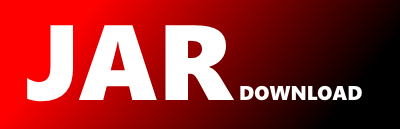
com.pulumi.gcp.container.kotlin.inputs.NodePoolUpgradeSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.container.inputs.NodePoolUpgradeSettingsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property blueGreenSettings The settings to adjust [blue green upgrades](https://cloud.google.com/kubernetes-engine/docs/concepts/node-pool-upgrade-strategies#blue-green-upgrade-strategy).
* Structure is documented below
* @property maxSurge The number of additional nodes that can be added to the node pool during
* an upgrade. Increasing `max_surge` raises the number of nodes that can be upgraded simultaneously.
* Can be set to 0 or greater.
* @property maxUnavailable The number of nodes that can be simultaneously unavailable during
* an upgrade. Increasing `max_unavailable` raises the number of nodes that can be upgraded in
* parallel. Can be set to 0 or greater.
* `max_surge` and `max_unavailable` must not be negative and at least one of them must be greater than zero.
* @property strategy The upgrade stragey to be used for upgrading the nodes.
*/
public data class NodePoolUpgradeSettingsArgs(
public val blueGreenSettings: Output? = null,
public val maxSurge: Output? = null,
public val maxUnavailable: Output? = null,
public val strategy: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.container.inputs.NodePoolUpgradeSettingsArgs =
com.pulumi.gcp.container.inputs.NodePoolUpgradeSettingsArgs.builder()
.blueGreenSettings(blueGreenSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maxSurge(maxSurge?.applyValue({ args0 -> args0 }))
.maxUnavailable(maxUnavailable?.applyValue({ args0 -> args0 }))
.strategy(strategy?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [NodePoolUpgradeSettingsArgs].
*/
@PulumiTagMarker
public class NodePoolUpgradeSettingsArgsBuilder internal constructor() {
private var blueGreenSettings: Output? = null
private var maxSurge: Output? = null
private var maxUnavailable: Output? = null
private var strategy: Output? = null
/**
* @param value The settings to adjust [blue green upgrades](https://cloud.google.com/kubernetes-engine/docs/concepts/node-pool-upgrade-strategies#blue-green-upgrade-strategy).
* Structure is documented below
*/
@JvmName("dgvpyhrhcibgsngm")
public suspend fun blueGreenSettings(`value`: Output) {
this.blueGreenSettings = value
}
/**
* @param value The number of additional nodes that can be added to the node pool during
* an upgrade. Increasing `max_surge` raises the number of nodes that can be upgraded simultaneously.
* Can be set to 0 or greater.
*/
@JvmName("blwrlgadfsxvncbk")
public suspend fun maxSurge(`value`: Output) {
this.maxSurge = value
}
/**
* @param value The number of nodes that can be simultaneously unavailable during
* an upgrade. Increasing `max_unavailable` raises the number of nodes that can be upgraded in
* parallel. Can be set to 0 or greater.
* `max_surge` and `max_unavailable` must not be negative and at least one of them must be greater than zero.
*/
@JvmName("loneynudwmfyepeb")
public suspend fun maxUnavailable(`value`: Output) {
this.maxUnavailable = value
}
/**
* @param value The upgrade stragey to be used for upgrading the nodes.
*/
@JvmName("rcqgbvxiwltwqikn")
public suspend fun strategy(`value`: Output) {
this.strategy = value
}
/**
* @param value The settings to adjust [blue green upgrades](https://cloud.google.com/kubernetes-engine/docs/concepts/node-pool-upgrade-strategies#blue-green-upgrade-strategy).
* Structure is documented below
*/
@JvmName("otkrvvnrstvowbvv")
public suspend fun blueGreenSettings(`value`: NodePoolUpgradeSettingsBlueGreenSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.blueGreenSettings = mapped
}
/**
* @param argument The settings to adjust [blue green upgrades](https://cloud.google.com/kubernetes-engine/docs/concepts/node-pool-upgrade-strategies#blue-green-upgrade-strategy).
* Structure is documented below
*/
@JvmName("ikpmwfbyhboslyvl")
public suspend fun blueGreenSettings(argument: suspend NodePoolUpgradeSettingsBlueGreenSettingsArgsBuilder.() -> Unit) {
val toBeMapped = NodePoolUpgradeSettingsBlueGreenSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.blueGreenSettings = mapped
}
/**
* @param value The number of additional nodes that can be added to the node pool during
* an upgrade. Increasing `max_surge` raises the number of nodes that can be upgraded simultaneously.
* Can be set to 0 or greater.
*/
@JvmName("ulivbfmlnexkiiit")
public suspend fun maxSurge(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxSurge = mapped
}
/**
* @param value The number of nodes that can be simultaneously unavailable during
* an upgrade. Increasing `max_unavailable` raises the number of nodes that can be upgraded in
* parallel. Can be set to 0 or greater.
* `max_surge` and `max_unavailable` must not be negative and at least one of them must be greater than zero.
*/
@JvmName("xyhuxmmijgjprgxw")
public suspend fun maxUnavailable(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxUnavailable = mapped
}
/**
* @param value The upgrade stragey to be used for upgrading the nodes.
*/
@JvmName("tkgorxedqidrikyx")
public suspend fun strategy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.strategy = mapped
}
internal fun build(): NodePoolUpgradeSettingsArgs = NodePoolUpgradeSettingsArgs(
blueGreenSettings = blueGreenSettings,
maxSurge = maxSurge,
maxUnavailable = maxUnavailable,
strategy = strategy,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy