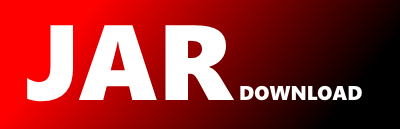
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.outputs
import kotlin.Suppress
/**
*
* @property cloudrunConfig . Structure is documented below.
* @property configConnectorConfig .
* The status of the ConfigConnector addon. It is disabled by default; Set `enabled = true` to enable.
* @property dnsCacheConfig .
* The status of the NodeLocal DNSCache addon. It is disabled by default.
* Set `enabled = true` to enable.
* **Enabling/Disabling NodeLocal DNSCache in an existing cluster is a disruptive operation.
* All cluster nodes running GKE 1.15 and higher are recreated.**
* @property gcePersistentDiskCsiDriverConfig .
* Whether this cluster should enable the Google Compute Engine Persistent Disk Container Storage Interface (CSI) Driver. Set `enabled = true` to enable.
* **Note:** The Compute Engine persistent disk CSI Driver is enabled by default on newly created clusters for the following versions: Linux clusters: GKE version 1.18.10-gke.2100 or later, or 1.19.3-gke.2100 or later.
* @property gcpFilestoreCsiDriverConfig The status of the Filestore CSI driver addon,
* which allows the usage of filestore instance as volumes.
* It is disabled by default; set `enabled = true` to enable.
* @property gcsFuseCsiDriverConfig The status of the GCSFuse CSI driver addon,
* which allows the usage of a gcs bucket as volumes.
* It is disabled by default for Standard clusters; set `enabled = true` to enable.
* It is enabled by default for Autopilot clusters with version 1.24 or later; set `enabled = true` to enable it explicitly.
* See [Enable the Cloud Storage FUSE CSI driver](https://cloud.google.com/kubernetes-engine/docs/how-to/persistent-volumes/cloud-storage-fuse-csi-driver#enable) for more information.
* @property gkeBackupAgentConfig .
* The status of the Backup for GKE agent addon. It is disabled by default; Set `enabled = true` to enable.
* @property horizontalPodAutoscaling The status of the Horizontal Pod Autoscaling
* addon, which increases or decreases the number of replica pods a replication controller
* has based on the resource usage of the existing pods.
* It is enabled by default;
* set `disabled = true` to disable.
* @property httpLoadBalancing The status of the HTTP (L7) load balancing
* controller addon, which makes it easy to set up HTTP load balancers for services in a
* cluster. It is enabled by default; set `disabled = true` to disable.
* @property istioConfig .
* Structure is documented below.
* @property kalmConfig .
* Configuration for the KALM addon, which manages the lifecycle of k8s. It is disabled by default; Set `enabled = true` to enable.
* @property networkPolicyConfig Whether we should enable the network policy addon
* for the master. This must be enabled in order to enable network policy for the nodes.
* To enable this, you must also define a `network_policy` block,
* otherwise nothing will happen.
* It can only be disabled if the nodes already do not have network policies enabled.
* Defaults to disabled; set `disabled = false` to enable.
* @property statefulHaConfig .
* The status of the Stateful HA addon, which provides automatic configurable failover for stateful applications.
* It is disabled by default for Standard clusters. Set `enabled = true` to enable.
* This example `addons_config` disables two addons:
*/
public data class ClusterAddonsConfig(
public val cloudrunConfig: ClusterAddonsConfigCloudrunConfig? = null,
public val configConnectorConfig: ClusterAddonsConfigConfigConnectorConfig? = null,
public val dnsCacheConfig: ClusterAddonsConfigDnsCacheConfig? = null,
public val gcePersistentDiskCsiDriverConfig: ClusterAddonsConfigGcePersistentDiskCsiDriverConfig? =
null,
public val gcpFilestoreCsiDriverConfig: ClusterAddonsConfigGcpFilestoreCsiDriverConfig? = null,
public val gcsFuseCsiDriverConfig: ClusterAddonsConfigGcsFuseCsiDriverConfig? = null,
public val gkeBackupAgentConfig: ClusterAddonsConfigGkeBackupAgentConfig? = null,
public val horizontalPodAutoscaling: ClusterAddonsConfigHorizontalPodAutoscaling? = null,
public val httpLoadBalancing: ClusterAddonsConfigHttpLoadBalancing? = null,
public val istioConfig: ClusterAddonsConfigIstioConfig? = null,
public val kalmConfig: ClusterAddonsConfigKalmConfig? = null,
public val networkPolicyConfig: ClusterAddonsConfigNetworkPolicyConfig? = null,
public val statefulHaConfig: ClusterAddonsConfigStatefulHaConfig? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.container.outputs.ClusterAddonsConfig): ClusterAddonsConfig = ClusterAddonsConfig(
cloudrunConfig = javaType.cloudrunConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigCloudrunConfig.Companion.toKotlin(args0)
})
}).orElse(null),
configConnectorConfig = javaType.configConnectorConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigConfigConnectorConfig.Companion.toKotlin(args0)
})
}).orElse(null),
dnsCacheConfig = javaType.dnsCacheConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigDnsCacheConfig.Companion.toKotlin(args0)
})
}).orElse(null),
gcePersistentDiskCsiDriverConfig = javaType.gcePersistentDiskCsiDriverConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigGcePersistentDiskCsiDriverConfig.Companion.toKotlin(args0)
})
}).orElse(null),
gcpFilestoreCsiDriverConfig = javaType.gcpFilestoreCsiDriverConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigGcpFilestoreCsiDriverConfig.Companion.toKotlin(args0)
})
}).orElse(null),
gcsFuseCsiDriverConfig = javaType.gcsFuseCsiDriverConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigGcsFuseCsiDriverConfig.Companion.toKotlin(args0)
})
}).orElse(null),
gkeBackupAgentConfig = javaType.gkeBackupAgentConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigGkeBackupAgentConfig.Companion.toKotlin(args0)
})
}).orElse(null),
horizontalPodAutoscaling = javaType.horizontalPodAutoscaling().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigHorizontalPodAutoscaling.Companion.toKotlin(args0)
})
}).orElse(null),
httpLoadBalancing = javaType.httpLoadBalancing().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigHttpLoadBalancing.Companion.toKotlin(args0)
})
}).orElse(null),
istioConfig = javaType.istioConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigIstioConfig.Companion.toKotlin(args0)
})
}).orElse(null),
kalmConfig = javaType.kalmConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigKalmConfig.Companion.toKotlin(args0)
})
}).orElse(null),
networkPolicyConfig = javaType.networkPolicyConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigNetworkPolicyConfig.Companion.toKotlin(args0)
})
}).orElse(null),
statefulHaConfig = javaType.statefulHaConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterAddonsConfigStatefulHaConfig.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy