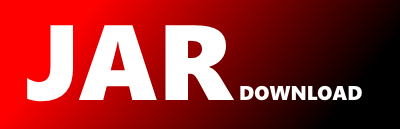
com.pulumi.gcp.container.kotlin.outputs.AwsClusterControlPlane.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property awsServicesAuthentication Authentication configuration for management of AWS resources.
* @property configEncryption The ARN of the AWS KMS key used to encrypt cluster configuration.
* @property databaseEncryption The ARN of the AWS KMS key used to encrypt cluster secrets.
* @property iamInstanceProfile The name of the AWS IAM instance pofile to assign to each control plane replica.
* @property instancePlacement Details of placement information for an instance.
* @property instanceType Optional. The AWS instance type. When unspecified, it defaults to `m5.large`.
* @property mainVolume Optional. Configuration related to the main volume provisioned for each control plane replica. The main volume is in charge of storing all of the cluster's etcd state. Volumes will be provisioned in the availability zone associated with the corresponding subnet. When unspecified, it defaults to 8 GiB with the GP2 volume type.
* @property proxyConfig Proxy configuration for outbound HTTP(S) traffic.
* @property rootVolume Optional. Configuration related to the root volume provisioned for each control plane replica. Volumes will be provisioned in the availability zone associated with the corresponding subnet. When unspecified, it defaults to 32 GiB with the GP2 volume type.
* @property securityGroupIds Optional. The IDs of additional security groups to add to control plane replicas. The Anthos Multi-Cloud API will automatically create and manage security groups with the minimum rules needed for a functioning cluster.
* @property sshConfig Optional. SSH configuration for how to access the underlying control plane machines.
* @property subnetIds The list of subnets where control plane replicas will run. A replica will be provisioned on each subnet and up to three values can be provided. Each subnet must be in a different AWS Availability Zone (AZ).
* @property tags Optional. A set of AWS resource tags to propagate to all underlying managed AWS resources. Specify at most 50 pairs containing alphanumerics, spaces, and symbols (.+-=_:@/). Keys can be up to 127 Unicode characters. Values can be up to 255 Unicode characters.
* @property version The Kubernetes version to run on control plane replicas (e.g. `1.19.10-gke.1000`). You can list all supported versions on a given Google Cloud region by calling .
*/
public data class AwsClusterControlPlane(
public val awsServicesAuthentication: AwsClusterControlPlaneAwsServicesAuthentication,
public val configEncryption: AwsClusterControlPlaneConfigEncryption,
public val databaseEncryption: AwsClusterControlPlaneDatabaseEncryption,
public val iamInstanceProfile: String,
public val instancePlacement: AwsClusterControlPlaneInstancePlacement? = null,
public val instanceType: String? = null,
public val mainVolume: AwsClusterControlPlaneMainVolume? = null,
public val proxyConfig: AwsClusterControlPlaneProxyConfig? = null,
public val rootVolume: AwsClusterControlPlaneRootVolume? = null,
public val securityGroupIds: List? = null,
public val sshConfig: AwsClusterControlPlaneSshConfig? = null,
public val subnetIds: List,
public val tags: Map? = null,
public val version: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.container.outputs.AwsClusterControlPlane): AwsClusterControlPlane = AwsClusterControlPlane(
awsServicesAuthentication = javaType.awsServicesAuthentication().let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsClusterControlPlaneAwsServicesAuthentication.Companion.toKotlin(args0)
}),
configEncryption = javaType.configEncryption().let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsClusterControlPlaneConfigEncryption.Companion.toKotlin(args0)
}),
databaseEncryption = javaType.databaseEncryption().let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsClusterControlPlaneDatabaseEncryption.Companion.toKotlin(args0)
}),
iamInstanceProfile = javaType.iamInstanceProfile(),
instancePlacement = javaType.instancePlacement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsClusterControlPlaneInstancePlacement.Companion.toKotlin(args0)
})
}).orElse(null),
instanceType = javaType.instanceType().map({ args0 -> args0 }).orElse(null),
mainVolume = javaType.mainVolume().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsClusterControlPlaneMainVolume.Companion.toKotlin(args0)
})
}).orElse(null),
proxyConfig = javaType.proxyConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsClusterControlPlaneProxyConfig.Companion.toKotlin(args0)
})
}).orElse(null),
rootVolume = javaType.rootVolume().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsClusterControlPlaneRootVolume.Companion.toKotlin(args0)
})
}).orElse(null),
securityGroupIds = javaType.securityGroupIds().map({ args0 -> args0 }),
sshConfig = javaType.sshConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsClusterControlPlaneSshConfig.Companion.toKotlin(args0)
})
}).orElse(null),
subnetIds = javaType.subnetIds().map({ args0 -> args0 }),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
version = javaType.version(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy