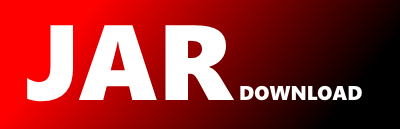
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePool.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property autoscalings Configuration required by cluster autoscaler to adjust the size of the node pool to the current cluster usage.
* @property initialNodeCount The initial number of nodes for the pool. In regional or multi-zonal clusters, this is the number of nodes per zone. Changing this will force recreation of the resource.
* @property instanceGroupUrls The resource URLs of the managed instance groups associated with this node pool.
* @property managedInstanceGroupUrls List of instance group URLs which have been assigned to this node pool.
* @property managements Node management configuration, wherein auto-repair and auto-upgrade is configured.
* @property maxPodsPerNode The maximum number of pods per node in this node pool. Note that this does not work on node pools which are "route-based" - that is, node pools belonging to clusters that do not have IP Aliasing enabled.
* @property name The name of the cluster.
* @property namePrefix Creates a unique name for the node pool beginning with the specified prefix. Conflicts with name.
* @property networkConfigs Networking configuration for this NodePool. If specified, it overrides the cluster-level defaults.
* @property nodeConfigs The configuration of the nodepool
* @property nodeCount The number of nodes per instance group. This field can be used to update the number of nodes per instance group but should not be used alongside autoscaling.
* @property nodeLocations The list of zones in which the node pool's nodes should be located. Nodes must be in the region of their regional cluster or in the same region as their cluster's zone for zonal clusters. If unspecified, the cluster-level node_locations will be used.
* @property placementPolicies Specifies the node placement policy
* @property queuedProvisionings Specifies the configuration of queued provisioning
* @property upgradeSettings Specify node upgrade settings to change how many nodes GKE attempts to upgrade at once. The number of nodes upgraded simultaneously is the sum of max_surge and max_unavailable. The maximum number of nodes upgraded simultaneously is limited to 20.
* @property version
*/
public data class GetClusterNodePool(
public val autoscalings: List,
public val initialNodeCount: Int,
public val instanceGroupUrls: List,
public val managedInstanceGroupUrls: List,
public val managements: List,
public val maxPodsPerNode: Int,
public val name: String,
public val namePrefix: String,
public val networkConfigs: List,
public val nodeConfigs: List,
public val nodeCount: Int,
public val nodeLocations: List,
public val placementPolicies: List,
public val queuedProvisionings: List,
public val upgradeSettings: List,
public val version: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.container.outputs.GetClusterNodePool): GetClusterNodePool = GetClusterNodePool(
autoscalings = javaType.autoscalings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolAutoscaling.Companion.toKotlin(args0)
})
}),
initialNodeCount = javaType.initialNodeCount(),
instanceGroupUrls = javaType.instanceGroupUrls().map({ args0 -> args0 }),
managedInstanceGroupUrls = javaType.managedInstanceGroupUrls().map({ args0 -> args0 }),
managements = javaType.managements().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolManagement.Companion.toKotlin(args0)
})
}),
maxPodsPerNode = javaType.maxPodsPerNode(),
name = javaType.name(),
namePrefix = javaType.namePrefix(),
networkConfigs = javaType.networkConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNetworkConfig.Companion.toKotlin(args0)
})
}),
nodeConfigs = javaType.nodeConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfig.Companion.toKotlin(args0)
})
}),
nodeCount = javaType.nodeCount(),
nodeLocations = javaType.nodeLocations().map({ args0 -> args0 }),
placementPolicies = javaType.placementPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolPlacementPolicy.Companion.toKotlin(args0)
})
}),
queuedProvisionings = javaType.queuedProvisionings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolQueuedProvisioning.Companion.toKotlin(args0)
})
}),
upgradeSettings = javaType.upgradeSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolUpgradeSetting.Companion.toKotlin(args0)
})
}),
version = javaType.version(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy