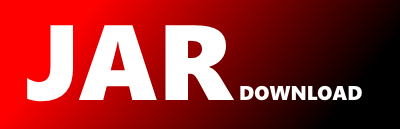
com.pulumi.gcp.container.kotlin.outputs.AwsNodePoolConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property autoscalingMetricsCollection Optional. Configuration related to CloudWatch metrics collection on the Auto Scaling group of the node pool. When unspecified, metrics collection is disabled.
* @property configEncryption The ARN of the AWS KMS key used to encrypt node pool configuration.
* @property iamInstanceProfile The name of the AWS IAM role assigned to nodes in the pool.
* @property imageType The OS image type to use on node pool instances.
* @property instancePlacement Details of placement information for an instance.
* @property instanceType Optional. The AWS instance type. When unspecified, it defaults to `m5.large`.
* @property labels Optional. The initial labels assigned to nodes of this node pool. An object containing a list of "key": value pairs. Example: { "name": "wrench", "mass": "1.3kg", "count": "3" }.
* @property proxyConfig Proxy configuration for outbound HTTP(S) traffic.
* @property rootVolume Optional. Template for the root volume provisioned for node pool nodes. Volumes will be provisioned in the availability zone assigned to the node pool subnet. When unspecified, it defaults to 32 GiB with the GP2 volume type.
* @property securityGroupIds Optional. The IDs of additional security groups to add to nodes in this pool. The manager will automatically create security groups with minimum rules needed for a functioning cluster.
* @property spotConfig Optional. When specified, the node pool will provision Spot instances from the set of spot_config.instance_types. This field is mutually exclusive with `instance_type`
* @property sshConfig Optional. The SSH configuration.
* @property tags Optional. Key/value metadata to assign to each underlying AWS resource. Specify at most 50 pairs containing alphanumerics, spaces, and symbols (.+-=_:@/). Keys can be up to 127 Unicode characters. Values can be up to 255 Unicode characters.
* @property taints Optional. The initial taints assigned to nodes of this node pool.
*/
public data class AwsNodePoolConfig(
public val autoscalingMetricsCollection: AwsNodePoolConfigAutoscalingMetricsCollection? = null,
public val configEncryption: AwsNodePoolConfigConfigEncryption,
public val iamInstanceProfile: String,
public val imageType: String? = null,
public val instancePlacement: AwsNodePoolConfigInstancePlacement? = null,
public val instanceType: String? = null,
public val labels: Map? = null,
public val proxyConfig: AwsNodePoolConfigProxyConfig? = null,
public val rootVolume: AwsNodePoolConfigRootVolume? = null,
public val securityGroupIds: List? = null,
public val spotConfig: AwsNodePoolConfigSpotConfig? = null,
public val sshConfig: AwsNodePoolConfigSshConfig? = null,
public val tags: Map? = null,
public val taints: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.container.outputs.AwsNodePoolConfig): AwsNodePoolConfig = AwsNodePoolConfig(
autoscalingMetricsCollection = javaType.autoscalingMetricsCollection().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsNodePoolConfigAutoscalingMetricsCollection.Companion.toKotlin(args0)
})
}).orElse(null),
configEncryption = javaType.configEncryption().let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsNodePoolConfigConfigEncryption.Companion.toKotlin(args0)
}),
iamInstanceProfile = javaType.iamInstanceProfile(),
imageType = javaType.imageType().map({ args0 -> args0 }).orElse(null),
instancePlacement = javaType.instancePlacement().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsNodePoolConfigInstancePlacement.Companion.toKotlin(args0)
})
}).orElse(null),
instanceType = javaType.instanceType().map({ args0 -> args0 }).orElse(null),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
proxyConfig = javaType.proxyConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsNodePoolConfigProxyConfig.Companion.toKotlin(args0)
})
}).orElse(null),
rootVolume = javaType.rootVolume().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsNodePoolConfigRootVolume.Companion.toKotlin(args0)
})
}).orElse(null),
securityGroupIds = javaType.securityGroupIds().map({ args0 -> args0 }),
spotConfig = javaType.spotConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsNodePoolConfigSpotConfig.Companion.toKotlin(args0)
})
}).orElse(null),
sshConfig = javaType.sshConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsNodePoolConfigSshConfig.Companion.toKotlin(args0)
})
}).orElse(null),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
taints = javaType.taints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.AwsNodePoolConfigTaint.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy