z"
* end_time = "2019-01-02T00:00:00Z"
* recurrence = "FREQ=DAILY"
* }
* maintenance_exclusion{
* exclusion_name = "batch job"
* start_time = "2019-01-01T00:00:00Z"
* end_time = "2019-01-02T00:00:00Z"
* exclusion_options {
* scope = "NO_UPGRADES"
* }
* }
* maintenance_exclusion{
* exclusion_name = "holiday data load"
* start_time = "2019-05-01T00:00:00Z"
* end_time = "2019-05-02T00:00:00Z"
* exclusion_options {
* scope = "NO_MINOR_UPGRADES"
* }
* }
* }
* ```
*/
public data class ClusterMaintenancePolicyMaintenanceExclusionExclusionOptionsArgs(
public val scope: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.container.inputs.ClusterMaintenancePolicyMaintenanceExclusionExclusionOptionsArgs =
com.pulumi.gcp.container.inputs.ClusterMaintenancePolicyMaintenanceExclusionExclusionOptionsArgs.builder()
.scope(scope.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterMaintenancePolicyMaintenanceExclusionExclusionOptionsArgs].
*/
@PulumiTagMarker
public class ClusterMaintenancePolicyMaintenanceExclusionExclusionOptionsArgsBuilder internal constructor() {
private var scope: Output? = null
/**
* @param value The scope of automatic upgrades to restrict in the exclusion window. One of: **NO_UPGRADES | NO_MINOR_UPGRADES | NO_MINOR_OR_NODE_UPGRADES**
* Specify `start_time` and `end_time` in [RFC3339](https://www.ietf.org/rfc/rfc3339.txt) "Zulu" date format. The start time's date is
* the initial date that the window starts, and the end time is used for calculating duration.Specify `recurrence` in
* [RFC5545](https://tools.ietf.org/html/rfc5545#section-3.8.5.3) RRULE format, to specify when this recurs.
* Note that GKE may accept other formats, but will return values in UTC, causing a permanent diff.
* Examples:
* ```
* maintenance_policy {
* recurring_window {
* start_time = "2019-01-01T00:00:00Z"
* end_time = "2019-01-02T00:00:00Z"
* recurrence = "FREQ=DAILY"
* }
* maintenance_exclusion{
* exclusion_name = "batch job"
* start_time = "2019-01-01T00:00:00Z"
* end_time = "2019-01-02T00:00:00Z"
* exclusion_options {
* scope = "NO_UPGRADES"
* }
* }
* maintenance_exclusion{
* exclusion_name = "holiday data load"
* start_time = "2019-05-01T00:00:00Z"
* end_time = "2019-05-02T00:00:00Z"
* exclusion_options {
* scope = "NO_MINOR_UPGRADES"
* }
* }
* }
* ```
*/
@JvmName("subbpcafxxvrghxk")
public suspend fun scope(`value`: Output) {
this.scope = value
}
/**
* @param value The scope of automatic upgrades to restrict in the exclusion window. One of: **NO_UPGRADES | NO_MINOR_UPGRADES | NO_MINOR_OR_NODE_UPGRADES**
* Specify `start_time` and `end_time` in [RFC3339](https://www.ietf.org/rfc/rfc3339.txt) "Zulu" date format. The start time's date is
* the initial date that the window starts, and the end time is used for calculating duration.Specify `recurrence` in
* [RFC5545](https://tools.ietf.org/html/rfc5545#section-3.8.5.3) RRULE format, to specify when this recurs.
* Note that GKE may accept other formats, but will return values in UTC, causing a permanent diff.
* Examples:
* ```
* maintenance_policy {
* recurring_window {
* start_time = "2019-01-01T00:00:00Z"
* end_time = "2019-01-02T00:00:00Z"
* recurrence = "FREQ=DAILY"
* }
* maintenance_exclusion{
* exclusion_name = "batch job"
* start_time = "2019-01-01T00:00:00Z"
* end_time = "2019-01-02T00:00:00Z"
* exclusion_options {
* scope = "NO_UPGRADES"
* }
* }
* maintenance_exclusion{
* exclusion_name = "holiday data load"
* start_time = "2019-05-01T00:00:00Z"
* end_time = "2019-05-02T00:00:00Z"
* exclusion_options {
* scope = "NO_MINOR_UPGRADES"
* }
* }
* }
* ```
*/
@JvmName("jbkcygjmjaomnhfr")
public suspend fun scope(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.scope = mapped
}
internal fun build(): ClusterMaintenancePolicyMaintenanceExclusionExclusionOptionsArgs =
ClusterMaintenancePolicyMaintenanceExclusionExclusionOptionsArgs(
scope = scope ?: throw PulumiNullFieldException("scope"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy