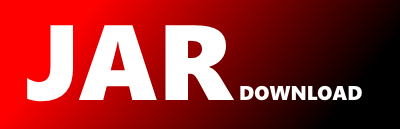
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.outputs
import kotlin.Any
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property advancedMachineFeatures Specifies options for controlling advanced machine features.
* @property bootDiskKmsKey The Customer Managed Encryption Key used to encrypt the boot disk attached to each node in the node pool.
* @property confidentialNodes Configuration for the confidential nodes feature, which makes nodes run on confidential VMs. Warning: This configuration can't be changed (or added/removed) after pool creation without deleting and recreating the entire pool.
* @property diskSizeGb Size of the disk attached to each node, specified in GB. The smallest allowed disk size is 10GB.
* @property diskType Type of the disk attached to each node. Such as pd-standard, pd-balanced or pd-ssd
* @property effectiveTaints List of kubernetes taints applied to each node.
* @property enableConfidentialStorage If enabled boot disks are configured with confidential mode.
* @property ephemeralStorageConfigs Parameters for the ephemeral storage filesystem. If unspecified, ephemeral storage is backed by the boot disk.
* @property ephemeralStorageLocalSsdConfigs Parameters for the ephemeral storage filesystem. If unspecified, ephemeral storage is backed by the boot disk.
* @property fastSockets Enable or disable NCCL Fast Socket in the node pool.
* @property gcfsConfigs GCFS configuration for this node.
* @property guestAccelerators List of the type and count of accelerator cards attached to the instance.
* @property gvnics Enable or disable gvnic in the node pool.
* @property hostMaintenancePolicies The maintenance policy for the hosts on which the GKE VMs run on.
* @property imageType The image type to use for this node. Note that for a given image type, the latest version of it will be used.
* @property kubeletConfigs Node kubelet configs.
* @property labels The map of Kubernetes labels (key/value pairs) to be applied to each node. These will added in addition to any default label(s) that Kubernetes may apply to the node.
* @property linuxNodeConfigs Parameters that can be configured on Linux nodes.
* @property localNvmeSsdBlockConfigs Parameters for raw-block local NVMe SSDs.
* @property localSsdCount The number of local SSD disks to be attached to the node.
* @property loggingVariant Type of logging agent that is used as the default value for node pools in the cluster. Valid values include DEFAULT and MAX_THROUGHPUT.
* @property machineType The name of a Google Compute Engine machine type.
* @property metadata The metadata key/value pairs assigned to instances in the cluster.
* @property minCpuPlatform Minimum CPU platform to be used by this instance. The instance may be scheduled on the specified or newer CPU platform.
* @property nodeGroup Setting this field will assign instances of this pool to run on the specified node group. This is useful for running workloads on sole tenant nodes.
* @property oauthScopes The set of Google API scopes to be made available on all of the node VMs.
* @property preemptible Whether the nodes are created as preemptible VM instances.
* @property reservationAffinities The reservation affinity configuration for the node pool.
* @property resourceLabels The GCE resource labels (a map of key/value pairs) to be applied to the node pool.
* @property resourceManagerTags A map of resource manager tags. Resource manager tag keys and values have the same definition as resource manager tags. Keys must be in the format tagKeys/{tag_key_id}, and values are in the format tagValues/456. The field is ignored (both PUT & PATCH) when empty.
* @property sandboxConfigs Sandbox configuration for this node.
* @property secondaryBootDisks Secondary boot disks for preloading data or container images.
* @property serviceAccount The Google Cloud Platform Service Account to be used by the node VMs.
* @property shieldedInstanceConfigs Shielded Instance options.
* @property soleTenantConfigs Node affinity options for sole tenant node pools.
* @property spot Whether the nodes are created as spot VM instances.
* @property tags The list of instance tags applied to all nodes.
* @property taints List of Kubernetes taints to be applied to each node.
* @property workloadMetadataConfigs The workload metadata configuration for this node.
*/
public data class GetClusterNodePoolNodeConfig(
public val advancedMachineFeatures: List,
public val bootDiskKmsKey: String,
public val confidentialNodes: List,
public val diskSizeGb: Int,
public val diskType: String,
public val effectiveTaints: List,
public val enableConfidentialStorage: Boolean,
public val ephemeralStorageConfigs: List,
public val ephemeralStorageLocalSsdConfigs: List,
public val fastSockets: List,
public val gcfsConfigs: List,
public val guestAccelerators: List,
public val gvnics: List,
public val hostMaintenancePolicies: List,
public val imageType: String,
public val kubeletConfigs: List,
public val labels: Map,
public val linuxNodeConfigs: List,
public val localNvmeSsdBlockConfigs: List,
public val localSsdCount: Int,
public val loggingVariant: String,
public val machineType: String,
public val metadata: Map,
public val minCpuPlatform: String,
public val nodeGroup: String,
public val oauthScopes: List,
public val preemptible: Boolean,
public val reservationAffinities: List,
public val resourceLabels: Map,
public val resourceManagerTags: Map,
public val sandboxConfigs: List,
public val secondaryBootDisks: List,
public val serviceAccount: String,
public val shieldedInstanceConfigs: List,
public val soleTenantConfigs: List,
public val spot: Boolean,
public val tags: List,
public val taints: List,
public val workloadMetadataConfigs: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.container.outputs.GetClusterNodePoolNodeConfig): GetClusterNodePoolNodeConfig = GetClusterNodePoolNodeConfig(
advancedMachineFeatures = javaType.advancedMachineFeatures().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigAdvancedMachineFeature.Companion.toKotlin(args0)
})
}),
bootDiskKmsKey = javaType.bootDiskKmsKey(),
confidentialNodes = javaType.confidentialNodes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigConfidentialNode.Companion.toKotlin(args0)
})
}),
diskSizeGb = javaType.diskSizeGb(),
diskType = javaType.diskType(),
effectiveTaints = javaType.effectiveTaints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigEffectiveTaint.Companion.toKotlin(args0)
})
}),
enableConfidentialStorage = javaType.enableConfidentialStorage(),
ephemeralStorageConfigs = javaType.ephemeralStorageConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigEphemeralStorageConfig.Companion.toKotlin(args0)
})
}),
ephemeralStorageLocalSsdConfigs = javaType.ephemeralStorageLocalSsdConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigEphemeralStorageLocalSsdConfig.Companion.toKotlin(args0)
})
}),
fastSockets = javaType.fastSockets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigFastSocket.Companion.toKotlin(args0)
})
}),
gcfsConfigs = javaType.gcfsConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigGcfsConfig.Companion.toKotlin(args0)
})
}),
guestAccelerators = javaType.guestAccelerators().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigGuestAccelerator.Companion.toKotlin(args0)
})
}),
gvnics = javaType.gvnics().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigGvnic.Companion.toKotlin(args0)
})
}),
hostMaintenancePolicies = javaType.hostMaintenancePolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigHostMaintenancePolicy.Companion.toKotlin(args0)
})
}),
imageType = javaType.imageType(),
kubeletConfigs = javaType.kubeletConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigKubeletConfig.Companion.toKotlin(args0)
})
}),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
linuxNodeConfigs = javaType.linuxNodeConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigLinuxNodeConfig.Companion.toKotlin(args0)
})
}),
localNvmeSsdBlockConfigs = javaType.localNvmeSsdBlockConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigLocalNvmeSsdBlockConfig.Companion.toKotlin(args0)
})
}),
localSsdCount = javaType.localSsdCount(),
loggingVariant = javaType.loggingVariant(),
machineType = javaType.machineType(),
metadata = javaType.metadata().map({ args0 -> args0.key.to(args0.value) }).toMap(),
minCpuPlatform = javaType.minCpuPlatform(),
nodeGroup = javaType.nodeGroup(),
oauthScopes = javaType.oauthScopes().map({ args0 -> args0 }),
preemptible = javaType.preemptible(),
reservationAffinities = javaType.reservationAffinities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigReservationAffinity.Companion.toKotlin(args0)
})
}),
resourceLabels = javaType.resourceLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
resourceManagerTags = javaType.resourceManagerTags().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
sandboxConfigs = javaType.sandboxConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigSandboxConfig.Companion.toKotlin(args0)
})
}),
secondaryBootDisks = javaType.secondaryBootDisks().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigSecondaryBootDisk.Companion.toKotlin(args0)
})
}),
serviceAccount = javaType.serviceAccount(),
shieldedInstanceConfigs = javaType.shieldedInstanceConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigShieldedInstanceConfig.Companion.toKotlin(args0)
})
}),
soleTenantConfigs = javaType.soleTenantConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigSoleTenantConfig.Companion.toKotlin(args0)
})
}),
spot = javaType.spot(),
tags = javaType.tags().map({ args0 -> args0 }),
taints = javaType.taints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigTaint.Companion.toKotlin(args0)
})
}),
workloadMetadataConfigs = javaType.workloadMetadataConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.GetClusterNodePoolNodeConfigWorkloadMetadataConfig.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy