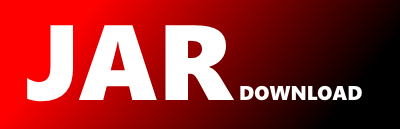
com.pulumi.gcp.container.kotlin.inputs.ClusterNodePoolNetworkConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.container.inputs.ClusterNodePoolNetworkConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property additionalNodeNetworkConfigs We specify the additional node networks for this node pool using this list. Each node network corresponds to an additional interface
* @property additionalPodNetworkConfigs We specify the additional pod networks for this node pool using this list. Each pod network corresponds to an additional alias IP range for the node
* @property createPodRange Whether to create a new range for pod IPs in this node pool. Defaults are provided for `pod_range` and `pod_ipv4_cidr_block` if they are not specified.
* @property enablePrivateNodes Whether nodes have internal IP addresses only.
* @property networkPerformanceConfig Network bandwidth tier configuration.
* @property podCidrOverprovisionConfig Configuration for node-pool level pod cidr overprovision. If not set, the cluster level setting will be inherited
* @property podIpv4CidrBlock The IP address range for pod IPs in this node pool. Only applicable if createPodRange is true. Set to blank to have a range chosen with the default size. Set to /netmask (e.g. /14) to have a range chosen with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14) to pick a specific range to use.
* @property podRange The ID of the secondary range for pod IPs. If `create_pod_range` is true, this ID is used for the new range. If `create_pod_range` is false, uses an existing secondary range with this ID.
*/
public data class ClusterNodePoolNetworkConfigArgs(
public val additionalNodeNetworkConfigs: Output>? = null,
public val additionalPodNetworkConfigs: Output>? = null,
public val createPodRange: Output? = null,
public val enablePrivateNodes: Output? = null,
public val networkPerformanceConfig: Output? = null,
public val podCidrOverprovisionConfig: Output? = null,
public val podIpv4CidrBlock: Output? = null,
public val podRange: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.container.inputs.ClusterNodePoolNetworkConfigArgs =
com.pulumi.gcp.container.inputs.ClusterNodePoolNetworkConfigArgs.builder()
.additionalNodeNetworkConfigs(
additionalNodeNetworkConfigs?.applyValue({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.additionalPodNetworkConfigs(
additionalPodNetworkConfigs?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.createPodRange(createPodRange?.applyValue({ args0 -> args0 }))
.enablePrivateNodes(enablePrivateNodes?.applyValue({ args0 -> args0 }))
.networkPerformanceConfig(
networkPerformanceConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.podCidrOverprovisionConfig(
podCidrOverprovisionConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.podIpv4CidrBlock(podIpv4CidrBlock?.applyValue({ args0 -> args0 }))
.podRange(podRange?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterNodePoolNetworkConfigArgs].
*/
@PulumiTagMarker
public class ClusterNodePoolNetworkConfigArgsBuilder internal constructor() {
private var additionalNodeNetworkConfigs:
Output>? = null
private var additionalPodNetworkConfigs:
Output>? = null
private var createPodRange: Output? = null
private var enablePrivateNodes: Output? = null
private var networkPerformanceConfig:
Output? = null
private var podCidrOverprovisionConfig:
Output? = null
private var podIpv4CidrBlock: Output? = null
private var podRange: Output? = null
/**
* @param value We specify the additional node networks for this node pool using this list. Each node network corresponds to an additional interface
*/
@JvmName("odjufwyfyihmvisw")
public suspend fun additionalNodeNetworkConfigs(`value`: Output>) {
this.additionalNodeNetworkConfigs = value
}
@JvmName("knxmjicqjgvxxiya")
public suspend fun additionalNodeNetworkConfigs(vararg values: Output) {
this.additionalNodeNetworkConfigs = Output.all(values.asList())
}
/**
* @param values We specify the additional node networks for this node pool using this list. Each node network corresponds to an additional interface
*/
@JvmName("wsipdjksafjxubts")
public suspend fun additionalNodeNetworkConfigs(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy