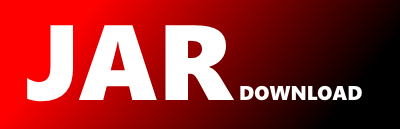
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.outputs
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property cloudrunConfigs The status of the CloudRun addon. It is disabled by default. Set disabled = false to enable.
* @property configConnectorConfigs The of the Config Connector addon.
* @property dnsCacheConfigs The status of the NodeLocal DNSCache addon. It is disabled by default. Set enabled = true to enable.
* @property gcePersistentDiskCsiDriverConfigs Whether this cluster should enable the Google Compute Engine Persistent Disk Container Storage Interface (CSI) Driver. Set enabled = true to enable. The Compute Engine persistent disk CSI Driver is enabled by default on newly created clusters for the following versions: Linux clusters: GKE version 1.18.10-gke.2100 or later, or 1.19.3-gke.2100 or later.
* @property gcpFilestoreCsiDriverConfigs The status of the Filestore CSI driver addon, which allows the usage of filestore instance as volumes. Defaults to disabled; set enabled = true to enable.
* @property gcsFuseCsiDriverConfigs The status of the GCS Fuse CSI driver addon, which allows the usage of gcs bucket as volumes. Defaults to disabled; set enabled = true to enable.
* @property gkeBackupAgentConfigs The status of the Backup for GKE Agent addon. It is disabled by default. Set enabled = true to enable.
* @property horizontalPodAutoscalings The status of the Horizontal Pod Autoscaling addon, which increases or decreases the number of replica pods a replication controller has based on the resource usage of the existing pods. It ensures that a Heapster pod is running in the cluster, which is also used by the Cloud Monitoring service. It is enabled by default; set disabled = true to disable.
* @property httpLoadBalancings The status of the HTTP (L7) load balancing controller addon, which makes it easy to set up HTTP load balancers for services in a cluster. It is enabled by default; set disabled = true to disable.
* @property istioConfigs The status of the Istio addon.
* @property kalmConfigs Configuration for the KALM addon, which manages the lifecycle of k8s. It is disabled by default; Set enabled = true to enable.
* @property networkPolicyConfigs Whether we should enable the network policy addon for the master. This must be enabled in order to enable network policy for the nodes. To enable this, you must also define a network_policy block, otherwise nothing will happen. It can only be disabled if the nodes already do not have network policies enabled. Defaults to disabled; set disabled = false to enable.
* @property statefulHaConfigs The status of the Stateful HA addon, which provides automatic configurable failover for stateful applications. Defaults to disabled; set enabled = true to enable.
*/
public data class GetClusterAddonsConfig(
public val cloudrunConfigs: List,
public val configConnectorConfigs: List,
public val dnsCacheConfigs: List,
public val gcePersistentDiskCsiDriverConfigs: List,
public val gcpFilestoreCsiDriverConfigs: List,
public val gcsFuseCsiDriverConfigs: List,
public val gkeBackupAgentConfigs: List,
public val horizontalPodAutoscalings: List,
public val httpLoadBalancings: List,
public val istioConfigs: List,
public val kalmConfigs: List,
public val networkPolicyConfigs: List,
public val statefulHaConfigs: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.container.outputs.GetClusterAddonsConfig): GetClusterAddonsConfig = GetClusterAddonsConfig(
cloudrunConfigs = javaType.cloudrunConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigCloudrunConfig.Companion.toKotlin(args0)
})
}),
configConnectorConfigs = javaType.configConnectorConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigConfigConnectorConfig.Companion.toKotlin(args0)
})
}),
dnsCacheConfigs = javaType.dnsCacheConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigDnsCacheConfig.Companion.toKotlin(args0)
})
}),
gcePersistentDiskCsiDriverConfigs = javaType.gcePersistentDiskCsiDriverConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigGcePersistentDiskCsiDriverConfig.Companion.toKotlin(args0)
})
}),
gcpFilestoreCsiDriverConfigs = javaType.gcpFilestoreCsiDriverConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigGcpFilestoreCsiDriverConfig.Companion.toKotlin(args0)
})
}),
gcsFuseCsiDriverConfigs = javaType.gcsFuseCsiDriverConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigGcsFuseCsiDriverConfig.Companion.toKotlin(args0)
})
}),
gkeBackupAgentConfigs = javaType.gkeBackupAgentConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigGkeBackupAgentConfig.Companion.toKotlin(args0)
})
}),
horizontalPodAutoscalings = javaType.horizontalPodAutoscalings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigHorizontalPodAutoscaling.Companion.toKotlin(args0)
})
}),
httpLoadBalancings = javaType.httpLoadBalancings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigHttpLoadBalancing.Companion.toKotlin(args0)
})
}),
istioConfigs = javaType.istioConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigIstioConfig.Companion.toKotlin(args0)
})
}),
kalmConfigs = javaType.kalmConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigKalmConfig.Companion.toKotlin(args0)
})
}),
networkPolicyConfigs = javaType.networkPolicyConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigNetworkPolicyConfig.Companion.toKotlin(args0)
})
}),
statefulHaConfigs = javaType.statefulHaConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterAddonsConfigStatefulHaConfig.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy