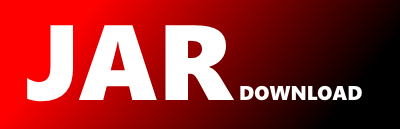
com.pulumi.gcp.container.kotlin.inputs.ClusterPrivateClusterConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.container.inputs.ClusterPrivateClusterConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property enablePrivateEndpoint When `true`, the cluster's private
* endpoint is used as the cluster endpoint and access through the public endpoint
* is disabled. When `false`, either endpoint can be used. This field only applies
* to private clusters, when `enable_private_nodes` is `true`.
* @property enablePrivateNodes Enables the private cluster feature,
* creating a private endpoint on the cluster. In a private cluster, nodes only
* have RFC 1918 private addresses and communicate with the master's private
* endpoint via private networking.
* @property masterGlobalAccessConfig Controls cluster master global
* access settings. If unset, the provider will no longer manage this field and will
* not modify the previously-set value. Structure is documented below.
* @property masterIpv4CidrBlock The IP range in CIDR notation to use for
* the hosted master network. This range will be used for assigning private IP
* addresses to the cluster master(s) and the ILB VIP. This range must not overlap
* with any other ranges in use within the cluster's network, and it must be a /28
* subnet. See [Private Cluster Limitations](https://cloud.google.com/kubernetes-engine/docs/how-to/private-clusters#req_res_lim)
* for more details. This field only applies to private clusters, when
* `enable_private_nodes` is `true`.
* @property peeringName The name of the peering between this cluster and the Google owned VPC.
* @property privateEndpoint The internal IP address of this cluster's master endpoint.
* @property privateEndpointSubnetwork Subnetwork in cluster's network where master's endpoint will be provisioned.
* @property publicEndpoint The external IP address of this cluster's master endpoint.
* !> The Google provider is unable to validate certain configurations of
* `private_cluster_config` when `enable_private_nodes` is `false`. It's
* recommended that you omit the block entirely if the field is not set to `true`.
*/
public data class ClusterPrivateClusterConfigArgs(
public val enablePrivateEndpoint: Output? = null,
public val enablePrivateNodes: Output? = null,
public val masterGlobalAccessConfig: Output? = null,
public val masterIpv4CidrBlock: Output? = null,
public val peeringName: Output? = null,
public val privateEndpoint: Output? = null,
public val privateEndpointSubnetwork: Output? = null,
public val publicEndpoint: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.container.inputs.ClusterPrivateClusterConfigArgs =
com.pulumi.gcp.container.inputs.ClusterPrivateClusterConfigArgs.builder()
.enablePrivateEndpoint(enablePrivateEndpoint?.applyValue({ args0 -> args0 }))
.enablePrivateNodes(enablePrivateNodes?.applyValue({ args0 -> args0 }))
.masterGlobalAccessConfig(
masterGlobalAccessConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.masterIpv4CidrBlock(masterIpv4CidrBlock?.applyValue({ args0 -> args0 }))
.peeringName(peeringName?.applyValue({ args0 -> args0 }))
.privateEndpoint(privateEndpoint?.applyValue({ args0 -> args0 }))
.privateEndpointSubnetwork(privateEndpointSubnetwork?.applyValue({ args0 -> args0 }))
.publicEndpoint(publicEndpoint?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterPrivateClusterConfigArgs].
*/
@PulumiTagMarker
public class ClusterPrivateClusterConfigArgsBuilder internal constructor() {
private var enablePrivateEndpoint: Output? = null
private var enablePrivateNodes: Output? = null
private var masterGlobalAccessConfig:
Output? = null
private var masterIpv4CidrBlock: Output? = null
private var peeringName: Output? = null
private var privateEndpoint: Output? = null
private var privateEndpointSubnetwork: Output? = null
private var publicEndpoint: Output? = null
/**
* @param value When `true`, the cluster's private
* endpoint is used as the cluster endpoint and access through the public endpoint
* is disabled. When `false`, either endpoint can be used. This field only applies
* to private clusters, when `enable_private_nodes` is `true`.
*/
@JvmName("msnwmfmkvwhorgtm")
public suspend fun enablePrivateEndpoint(`value`: Output) {
this.enablePrivateEndpoint = value
}
/**
* @param value Enables the private cluster feature,
* creating a private endpoint on the cluster. In a private cluster, nodes only
* have RFC 1918 private addresses and communicate with the master's private
* endpoint via private networking.
*/
@JvmName("tkfkslscdbdntcqv")
public suspend fun enablePrivateNodes(`value`: Output) {
this.enablePrivateNodes = value
}
/**
* @param value Controls cluster master global
* access settings. If unset, the provider will no longer manage this field and will
* not modify the previously-set value. Structure is documented below.
*/
@JvmName("klfbnbtxwnpnedmc")
public suspend fun masterGlobalAccessConfig(`value`: Output) {
this.masterGlobalAccessConfig = value
}
/**
* @param value The IP range in CIDR notation to use for
* the hosted master network. This range will be used for assigning private IP
* addresses to the cluster master(s) and the ILB VIP. This range must not overlap
* with any other ranges in use within the cluster's network, and it must be a /28
* subnet. See [Private Cluster Limitations](https://cloud.google.com/kubernetes-engine/docs/how-to/private-clusters#req_res_lim)
* for more details. This field only applies to private clusters, when
* `enable_private_nodes` is `true`.
*/
@JvmName("ckuxgkodfpmyeejg")
public suspend fun masterIpv4CidrBlock(`value`: Output) {
this.masterIpv4CidrBlock = value
}
/**
* @param value The name of the peering between this cluster and the Google owned VPC.
*/
@JvmName("pjpybhorkeitcnse")
public suspend fun peeringName(`value`: Output) {
this.peeringName = value
}
/**
* @param value The internal IP address of this cluster's master endpoint.
*/
@JvmName("gamjwuiwmwdaynxw")
public suspend fun privateEndpoint(`value`: Output) {
this.privateEndpoint = value
}
/**
* @param value Subnetwork in cluster's network where master's endpoint will be provisioned.
*/
@JvmName("iahjlqivlavekxct")
public suspend fun privateEndpointSubnetwork(`value`: Output) {
this.privateEndpointSubnetwork = value
}
/**
* @param value The external IP address of this cluster's master endpoint.
* !> The Google provider is unable to validate certain configurations of
* `private_cluster_config` when `enable_private_nodes` is `false`. It's
* recommended that you omit the block entirely if the field is not set to `true`.
*/
@JvmName("egmjyvkbosiskyty")
public suspend fun publicEndpoint(`value`: Output) {
this.publicEndpoint = value
}
/**
* @param value When `true`, the cluster's private
* endpoint is used as the cluster endpoint and access through the public endpoint
* is disabled. When `false`, either endpoint can be used. This field only applies
* to private clusters, when `enable_private_nodes` is `true`.
*/
@JvmName("nshevffckmoynkrt")
public suspend fun enablePrivateEndpoint(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enablePrivateEndpoint = mapped
}
/**
* @param value Enables the private cluster feature,
* creating a private endpoint on the cluster. In a private cluster, nodes only
* have RFC 1918 private addresses and communicate with the master's private
* endpoint via private networking.
*/
@JvmName("sijdgmgvnbuepqcy")
public suspend fun enablePrivateNodes(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enablePrivateNodes = mapped
}
/**
* @param value Controls cluster master global
* access settings. If unset, the provider will no longer manage this field and will
* not modify the previously-set value. Structure is documented below.
*/
@JvmName("fotnstctxaxvyudo")
public suspend fun masterGlobalAccessConfig(`value`: ClusterPrivateClusterConfigMasterGlobalAccessConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.masterGlobalAccessConfig = mapped
}
/**
* @param argument Controls cluster master global
* access settings. If unset, the provider will no longer manage this field and will
* not modify the previously-set value. Structure is documented below.
*/
@JvmName("nefsymseddvihrpf")
public suspend fun masterGlobalAccessConfig(argument: suspend ClusterPrivateClusterConfigMasterGlobalAccessConfigArgsBuilder.() -> Unit) {
val toBeMapped = ClusterPrivateClusterConfigMasterGlobalAccessConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.masterGlobalAccessConfig = mapped
}
/**
* @param value The IP range in CIDR notation to use for
* the hosted master network. This range will be used for assigning private IP
* addresses to the cluster master(s) and the ILB VIP. This range must not overlap
* with any other ranges in use within the cluster's network, and it must be a /28
* subnet. See [Private Cluster Limitations](https://cloud.google.com/kubernetes-engine/docs/how-to/private-clusters#req_res_lim)
* for more details. This field only applies to private clusters, when
* `enable_private_nodes` is `true`.
*/
@JvmName("cygnjuyqiqsmrhdd")
public suspend fun masterIpv4CidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.masterIpv4CidrBlock = mapped
}
/**
* @param value The name of the peering between this cluster and the Google owned VPC.
*/
@JvmName("ljsgmqlfxngnqycw")
public suspend fun peeringName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.peeringName = mapped
}
/**
* @param value The internal IP address of this cluster's master endpoint.
*/
@JvmName("dgwyudmktxrprmrp")
public suspend fun privateEndpoint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateEndpoint = mapped
}
/**
* @param value Subnetwork in cluster's network where master's endpoint will be provisioned.
*/
@JvmName("tlujhgyuauuvmpuf")
public suspend fun privateEndpointSubnetwork(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateEndpointSubnetwork = mapped
}
/**
* @param value The external IP address of this cluster's master endpoint.
* !> The Google provider is unable to validate certain configurations of
* `private_cluster_config` when `enable_private_nodes` is `false`. It's
* recommended that you omit the block entirely if the field is not set to `true`.
*/
@JvmName("ocvtmilkmjggyiux")
public suspend fun publicEndpoint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publicEndpoint = mapped
}
internal fun build(): ClusterPrivateClusterConfigArgs = ClusterPrivateClusterConfigArgs(
enablePrivateEndpoint = enablePrivateEndpoint,
enablePrivateNodes = enablePrivateNodes,
masterGlobalAccessConfig = masterGlobalAccessConfig,
masterIpv4CidrBlock = masterIpv4CidrBlock,
peeringName = peeringName,
privateEndpoint = privateEndpoint,
privateEndpointSubnetwork = privateEndpointSubnetwork,
publicEndpoint = publicEndpoint,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy