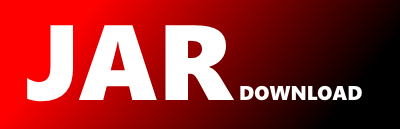
com.pulumi.gcp.databasemigrationservice.kotlin.ConnectionProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.databasemigrationservice.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.databasemigrationservice.ConnectionProfileArgs.builder
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileAlloydbArgs
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileAlloydbArgsBuilder
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileCloudsqlArgs
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileCloudsqlArgsBuilder
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileMysqlArgs
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileMysqlArgsBuilder
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileOracleArgs
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileOracleArgsBuilder
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfilePostgresqlArgs
import com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfilePostgresqlArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A connection profile definition.
* To get more information about ConnectionProfile, see:
* * [API documentation](https://cloud.google.com/database-migration/docs/reference/rest/v1/projects.locations.connectionProfiles/create)
* * How-to Guides
* * [Database Migration](https://cloud.google.com/database-migration/docs/)
* ## Example Usage
* ### Database Migration Service Connection Profile Cloudsql
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const project = gcp.organizations.getProject({});
* const cloudsqldb = new gcp.sql.DatabaseInstance("cloudsqldb", {
* name: "my-database",
* databaseVersion: "MYSQL_5_7",
* settings: {
* tier: "db-n1-standard-1",
* deletionProtectionEnabled: false,
* },
* deletionProtection: false,
* });
* const sqlClientCert = new gcp.sql.SslCert("sql_client_cert", {
* commonName: "my-cert",
* instance: cloudsqldb.name,
* });
* const sqldbUser = new gcp.sql.User("sqldb_user", {
* name: "my-username",
* instance: cloudsqldb.name,
* password: "my-password",
* });
* const cloudsqlprofile = new gcp.databasemigrationservice.ConnectionProfile("cloudsqlprofile", {
* location: "us-central1",
* connectionProfileId: "my-fromprofileid",
* displayName: "my-fromprofileid_display",
* labels: {
* foo: "bar",
* },
* mysql: {
* host: cloudsqldb.ipAddresses.apply(ipAddresses => ipAddresses[0].ipAddress),
* port: 3306,
* username: sqldbUser.name,
* password: sqldbUser.password,
* ssl: {
* clientKey: sqlClientCert.privateKey,
* clientCertificate: sqlClientCert.cert,
* caCertificate: sqlClientCert.serverCaCert,
* },
* cloudSqlId: "my-database",
* },
* });
* const cloudsqlprofileDestination = new gcp.databasemigrationservice.ConnectionProfile("cloudsqlprofile_destination", {
* location: "us-central1",
* connectionProfileId: "my-toprofileid",
* displayName: "my-toprofileid_displayname",
* labels: {
* foo: "bar",
* },
* cloudsql: {
* settings: {
* databaseVersion: "MYSQL_5_7",
* userLabels: {
* cloudfoo: "cloudbar",
* },
* tier: "db-n1-standard-1",
* edition: "ENTERPRISE",
* storageAutoResizeLimit: "0",
* activationPolicy: "ALWAYS",
* ipConfig: {
* enableIpv4: true,
* requireSsl: true,
* },
* autoStorageIncrease: true,
* dataDiskType: "PD_HDD",
* dataDiskSizeGb: "11",
* zone: "us-central1-b",
* sourceId: project.then(project => `projects/${project.projectId}/locations/us-central1/connectionProfiles/my-fromprofileid`),
* rootPassword: "testpasscloudsql",
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project = gcp.organizations.get_project()
* cloudsqldb = gcp.sql.DatabaseInstance("cloudsqldb",
* name="my-database",
* database_version="MYSQL_5_7",
* settings=gcp.sql.DatabaseInstanceSettingsArgs(
* tier="db-n1-standard-1",
* deletion_protection_enabled=False,
* ),
* deletion_protection=False)
* sql_client_cert = gcp.sql.SslCert("sql_client_cert",
* common_name="my-cert",
* instance=cloudsqldb.name)
* sqldb_user = gcp.sql.User("sqldb_user",
* name="my-username",
* instance=cloudsqldb.name,
* password="my-password")
* cloudsqlprofile = gcp.databasemigrationservice.ConnectionProfile("cloudsqlprofile",
* location="us-central1",
* connection_profile_id="my-fromprofileid",
* display_name="my-fromprofileid_display",
* labels={
* "foo": "bar",
* },
* mysql=gcp.databasemigrationservice.ConnectionProfileMysqlArgs(
* host=cloudsqldb.ip_addresses[0].ip_address,
* port=3306,
* username=sqldb_user.name,
* password=sqldb_user.password,
* ssl=gcp.databasemigrationservice.ConnectionProfileMysqlSslArgs(
* client_key=sql_client_cert.private_key,
* client_certificate=sql_client_cert.cert,
* ca_certificate=sql_client_cert.server_ca_cert,
* ),
* cloud_sql_id="my-database",
* ))
* cloudsqlprofile_destination = gcp.databasemigrationservice.ConnectionProfile("cloudsqlprofile_destination",
* location="us-central1",
* connection_profile_id="my-toprofileid",
* display_name="my-toprofileid_displayname",
* labels={
* "foo": "bar",
* },
* cloudsql=gcp.databasemigrationservice.ConnectionProfileCloudsqlArgs(
* settings=gcp.databasemigrationservice.ConnectionProfileCloudsqlSettingsArgs(
* database_version="MYSQL_5_7",
* user_labels={
* "cloudfoo": "cloudbar",
* },
* tier="db-n1-standard-1",
* edition="ENTERPRISE",
* storage_auto_resize_limit="0",
* activation_policy="ALWAYS",
* ip_config=gcp.databasemigrationservice.ConnectionProfileCloudsqlSettingsIpConfigArgs(
* enable_ipv4=True,
* require_ssl=True,
* ),
* auto_storage_increase=True,
* data_disk_type="PD_HDD",
* data_disk_size_gb="11",
* zone="us-central1-b",
* source_id=f"projects/{project.project_id}/locations/us-central1/connectionProfiles/my-fromprofileid",
* root_password="testpasscloudsql",
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var project = Gcp.Organizations.GetProject.Invoke();
* var cloudsqldb = new Gcp.Sql.DatabaseInstance("cloudsqldb", new()
* {
* Name = "my-database",
* DatabaseVersion = "MYSQL_5_7",
* Settings = new Gcp.Sql.Inputs.DatabaseInstanceSettingsArgs
* {
* Tier = "db-n1-standard-1",
* DeletionProtectionEnabled = false,
* },
* DeletionProtection = false,
* });
* var sqlClientCert = new Gcp.Sql.SslCert("sql_client_cert", new()
* {
* CommonName = "my-cert",
* Instance = cloudsqldb.Name,
* });
* var sqldbUser = new Gcp.Sql.User("sqldb_user", new()
* {
* Name = "my-username",
* Instance = cloudsqldb.Name,
* Password = "my-password",
* });
* var cloudsqlprofile = new Gcp.DatabaseMigrationService.ConnectionProfile("cloudsqlprofile", new()
* {
* Location = "us-central1",
* ConnectionProfileId = "my-fromprofileid",
* DisplayName = "my-fromprofileid_display",
* Labels =
* {
* { "foo", "bar" },
* },
* Mysql = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileMysqlArgs
* {
* Host = cloudsqldb.IpAddresses.Apply(ipAddresses => ipAddresses[0].IpAddress),
* Port = 3306,
* Username = sqldbUser.Name,
* Password = sqldbUser.Password,
* Ssl = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileMysqlSslArgs
* {
* ClientKey = sqlClientCert.PrivateKey,
* ClientCertificate = sqlClientCert.Cert,
* CaCertificate = sqlClientCert.ServerCaCert,
* },
* CloudSqlId = "my-database",
* },
* });
* var cloudsqlprofileDestination = new Gcp.DatabaseMigrationService.ConnectionProfile("cloudsqlprofile_destination", new()
* {
* Location = "us-central1",
* ConnectionProfileId = "my-toprofileid",
* DisplayName = "my-toprofileid_displayname",
* Labels =
* {
* { "foo", "bar" },
* },
* Cloudsql = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileCloudsqlArgs
* {
* Settings = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileCloudsqlSettingsArgs
* {
* DatabaseVersion = "MYSQL_5_7",
* UserLabels =
* {
* { "cloudfoo", "cloudbar" },
* },
* Tier = "db-n1-standard-1",
* Edition = "ENTERPRISE",
* StorageAutoResizeLimit = "0",
* ActivationPolicy = "ALWAYS",
* IpConfig = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileCloudsqlSettingsIpConfigArgs
* {
* EnableIpv4 = true,
* RequireSsl = true,
* },
* AutoStorageIncrease = true,
* DataDiskType = "PD_HDD",
* DataDiskSizeGb = "11",
* Zone = "us-central1-b",
* SourceId = $"projects/{project.Apply(getProjectResult => getProjectResult.ProjectId)}/locations/us-central1/connectionProfiles/my-fromprofileid",
* RootPassword = "testpasscloudsql",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/databasemigrationservice"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/sql"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* cloudsqldb, err := sql.NewDatabaseInstance(ctx, "cloudsqldb", &sql.DatabaseInstanceArgs{
* Name: pulumi.String("my-database"),
* DatabaseVersion: pulumi.String("MYSQL_5_7"),
* Settings: &sql.DatabaseInstanceSettingsArgs{
* Tier: pulumi.String("db-n1-standard-1"),
* DeletionProtectionEnabled: pulumi.Bool(false),
* },
* DeletionProtection: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* sqlClientCert, err := sql.NewSslCert(ctx, "sql_client_cert", &sql.SslCertArgs{
* CommonName: pulumi.String("my-cert"),
* Instance: cloudsqldb.Name,
* })
* if err != nil {
* return err
* }
* sqldbUser, err := sql.NewUser(ctx, "sqldb_user", &sql.UserArgs{
* Name: pulumi.String("my-username"),
* Instance: cloudsqldb.Name,
* Password: pulumi.String("my-password"),
* })
* if err != nil {
* return err
* }
* _, err = databasemigrationservice.NewConnectionProfile(ctx, "cloudsqlprofile", &databasemigrationservice.ConnectionProfileArgs{
* Location: pulumi.String("us-central1"),
* ConnectionProfileId: pulumi.String("my-fromprofileid"),
* DisplayName: pulumi.String("my-fromprofileid_display"),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Mysql: &databasemigrationservice.ConnectionProfileMysqlArgs{
* Host: cloudsqldb.IpAddresses.ApplyT(func(ipAddresses []sql.DatabaseInstanceIpAddress) (*string, error) {
* return &ipAddresses[0].IpAddress, nil
* }).(pulumi.StringPtrOutput),
* Port: pulumi.Int(3306),
* Username: sqldbUser.Name,
* Password: sqldbUser.Password,
* Ssl: &databasemigrationservice.ConnectionProfileMysqlSslArgs{
* ClientKey: sqlClientCert.PrivateKey,
* ClientCertificate: sqlClientCert.Cert,
* CaCertificate: sqlClientCert.ServerCaCert,
* },
* CloudSqlId: pulumi.String("my-database"),
* },
* })
* if err != nil {
* return err
* }
* _, err = databasemigrationservice.NewConnectionProfile(ctx, "cloudsqlprofile_destination", &databasemigrationservice.ConnectionProfileArgs{
* Location: pulumi.String("us-central1"),
* ConnectionProfileId: pulumi.String("my-toprofileid"),
* DisplayName: pulumi.String("my-toprofileid_displayname"),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Cloudsql: &databasemigrationservice.ConnectionProfileCloudsqlArgs{
* Settings: &databasemigrationservice.ConnectionProfileCloudsqlSettingsArgs{
* DatabaseVersion: pulumi.String("MYSQL_5_7"),
* UserLabels: pulumi.StringMap{
* "cloudfoo": pulumi.String("cloudbar"),
* },
* Tier: pulumi.String("db-n1-standard-1"),
* Edition: pulumi.String("ENTERPRISE"),
* StorageAutoResizeLimit: pulumi.String("0"),
* ActivationPolicy: pulumi.String("ALWAYS"),
* IpConfig: &databasemigrationservice.ConnectionProfileCloudsqlSettingsIpConfigArgs{
* EnableIpv4: pulumi.Bool(true),
* RequireSsl: pulumi.Bool(true),
* },
* AutoStorageIncrease: pulumi.Bool(true),
* DataDiskType: pulumi.String("PD_HDD"),
* DataDiskSizeGb: pulumi.String("11"),
* Zone: pulumi.String("us-central1-b"),
* SourceId: pulumi.String(fmt.Sprintf("projects/%v/locations/us-central1/connectionProfiles/my-fromprofileid", project.ProjectId)),
* RootPassword: pulumi.String("testpasscloudsql"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.sql.DatabaseInstance;
* import com.pulumi.gcp.sql.DatabaseInstanceArgs;
* import com.pulumi.gcp.sql.inputs.DatabaseInstanceSettingsArgs;
* import com.pulumi.gcp.sql.SslCert;
* import com.pulumi.gcp.sql.SslCertArgs;
* import com.pulumi.gcp.sql.User;
* import com.pulumi.gcp.sql.UserArgs;
* import com.pulumi.gcp.databasemigrationservice.ConnectionProfile;
* import com.pulumi.gcp.databasemigrationservice.ConnectionProfileArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileMysqlArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileMysqlSslArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileCloudsqlArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileCloudsqlSettingsArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileCloudsqlSettingsIpConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var project = OrganizationsFunctions.getProject();
* var cloudsqldb = new DatabaseInstance("cloudsqldb", DatabaseInstanceArgs.builder()
* .name("my-database")
* .databaseVersion("MYSQL_5_7")
* .settings(DatabaseInstanceSettingsArgs.builder()
* .tier("db-n1-standard-1")
* .deletionProtectionEnabled(false)
* .build())
* .deletionProtection(false)
* .build());
* var sqlClientCert = new SslCert("sqlClientCert", SslCertArgs.builder()
* .commonName("my-cert")
* .instance(cloudsqldb.name())
* .build());
* var sqldbUser = new User("sqldbUser", UserArgs.builder()
* .name("my-username")
* .instance(cloudsqldb.name())
* .password("my-password")
* .build());
* var cloudsqlprofile = new ConnectionProfile("cloudsqlprofile", ConnectionProfileArgs.builder()
* .location("us-central1")
* .connectionProfileId("my-fromprofileid")
* .displayName("my-fromprofileid_display")
* .labels(Map.of("foo", "bar"))
* .mysql(ConnectionProfileMysqlArgs.builder()
* .host(cloudsqldb.ipAddresses().applyValue(ipAddresses -> ipAddresses[0].ipAddress()))
* .port(3306)
* .username(sqldbUser.name())
* .password(sqldbUser.password())
* .ssl(ConnectionProfileMysqlSslArgs.builder()
* .clientKey(sqlClientCert.privateKey())
* .clientCertificate(sqlClientCert.cert())
* .caCertificate(sqlClientCert.serverCaCert())
* .build())
* .cloudSqlId("my-database")
* .build())
* .build());
* var cloudsqlprofileDestination = new ConnectionProfile("cloudsqlprofileDestination", ConnectionProfileArgs.builder()
* .location("us-central1")
* .connectionProfileId("my-toprofileid")
* .displayName("my-toprofileid_displayname")
* .labels(Map.of("foo", "bar"))
* .cloudsql(ConnectionProfileCloudsqlArgs.builder()
* .settings(ConnectionProfileCloudsqlSettingsArgs.builder()
* .databaseVersion("MYSQL_5_7")
* .userLabels(Map.of("cloudfoo", "cloudbar"))
* .tier("db-n1-standard-1")
* .edition("ENTERPRISE")
* .storageAutoResizeLimit("0")
* .activationPolicy("ALWAYS")
* .ipConfig(ConnectionProfileCloudsqlSettingsIpConfigArgs.builder()
* .enableIpv4(true)
* .requireSsl(true)
* .build())
* .autoStorageIncrease(true)
* .dataDiskType("PD_HDD")
* .dataDiskSizeGb("11")
* .zone("us-central1-b")
* .sourceId(String.format("projects/%s/locations/us-central1/connectionProfiles/my-fromprofileid", project.applyValue(getProjectResult -> getProjectResult.projectId())))
* .rootPassword("testpasscloudsql")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* cloudsqldb:
* type: gcp:sql:DatabaseInstance
* properties:
* name: my-database
* databaseVersion: MYSQL_5_7
* settings:
* tier: db-n1-standard-1
* deletionProtectionEnabled: false
* deletionProtection: false
* sqlClientCert:
* type: gcp:sql:SslCert
* name: sql_client_cert
* properties:
* commonName: my-cert
* instance: ${cloudsqldb.name}
* sqldbUser:
* type: gcp:sql:User
* name: sqldb_user
* properties:
* name: my-username
* instance: ${cloudsqldb.name}
* password: my-password
* cloudsqlprofile:
* type: gcp:databasemigrationservice:ConnectionProfile
* properties:
* location: us-central1
* connectionProfileId: my-fromprofileid
* displayName: my-fromprofileid_display
* labels:
* foo: bar
* mysql:
* host: ${cloudsqldb.ipAddresses[0].ipAddress}
* port: 3306
* username: ${sqldbUser.name}
* password: ${sqldbUser.password}
* ssl:
* clientKey: ${sqlClientCert.privateKey}
* clientCertificate: ${sqlClientCert.cert}
* caCertificate: ${sqlClientCert.serverCaCert}
* cloudSqlId: my-database
* cloudsqlprofileDestination:
* type: gcp:databasemigrationservice:ConnectionProfile
* name: cloudsqlprofile_destination
* properties:
* location: us-central1
* connectionProfileId: my-toprofileid
* displayName: my-toprofileid_displayname
* labels:
* foo: bar
* cloudsql:
* settings:
* databaseVersion: MYSQL_5_7
* userLabels:
* cloudfoo: cloudbar
* tier: db-n1-standard-1
* edition: ENTERPRISE
* storageAutoResizeLimit: '0'
* activationPolicy: ALWAYS
* ipConfig:
* enableIpv4: true
* requireSsl: true
* autoStorageIncrease: true
* dataDiskType: PD_HDD
* dataDiskSizeGb: '11'
* zone: us-central1-b
* sourceId: projects/${project.projectId}/locations/us-central1/connectionProfiles/my-fromprofileid
* rootPassword: testpasscloudsql
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Database Migration Service Connection Profile Postgres
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const postgresqldb = new gcp.sql.DatabaseInstance("postgresqldb", {
* name: "my-database",
* databaseVersion: "POSTGRES_12",
* settings: {
* tier: "db-custom-2-13312",
* },
* deletionProtection: false,
* });
* const sqlClientCert = new gcp.sql.SslCert("sql_client_cert", {
* commonName: "my-cert",
* instance: postgresqldb.name,
* });
* const sqldbUser = new gcp.sql.User("sqldb_user", {
* name: "my-username",
* instance: postgresqldb.name,
* password: "my-password",
* });
* const postgresprofile = new gcp.databasemigrationservice.ConnectionProfile("postgresprofile", {
* location: "us-central1",
* connectionProfileId: "my-profileid",
* displayName: "my-profileid_display",
* labels: {
* foo: "bar",
* },
* postgresql: {
* host: postgresqldb.ipAddresses.apply(ipAddresses => ipAddresses[0].ipAddress),
* port: 5432,
* username: sqldbUser.name,
* password: sqldbUser.password,
* ssl: {
* clientKey: sqlClientCert.privateKey,
* clientCertificate: sqlClientCert.cert,
* caCertificate: sqlClientCert.serverCaCert,
* },
* cloudSqlId: "my-database",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* postgresqldb = gcp.sql.DatabaseInstance("postgresqldb",
* name="my-database",
* database_version="POSTGRES_12",
* settings=gcp.sql.DatabaseInstanceSettingsArgs(
* tier="db-custom-2-13312",
* ),
* deletion_protection=False)
* sql_client_cert = gcp.sql.SslCert("sql_client_cert",
* common_name="my-cert",
* instance=postgresqldb.name)
* sqldb_user = gcp.sql.User("sqldb_user",
* name="my-username",
* instance=postgresqldb.name,
* password="my-password")
* postgresprofile = gcp.databasemigrationservice.ConnectionProfile("postgresprofile",
* location="us-central1",
* connection_profile_id="my-profileid",
* display_name="my-profileid_display",
* labels={
* "foo": "bar",
* },
* postgresql=gcp.databasemigrationservice.ConnectionProfilePostgresqlArgs(
* host=postgresqldb.ip_addresses[0].ip_address,
* port=5432,
* username=sqldb_user.name,
* password=sqldb_user.password,
* ssl=gcp.databasemigrationservice.ConnectionProfilePostgresqlSslArgs(
* client_key=sql_client_cert.private_key,
* client_certificate=sql_client_cert.cert,
* ca_certificate=sql_client_cert.server_ca_cert,
* ),
* cloud_sql_id="my-database",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var postgresqldb = new Gcp.Sql.DatabaseInstance("postgresqldb", new()
* {
* Name = "my-database",
* DatabaseVersion = "POSTGRES_12",
* Settings = new Gcp.Sql.Inputs.DatabaseInstanceSettingsArgs
* {
* Tier = "db-custom-2-13312",
* },
* DeletionProtection = false,
* });
* var sqlClientCert = new Gcp.Sql.SslCert("sql_client_cert", new()
* {
* CommonName = "my-cert",
* Instance = postgresqldb.Name,
* });
* var sqldbUser = new Gcp.Sql.User("sqldb_user", new()
* {
* Name = "my-username",
* Instance = postgresqldb.Name,
* Password = "my-password",
* });
* var postgresprofile = new Gcp.DatabaseMigrationService.ConnectionProfile("postgresprofile", new()
* {
* Location = "us-central1",
* ConnectionProfileId = "my-profileid",
* DisplayName = "my-profileid_display",
* Labels =
* {
* { "foo", "bar" },
* },
* Postgresql = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfilePostgresqlArgs
* {
* Host = postgresqldb.IpAddresses.Apply(ipAddresses => ipAddresses[0].IpAddress),
* Port = 5432,
* Username = sqldbUser.Name,
* Password = sqldbUser.Password,
* Ssl = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfilePostgresqlSslArgs
* {
* ClientKey = sqlClientCert.PrivateKey,
* ClientCertificate = sqlClientCert.Cert,
* CaCertificate = sqlClientCert.ServerCaCert,
* },
* CloudSqlId = "my-database",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/databasemigrationservice"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/sql"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* postgresqldb, err := sql.NewDatabaseInstance(ctx, "postgresqldb", &sql.DatabaseInstanceArgs{
* Name: pulumi.String("my-database"),
* DatabaseVersion: pulumi.String("POSTGRES_12"),
* Settings: &sql.DatabaseInstanceSettingsArgs{
* Tier: pulumi.String("db-custom-2-13312"),
* },
* DeletionProtection: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* sqlClientCert, err := sql.NewSslCert(ctx, "sql_client_cert", &sql.SslCertArgs{
* CommonName: pulumi.String("my-cert"),
* Instance: postgresqldb.Name,
* })
* if err != nil {
* return err
* }
* sqldbUser, err := sql.NewUser(ctx, "sqldb_user", &sql.UserArgs{
* Name: pulumi.String("my-username"),
* Instance: postgresqldb.Name,
* Password: pulumi.String("my-password"),
* })
* if err != nil {
* return err
* }
* _, err = databasemigrationservice.NewConnectionProfile(ctx, "postgresprofile", &databasemigrationservice.ConnectionProfileArgs{
* Location: pulumi.String("us-central1"),
* ConnectionProfileId: pulumi.String("my-profileid"),
* DisplayName: pulumi.String("my-profileid_display"),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Postgresql: &databasemigrationservice.ConnectionProfilePostgresqlArgs{
* Host: postgresqldb.IpAddresses.ApplyT(func(ipAddresses []sql.DatabaseInstanceIpAddress) (*string, error) {
* return &ipAddresses[0].IpAddress, nil
* }).(pulumi.StringPtrOutput),
* Port: pulumi.Int(5432),
* Username: sqldbUser.Name,
* Password: sqldbUser.Password,
* Ssl: &databasemigrationservice.ConnectionProfilePostgresqlSslArgs{
* ClientKey: sqlClientCert.PrivateKey,
* ClientCertificate: sqlClientCert.Cert,
* CaCertificate: sqlClientCert.ServerCaCert,
* },
* CloudSqlId: pulumi.String("my-database"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.sql.DatabaseInstance;
* import com.pulumi.gcp.sql.DatabaseInstanceArgs;
* import com.pulumi.gcp.sql.inputs.DatabaseInstanceSettingsArgs;
* import com.pulumi.gcp.sql.SslCert;
* import com.pulumi.gcp.sql.SslCertArgs;
* import com.pulumi.gcp.sql.User;
* import com.pulumi.gcp.sql.UserArgs;
* import com.pulumi.gcp.databasemigrationservice.ConnectionProfile;
* import com.pulumi.gcp.databasemigrationservice.ConnectionProfileArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfilePostgresqlArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfilePostgresqlSslArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var postgresqldb = new DatabaseInstance("postgresqldb", DatabaseInstanceArgs.builder()
* .name("my-database")
* .databaseVersion("POSTGRES_12")
* .settings(DatabaseInstanceSettingsArgs.builder()
* .tier("db-custom-2-13312")
* .build())
* .deletionProtection(false)
* .build());
* var sqlClientCert = new SslCert("sqlClientCert", SslCertArgs.builder()
* .commonName("my-cert")
* .instance(postgresqldb.name())
* .build());
* var sqldbUser = new User("sqldbUser", UserArgs.builder()
* .name("my-username")
* .instance(postgresqldb.name())
* .password("my-password")
* .build());
* var postgresprofile = new ConnectionProfile("postgresprofile", ConnectionProfileArgs.builder()
* .location("us-central1")
* .connectionProfileId("my-profileid")
* .displayName("my-profileid_display")
* .labels(Map.of("foo", "bar"))
* .postgresql(ConnectionProfilePostgresqlArgs.builder()
* .host(postgresqldb.ipAddresses().applyValue(ipAddresses -> ipAddresses[0].ipAddress()))
* .port(5432)
* .username(sqldbUser.name())
* .password(sqldbUser.password())
* .ssl(ConnectionProfilePostgresqlSslArgs.builder()
* .clientKey(sqlClientCert.privateKey())
* .clientCertificate(sqlClientCert.cert())
* .caCertificate(sqlClientCert.serverCaCert())
* .build())
* .cloudSqlId("my-database")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* postgresqldb:
* type: gcp:sql:DatabaseInstance
* properties:
* name: my-database
* databaseVersion: POSTGRES_12
* settings:
* tier: db-custom-2-13312
* deletionProtection: false
* sqlClientCert:
* type: gcp:sql:SslCert
* name: sql_client_cert
* properties:
* commonName: my-cert
* instance: ${postgresqldb.name}
* sqldbUser:
* type: gcp:sql:User
* name: sqldb_user
* properties:
* name: my-username
* instance: ${postgresqldb.name}
* password: my-password
* postgresprofile:
* type: gcp:databasemigrationservice:ConnectionProfile
* properties:
* location: us-central1
* connectionProfileId: my-profileid
* displayName: my-profileid_display
* labels:
* foo: bar
* postgresql:
* host: ${postgresqldb.ipAddresses[0].ipAddress}
* port: 5432
* username: ${sqldbUser.name}
* password: ${sqldbUser.password}
* ssl:
* clientKey: ${sqlClientCert.privateKey}
* clientCertificate: ${sqlClientCert.cert}
* caCertificate: ${sqlClientCert.serverCaCert}
* cloudSqlId: my-database
* ```
*
* ### Database Migration Service Connection Profile Oracle
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const oracleprofile = new gcp.databasemigrationservice.ConnectionProfile("oracleprofile", {
* location: "us-central1",
* connectionProfileId: "my-profileid",
* displayName: "my-profileid_display",
* labels: {
* foo: "bar",
* },
* oracle: {
* host: "host",
* port: 1521,
* username: "username",
* password: "password",
* databaseService: "dbprovider",
* staticServiceIpConnectivity: {},
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* oracleprofile = gcp.databasemigrationservice.ConnectionProfile("oracleprofile",
* location="us-central1",
* connection_profile_id="my-profileid",
* display_name="my-profileid_display",
* labels={
* "foo": "bar",
* },
* oracle=gcp.databasemigrationservice.ConnectionProfileOracleArgs(
* host="host",
* port=1521,
* username="username",
* password="password",
* database_service="dbprovider",
* static_service_ip_connectivity=gcp.databasemigrationservice.ConnectionProfileOracleStaticServiceIpConnectivityArgs(),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var oracleprofile = new Gcp.DatabaseMigrationService.ConnectionProfile("oracleprofile", new()
* {
* Location = "us-central1",
* ConnectionProfileId = "my-profileid",
* DisplayName = "my-profileid_display",
* Labels =
* {
* { "foo", "bar" },
* },
* Oracle = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileOracleArgs
* {
* Host = "host",
* Port = 1521,
* Username = "username",
* Password = "password",
* DatabaseService = "dbprovider",
* StaticServiceIpConnectivity = null,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/databasemigrationservice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := databasemigrationservice.NewConnectionProfile(ctx, "oracleprofile", &databasemigrationservice.ConnectionProfileArgs{
* Location: pulumi.String("us-central1"),
* ConnectionProfileId: pulumi.String("my-profileid"),
* DisplayName: pulumi.String("my-profileid_display"),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Oracle: &databasemigrationservice.ConnectionProfileOracleArgs{
* Host: pulumi.String("host"),
* Port: pulumi.Int(1521),
* Username: pulumi.String("username"),
* Password: pulumi.String("password"),
* DatabaseService: pulumi.String("dbprovider"),
* StaticServiceIpConnectivity: nil,
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.databasemigrationservice.ConnectionProfile;
* import com.pulumi.gcp.databasemigrationservice.ConnectionProfileArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileOracleArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileOracleStaticServiceIpConnectivityArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var oracleprofile = new ConnectionProfile("oracleprofile", ConnectionProfileArgs.builder()
* .location("us-central1")
* .connectionProfileId("my-profileid")
* .displayName("my-profileid_display")
* .labels(Map.of("foo", "bar"))
* .oracle(ConnectionProfileOracleArgs.builder()
* .host("host")
* .port(1521)
* .username("username")
* .password("password")
* .databaseService("dbprovider")
* .staticServiceIpConnectivity()
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* oracleprofile:
* type: gcp:databasemigrationservice:ConnectionProfile
* properties:
* location: us-central1
* connectionProfileId: my-profileid
* displayName: my-profileid_display
* labels:
* foo: bar
* oracle:
* host: host
* port: 1521
* username: username
* password: password
* databaseService: dbprovider
* staticServiceIpConnectivity: {}
* ```
*
* ### Database Migration Service Connection Profile Alloydb
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const project = gcp.organizations.getProject({});
* const _default = new gcp.compute.Network("default", {name: "vpc-network"});
* const privateIpAlloc = new gcp.compute.GlobalAddress("private_ip_alloc", {
* name: "private-ip-alloc",
* addressType: "INTERNAL",
* purpose: "VPC_PEERING",
* prefixLength: 16,
* network: _default.id,
* });
* const vpcConnection = new gcp.servicenetworking.Connection("vpc_connection", {
* network: _default.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [privateIpAlloc.name],
* });
* const alloydbprofile = new gcp.databasemigrationservice.ConnectionProfile("alloydbprofile", {
* location: "us-central1",
* connectionProfileId: "my-profileid",
* displayName: "my-profileid_display",
* labels: {
* foo: "bar",
* },
* alloydb: {
* clusterId: "tf-test-dbmsalloycluster_52865",
* settings: {
* initialUser: {
* user: "alloyuser_85840",
* password: "alloypass_60302",
* },
* vpcNetwork: _default.id,
* labels: {
* alloyfoo: "alloybar",
* },
* primaryInstanceSettings: {
* id: "priminstid",
* machineConfig: {
* cpuCount: 2,
* },
* databaseFlags: {},
* labels: {
* alloysinstfoo: "allowinstbar",
* },
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project = gcp.organizations.get_project()
* default = gcp.compute.Network("default", name="vpc-network")
* private_ip_alloc = gcp.compute.GlobalAddress("private_ip_alloc",
* name="private-ip-alloc",
* address_type="INTERNAL",
* purpose="VPC_PEERING",
* prefix_length=16,
* network=default.id)
* vpc_connection = gcp.servicenetworking.Connection("vpc_connection",
* network=default.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[private_ip_alloc.name])
* alloydbprofile = gcp.databasemigrationservice.ConnectionProfile("alloydbprofile",
* location="us-central1",
* connection_profile_id="my-profileid",
* display_name="my-profileid_display",
* labels={
* "foo": "bar",
* },
* alloydb=gcp.databasemigrationservice.ConnectionProfileAlloydbArgs(
* cluster_id="tf-test-dbmsalloycluster_52865",
* settings=gcp.databasemigrationservice.ConnectionProfileAlloydbSettingsArgs(
* initial_user=gcp.databasemigrationservice.ConnectionProfileAlloydbSettingsInitialUserArgs(
* user="alloyuser_85840",
* password="alloypass_60302",
* ),
* vpc_network=default.id,
* labels={
* "alloyfoo": "alloybar",
* },
* primary_instance_settings=gcp.databasemigrationservice.ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsArgs(
* id="priminstid",
* machine_config=gcp.databasemigrationservice.ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsMachineConfigArgs(
* cpu_count=2,
* ),
* database_flags={},
* labels={
* "alloysinstfoo": "allowinstbar",
* },
* ),
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var project = Gcp.Organizations.GetProject.Invoke();
* var @default = new Gcp.Compute.Network("default", new()
* {
* Name = "vpc-network",
* });
* var privateIpAlloc = new Gcp.Compute.GlobalAddress("private_ip_alloc", new()
* {
* Name = "private-ip-alloc",
* AddressType = "INTERNAL",
* Purpose = "VPC_PEERING",
* PrefixLength = 16,
* Network = @default.Id,
* });
* var vpcConnection = new Gcp.ServiceNetworking.Connection("vpc_connection", new()
* {
* Network = @default.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* privateIpAlloc.Name,
* },
* });
* var alloydbprofile = new Gcp.DatabaseMigrationService.ConnectionProfile("alloydbprofile", new()
* {
* Location = "us-central1",
* ConnectionProfileId = "my-profileid",
* DisplayName = "my-profileid_display",
* Labels =
* {
* { "foo", "bar" },
* },
* Alloydb = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileAlloydbArgs
* {
* ClusterId = "tf-test-dbmsalloycluster_52865",
* Settings = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileAlloydbSettingsArgs
* {
* InitialUser = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileAlloydbSettingsInitialUserArgs
* {
* User = "alloyuser_85840",
* Password = "alloypass_60302",
* },
* VpcNetwork = @default.Id,
* Labels =
* {
* { "alloyfoo", "alloybar" },
* },
* PrimaryInstanceSettings = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsArgs
* {
* Id = "priminstid",
* MachineConfig = new Gcp.DatabaseMigrationService.Inputs.ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsMachineConfigArgs
* {
* CpuCount = 2,
* },
* DatabaseFlags = null,
* Labels =
* {
* { "alloysinstfoo", "allowinstbar" },
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/databasemigrationservice"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("vpc-network"),
* })
* if err != nil {
* return err
* }
* privateIpAlloc, err := compute.NewGlobalAddress(ctx, "private_ip_alloc", &compute.GlobalAddressArgs{
* Name: pulumi.String("private-ip-alloc"),
* AddressType: pulumi.String("INTERNAL"),
* Purpose: pulumi.String("VPC_PEERING"),
* PrefixLength: pulumi.Int(16),
* Network: _default.ID(),
* })
* if err != nil {
* return err
* }
* _, err = servicenetworking.NewConnection(ctx, "vpc_connection", &servicenetworking.ConnectionArgs{
* Network: _default.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* privateIpAlloc.Name,
* },
* })
* if err != nil {
* return err
* }
* _, err = databasemigrationservice.NewConnectionProfile(ctx, "alloydbprofile", &databasemigrationservice.ConnectionProfileArgs{
* Location: pulumi.String("us-central1"),
* ConnectionProfileId: pulumi.String("my-profileid"),
* DisplayName: pulumi.String("my-profileid_display"),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Alloydb: &databasemigrationservice.ConnectionProfileAlloydbArgs{
* ClusterId: pulumi.String("tf-test-dbmsalloycluster_52865"),
* Settings: &databasemigrationservice.ConnectionProfileAlloydbSettingsArgs{
* InitialUser: &databasemigrationservice.ConnectionProfileAlloydbSettingsInitialUserArgs{
* User: pulumi.String("alloyuser_85840"),
* Password: pulumi.String("alloypass_60302"),
* },
* VpcNetwork: _default.ID(),
* Labels: pulumi.StringMap{
* "alloyfoo": pulumi.String("alloybar"),
* },
* PrimaryInstanceSettings: &databasemigrationservice.ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsArgs{
* Id: pulumi.String("priminstid"),
* MachineConfig: &databasemigrationservice.ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsMachineConfigArgs{
* CpuCount: pulumi.Int(2),
* },
* DatabaseFlags: nil,
* Labels: pulumi.StringMap{
* "alloysinstfoo": pulumi.String("allowinstbar"),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import com.pulumi.gcp.databasemigrationservice.ConnectionProfile;
* import com.pulumi.gcp.databasemigrationservice.ConnectionProfileArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileAlloydbArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileAlloydbSettingsArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileAlloydbSettingsInitialUserArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsArgs;
* import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsMachineConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var project = OrganizationsFunctions.getProject();
* var default_ = new Network("default", NetworkArgs.builder()
* .name("vpc-network")
* .build());
* var privateIpAlloc = new GlobalAddress("privateIpAlloc", GlobalAddressArgs.builder()
* .name("private-ip-alloc")
* .addressType("INTERNAL")
* .purpose("VPC_PEERING")
* .prefixLength(16)
* .network(default_.id())
* .build());
* var vpcConnection = new Connection("vpcConnection", ConnectionArgs.builder()
* .network(default_.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(privateIpAlloc.name())
* .build());
* var alloydbprofile = new ConnectionProfile("alloydbprofile", ConnectionProfileArgs.builder()
* .location("us-central1")
* .connectionProfileId("my-profileid")
* .displayName("my-profileid_display")
* .labels(Map.of("foo", "bar"))
* .alloydb(ConnectionProfileAlloydbArgs.builder()
* .clusterId("tf-test-dbmsalloycluster_52865")
* .settings(ConnectionProfileAlloydbSettingsArgs.builder()
* .initialUser(ConnectionProfileAlloydbSettingsInitialUserArgs.builder()
* .user("alloyuser_85840")
* .password("alloypass_60302")
* .build())
* .vpcNetwork(default_.id())
* .labels(Map.of("alloyfoo", "alloybar"))
* .primaryInstanceSettings(ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsArgs.builder()
* .id("priminstid")
* .machineConfig(ConnectionProfileAlloydbSettingsPrimaryInstanceSettingsMachineConfigArgs.builder()
* .cpuCount(2)
* .build())
* .databaseFlags()
* .labels(Map.of("alloysinstfoo", "allowinstbar"))
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:Network
* properties:
* name: vpc-network
* privateIpAlloc:
* type: gcp:compute:GlobalAddress
* name: private_ip_alloc
* properties:
* name: private-ip-alloc
* addressType: INTERNAL
* purpose: VPC_PEERING
* prefixLength: 16
* network: ${default.id}
* vpcConnection:
* type: gcp:servicenetworking:Connection
* name: vpc_connection
* properties:
* network: ${default.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${privateIpAlloc.name}
* alloydbprofile:
* type: gcp:databasemigrationservice:ConnectionProfile
* properties:
* location: us-central1
* connectionProfileId: my-profileid
* displayName: my-profileid_display
* labels:
* foo: bar
* alloydb:
* clusterId: tf-test-dbmsalloycluster_52865
* settings:
* initialUser:
* user: alloyuser_85840
* password: alloypass_60302
* vpcNetwork: ${default.id}
* labels:
* alloyfoo: alloybar
* primaryInstanceSettings:
* id: priminstid
* machineConfig:
* cpuCount: 2
* databaseFlags: {}
* labels:
* alloysinstfoo: allowinstbar
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* ConnectionProfile can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/connectionProfiles/{{connection_profile_id}}`
* * `{{project}}/{{location}}/{{connection_profile_id}}`
* * `{{location}}/{{connection_profile_id}}`
* When using the `pulumi import` command, ConnectionProfile can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:databasemigrationservice/connectionProfile:ConnectionProfile default projects/{{project}}/locations/{{location}}/connectionProfiles/{{connection_profile_id}}
* ```
* ```sh
* $ pulumi import gcp:databasemigrationservice/connectionProfile:ConnectionProfile default {{project}}/{{location}}/{{connection_profile_id}}
* ```
* ```sh
* $ pulumi import gcp:databasemigrationservice/connectionProfile:ConnectionProfile default {{location}}/{{connection_profile_id}}
* ```
* @property alloydb Specifies required connection parameters, and the parameters required to create an AlloyDB destination cluster.
* Structure is documented below.
* @property cloudsql Specifies required connection parameters, and, optionally, the parameters required to create a Cloud SQL destination database instance.
* Structure is documented below.
* @property connectionProfileId The ID of the connection profile.
* - - -
* @property displayName The connection profile display name.
* @property labels The resource labels for connection profile to use to annotate any related underlying resources such as Compute Engine VMs.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property location The location where the connection profile should reside.
* @property mysql Specifies connection parameters required specifically for MySQL databases.
* Structure is documented below.
* @property oracle Specifies connection parameters required specifically for Oracle databases.
* Structure is documented below.
* @property postgresql Specifies connection parameters required specifically for PostgreSQL databases.
* Structure is documented below.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
public data class ConnectionProfileArgs(
public val alloydb: Output? = null,
public val cloudsql: Output? = null,
public val connectionProfileId: Output? = null,
public val displayName: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy