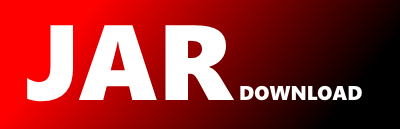
com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileAlloydbSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.databasemigrationservice.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileAlloydbSettingsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property initialUser Required. Input only. Initial user to setup during cluster creation.
* Structure is documented below.
* @property labels Labels for the AlloyDB cluster created by DMS.
* @property primaryInstanceSettings Settings for the cluster's primary instance
* Structure is documented below.
* @property vpcNetwork Required. The resource link for the VPC network in which cluster resources are created and from which they are accessible via Private IP. The network must belong to the same project as the cluster.
* It is specified in the form: 'projects/{project_number}/global/networks/{network_id}'. This is required to create a cluster.
*/
public data class ConnectionProfileAlloydbSettingsArgs(
public val initialUser: Output,
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy