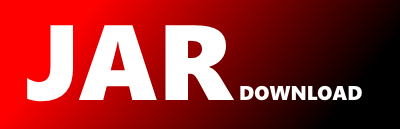
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin.outputs
import kotlin.Any
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property advancedMachineFeatures Specifies options for controlling advanced machine features.
* @property bootDiskKmsKey The Customer Managed Encryption Key used to encrypt the boot disk attached to each node in the node pool.
* @property confidentialNodes Configuration for Confidential Nodes feature. Structure is documented below.
* @property diskSizeGb Size of the disk attached to each node, specified in GB. The smallest allowed disk size is 10GB.
* @property diskType Type of the disk attached to each node. Such as pd-standard, pd-balanced or pd-ssd
* @property effectiveTaints List of kubernetes taints applied to each node.
* @property enableConfidentialStorage If enabled boot disks are configured with confidential mode.
* @property ephemeralStorageConfig Parameters for the ephemeral storage filesystem. If unspecified, ephemeral storage is backed by the boot disk.
* @property ephemeralStorageLocalSsdConfig Parameters for the ephemeral storage filesystem. If unspecified, ephemeral storage is backed by the boot disk.
* @property fastSocket Enable or disable NCCL Fast Socket in the node pool.
* @property gcfsConfig GCFS configuration for this node.
* @property guestAccelerators List of the type and count of accelerator cards attached to the instance.
* @property gvnic Enable or disable gvnic in the node pool.
* @property hostMaintenancePolicy The maintenance policy for the hosts on which the GKE VMs run on.
* @property imageType The image type to use for this node. Note that for a given image type, the latest version of it will be used.
* @property kubeletConfig Node kubelet configs.
* @property labels The map of Kubernetes labels (key/value pairs) to be applied to each node. These will added in addition to any default label(s) that Kubernetes may apply to the node.
* @property linuxNodeConfig Parameters that can be configured on Linux nodes.
* @property localNvmeSsdBlockConfig Parameters for raw-block local NVMe SSDs.
* @property localSsdCount The number of local SSD disks to be attached to the node.
* @property loggingVariant Type of logging agent that is used as the default value for node pools in the cluster. Valid values include DEFAULT and MAX_THROUGHPUT.
* @property machineType The name of a Google Compute Engine machine type.
* @property metadata The metadata key/value pairs assigned to instances in the cluster.
* @property minCpuPlatform Minimum CPU platform to be used by this instance. The instance may be scheduled on the specified or newer CPU platform.
* @property nodeGroup Setting this field will assign instances of this pool to run on the specified node group. This is useful for running workloads on sole tenant nodes.
* @property oauthScopes The set of Google API scopes to be made available on all of the node VMs.
* @property preemptible Whether the nodes are created as preemptible VM instances.
* @property reservationAffinity The reservation affinity configuration for the node pool.
* @property resourceLabels The GCE resource labels (a map of key/value pairs) to be applied to the node pool.
* @property resourceManagerTags A map of resource manager tags. Resource manager tag keys and values have the same definition as resource manager tags. Keys must be in the format tagKeys/{tag_key_id}, and values are in the format tagValues/456. The field is ignored (both PUT & PATCH) when empty.
* @property sandboxConfig Sandbox configuration for this node.
* @property secondaryBootDisks Secondary boot disks for preloading data or container images.
* @property serviceAccount The Google Cloud Platform Service Account to be used by the node VMs.
* @property shieldedInstanceConfig Shielded Instance options.
* @property soleTenantConfig Node affinity options for sole tenant node pools.
* @property spot Whether the nodes are created as spot VM instances.
* @property tags The list of instance tags applied to all nodes.
* @property taints List of Kubernetes taints to be applied to each node.
* @property workloadMetadataConfig The workload metadata configuration for this node.
*/
public data class NodePoolNodeConfig(
public val advancedMachineFeatures: NodePoolNodeConfigAdvancedMachineFeatures? = null,
public val bootDiskKmsKey: String? = null,
public val confidentialNodes: NodePoolNodeConfigConfidentialNodes? = null,
public val diskSizeGb: Int? = null,
public val diskType: String? = null,
public val effectiveTaints: List? = null,
public val enableConfidentialStorage: Boolean? = null,
public val ephemeralStorageConfig: NodePoolNodeConfigEphemeralStorageConfig? = null,
public val ephemeralStorageLocalSsdConfig: NodePoolNodeConfigEphemeralStorageLocalSsdConfig? =
null,
public val fastSocket: NodePoolNodeConfigFastSocket? = null,
public val gcfsConfig: NodePoolNodeConfigGcfsConfig? = null,
public val guestAccelerators: List? = null,
public val gvnic: NodePoolNodeConfigGvnic? = null,
public val hostMaintenancePolicy: NodePoolNodeConfigHostMaintenancePolicy? = null,
public val imageType: String? = null,
public val kubeletConfig: NodePoolNodeConfigKubeletConfig? = null,
public val labels: Map? = null,
public val linuxNodeConfig: NodePoolNodeConfigLinuxNodeConfig? = null,
public val localNvmeSsdBlockConfig: NodePoolNodeConfigLocalNvmeSsdBlockConfig? = null,
public val localSsdCount: Int? = null,
public val loggingVariant: String? = null,
public val machineType: String? = null,
public val metadata: Map? = null,
public val minCpuPlatform: String? = null,
public val nodeGroup: String? = null,
public val oauthScopes: List? = null,
public val preemptible: Boolean? = null,
public val reservationAffinity: NodePoolNodeConfigReservationAffinity? = null,
public val resourceLabels: Map? = null,
public val resourceManagerTags: Map? = null,
public val sandboxConfig: NodePoolNodeConfigSandboxConfig? = null,
public val secondaryBootDisks: List? = null,
public val serviceAccount: String? = null,
public val shieldedInstanceConfig: NodePoolNodeConfigShieldedInstanceConfig? = null,
public val soleTenantConfig: NodePoolNodeConfigSoleTenantConfig? = null,
public val spot: Boolean? = null,
public val tags: List? = null,
public val taints: List? = null,
public val workloadMetadataConfig: NodePoolNodeConfigWorkloadMetadataConfig? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.container.outputs.NodePoolNodeConfig): NodePoolNodeConfig = NodePoolNodeConfig(
advancedMachineFeatures = javaType.advancedMachineFeatures().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigAdvancedMachineFeatures.Companion.toKotlin(args0)
})
}).orElse(null),
bootDiskKmsKey = javaType.bootDiskKmsKey().map({ args0 -> args0 }).orElse(null),
confidentialNodes = javaType.confidentialNodes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigConfidentialNodes.Companion.toKotlin(args0)
})
}).orElse(null),
diskSizeGb = javaType.diskSizeGb().map({ args0 -> args0 }).orElse(null),
diskType = javaType.diskType().map({ args0 -> args0 }).orElse(null),
effectiveTaints = javaType.effectiveTaints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigEffectiveTaint.Companion.toKotlin(args0)
})
}),
enableConfidentialStorage = javaType.enableConfidentialStorage().map({ args0 ->
args0
}).orElse(null),
ephemeralStorageConfig = javaType.ephemeralStorageConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigEphemeralStorageConfig.Companion.toKotlin(args0)
})
}).orElse(null),
ephemeralStorageLocalSsdConfig = javaType.ephemeralStorageLocalSsdConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigEphemeralStorageLocalSsdConfig.Companion.toKotlin(args0)
})
}).orElse(null),
fastSocket = javaType.fastSocket().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigFastSocket.Companion.toKotlin(args0)
})
}).orElse(null),
gcfsConfig = javaType.gcfsConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigGcfsConfig.Companion.toKotlin(args0)
})
}).orElse(null),
guestAccelerators = javaType.guestAccelerators().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigGuestAccelerator.Companion.toKotlin(args0)
})
}),
gvnic = javaType.gvnic().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigGvnic.Companion.toKotlin(args0)
})
}).orElse(null),
hostMaintenancePolicy = javaType.hostMaintenancePolicy().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigHostMaintenancePolicy.Companion.toKotlin(args0)
})
}).orElse(null),
imageType = javaType.imageType().map({ args0 -> args0 }).orElse(null),
kubeletConfig = javaType.kubeletConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigKubeletConfig.Companion.toKotlin(args0)
})
}).orElse(null),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
linuxNodeConfig = javaType.linuxNodeConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigLinuxNodeConfig.Companion.toKotlin(args0)
})
}).orElse(null),
localNvmeSsdBlockConfig = javaType.localNvmeSsdBlockConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigLocalNvmeSsdBlockConfig.Companion.toKotlin(args0)
})
}).orElse(null),
localSsdCount = javaType.localSsdCount().map({ args0 -> args0 }).orElse(null),
loggingVariant = javaType.loggingVariant().map({ args0 -> args0 }).orElse(null),
machineType = javaType.machineType().map({ args0 -> args0 }).orElse(null),
metadata = javaType.metadata().map({ args0 -> args0.key.to(args0.value) }).toMap(),
minCpuPlatform = javaType.minCpuPlatform().map({ args0 -> args0 }).orElse(null),
nodeGroup = javaType.nodeGroup().map({ args0 -> args0 }).orElse(null),
oauthScopes = javaType.oauthScopes().map({ args0 -> args0 }),
preemptible = javaType.preemptible().map({ args0 -> args0 }).orElse(null),
reservationAffinity = javaType.reservationAffinity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigReservationAffinity.Companion.toKotlin(args0)
})
}).orElse(null),
resourceLabels = javaType.resourceLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
resourceManagerTags = javaType.resourceManagerTags().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
sandboxConfig = javaType.sandboxConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigSandboxConfig.Companion.toKotlin(args0)
})
}).orElse(null),
secondaryBootDisks = javaType.secondaryBootDisks().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigSecondaryBootDisk.Companion.toKotlin(args0)
})
}),
serviceAccount = javaType.serviceAccount().map({ args0 -> args0 }).orElse(null),
shieldedInstanceConfig = javaType.shieldedInstanceConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigShieldedInstanceConfig.Companion.toKotlin(args0)
})
}).orElse(null),
soleTenantConfig = javaType.soleTenantConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigSoleTenantConfig.Companion.toKotlin(args0)
})
}).orElse(null),
spot = javaType.spot().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 -> args0 }),
taints = javaType.taints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigTaint.Companion.toKotlin(args0)
})
}),
workloadMetadataConfig = javaType.workloadMetadataConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfigWorkloadMetadataConfig.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy