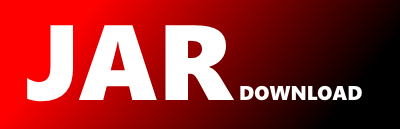
com.pulumi.gcp.container.kotlin.outputs.ClusterNodePool.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property autoscaling Configuration required by cluster autoscaler to adjust the size of the node pool to the current cluster usage.
* @property initialNodeCount The number of nodes to create in this
* cluster's default node pool. In regional or multi-zonal clusters, this is the
* number of nodes per zone. Must be set if `node_pool` is not set. If you're using
* `gcp.container.NodePool` objects with no default node pool, you'll need to
* set this to a value of at least `1`, alongside setting
* `remove_default_node_pool` to `true`.
* @property instanceGroupUrls The resource URLs of the managed instance groups associated with this node pool.
* @property managedInstanceGroupUrls List of instance group URLs which have been assigned to this node pool.
* @property management Node management configuration, wherein auto-repair and auto-upgrade is configured.
* @property maxPodsPerNode The maximum number of pods per node in this node pool. Note that this does not work on node pools which are "route-based" - that is, node pools belonging to clusters that do not have IP Aliasing enabled.
* @property name The name of the cluster, unique within the project and
* location.
* - - -
* @property namePrefix Creates a unique name for the node pool beginning with the specified prefix. Conflicts with name.
* @property networkConfig Configuration for
* [Adding Pod IP address ranges](https://cloud.google.com/kubernetes-engine/docs/how-to/multi-pod-cidr)) to the node pool. Structure is documented below
* @property nodeConfig Parameters used in creating the default node pool.
* Generally, this field should not be used at the same time as a
* `gcp.container.NodePool` or a `node_pool` block; this configuration
* manages the default node pool, which isn't recommended to be used.
* Structure is documented below.
* @property nodeCount The number of nodes per instance group. This field can be used to update the number of nodes per instance group but should not be used alongside autoscaling.
* @property nodeLocations The list of zones in which the cluster's nodes
* are located. Nodes must be in the region of their regional cluster or in the
* same region as their cluster's zone for zonal clusters. If this is specified for
* a zonal cluster, omit the cluster's zone.
* > A "multi-zonal" cluster is a zonal cluster with at least one additional zone
* defined; in a multi-zonal cluster, the cluster master is only present in a
* single zone while nodes are present in each of the primary zone and the node
* locations. In contrast, in a regional cluster, cluster master nodes are present
* in multiple zones in the region. For that reason, regional clusters should be
* preferred.
* @property placementPolicy Specifies the node placement policy
* @property queuedProvisioning Specifies the configuration of queued provisioning
* @property upgradeSettings Specify node upgrade settings to change how many nodes GKE attempts to upgrade at once. The number of nodes upgraded simultaneously is the sum of max_surge and max_unavailable. The maximum number of nodes upgraded simultaneously is limited to 20.
* @property version
*/
public data class ClusterNodePool(
public val autoscaling: ClusterNodePoolAutoscaling? = null,
public val initialNodeCount: Int? = null,
public val instanceGroupUrls: List? = null,
public val managedInstanceGroupUrls: List? = null,
public val management: ClusterNodePoolManagement? = null,
public val maxPodsPerNode: Int? = null,
public val name: String? = null,
public val namePrefix: String? = null,
public val networkConfig: ClusterNodePoolNetworkConfig? = null,
public val nodeConfig: ClusterNodePoolNodeConfig? = null,
public val nodeCount: Int? = null,
public val nodeLocations: List? = null,
public val placementPolicy: ClusterNodePoolPlacementPolicy? = null,
public val queuedProvisioning: ClusterNodePoolQueuedProvisioning? = null,
public val upgradeSettings: ClusterNodePoolUpgradeSettings? = null,
public val version: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.container.outputs.ClusterNodePool): ClusterNodePool = ClusterNodePool(
autoscaling = javaType.autoscaling().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterNodePoolAutoscaling.Companion.toKotlin(args0)
})
}).orElse(null),
initialNodeCount = javaType.initialNodeCount().map({ args0 -> args0 }).orElse(null),
instanceGroupUrls = javaType.instanceGroupUrls().map({ args0 -> args0 }),
managedInstanceGroupUrls = javaType.managedInstanceGroupUrls().map({ args0 -> args0 }),
management = javaType.management().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterNodePoolManagement.Companion.toKotlin(args0)
})
}).orElse(null),
maxPodsPerNode = javaType.maxPodsPerNode().map({ args0 -> args0 }).orElse(null),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
namePrefix = javaType.namePrefix().map({ args0 -> args0 }).orElse(null),
networkConfig = javaType.networkConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterNodePoolNetworkConfig.Companion.toKotlin(args0)
})
}).orElse(null),
nodeConfig = javaType.nodeConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterNodePoolNodeConfig.Companion.toKotlin(args0)
})
}).orElse(null),
nodeCount = javaType.nodeCount().map({ args0 -> args0 }).orElse(null),
nodeLocations = javaType.nodeLocations().map({ args0 -> args0 }),
placementPolicy = javaType.placementPolicy().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterNodePoolPlacementPolicy.Companion.toKotlin(args0)
})
}).orElse(null),
queuedProvisioning = javaType.queuedProvisioning().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterNodePoolQueuedProvisioning.Companion.toKotlin(args0)
})
}).orElse(null),
upgradeSettings = javaType.upgradeSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.ClusterNodePoolUpgradeSettings.Companion.toKotlin(args0)
})
}).orElse(null),
version = javaType.version().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy