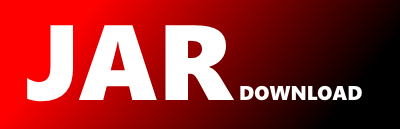
com.pulumi.gcp.databasemigrationservice.kotlin.inputs.ConnectionProfileOracleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.databasemigrationservice.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileOracleArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property databaseService Required. Database service for the Oracle connection.
* @property forwardSshConnectivity SSL configuration for the destination to connect to the source database.
* Structure is documented below.
* @property host Required. The IP or hostname of the source Oracle database.
* @property password Required. Input only. The password for the user that Database Migration Service will be using to connect to the database.
* This field is not returned on request, and the value is encrypted when stored in Database Migration Service.
* **Note**: This property is sensitive and will not be displayed in the plan.
* @property passwordSet (Output)
* Output only. Indicates If this connection profile password is stored.
* @property port Required. The network port of the source Oracle database.
* @property privateConnectivity Configuration for using a private network to communicate with the source database
* Structure is documented below.
* @property ssl SSL configuration for the destination to connect to the source database.
* Structure is documented below.
* @property staticServiceIpConnectivity This object has no nested fields.
* Static IP address connectivity configured on service project.
* @property username Required. The username that Database Migration Service will use to connect to the database. The value is encrypted when stored in Database Migration Service.
*/
public data class ConnectionProfileOracleArgs(
public val databaseService: Output,
public val forwardSshConnectivity: Output? =
null,
public val host: Output,
public val password: Output,
public val passwordSet: Output? = null,
public val port: Output,
public val privateConnectivity: Output? = null,
public val ssl: Output? = null,
public val staticServiceIpConnectivity: Output? = null,
public val username: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileOracleArgs = com.pulumi.gcp.databasemigrationservice.inputs.ConnectionProfileOracleArgs.builder()
.databaseService(databaseService.applyValue({ args0 -> args0 }))
.forwardSshConnectivity(
forwardSshConnectivity?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.host(host.applyValue({ args0 -> args0 }))
.password(password.applyValue({ args0 -> args0 }))
.passwordSet(passwordSet?.applyValue({ args0 -> args0 }))
.port(port.applyValue({ args0 -> args0 }))
.privateConnectivity(
privateConnectivity?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.ssl(ssl?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.staticServiceIpConnectivity(
staticServiceIpConnectivity?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.username(username.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConnectionProfileOracleArgs].
*/
@PulumiTagMarker
public class ConnectionProfileOracleArgsBuilder internal constructor() {
private var databaseService: Output? = null
private var forwardSshConnectivity: Output? =
null
private var host: Output? = null
private var password: Output? = null
private var passwordSet: Output? = null
private var port: Output? = null
private var privateConnectivity: Output? = null
private var ssl: Output? = null
private var staticServiceIpConnectivity:
Output? = null
private var username: Output? = null
/**
* @param value Required. Database service for the Oracle connection.
*/
@JvmName("vxuwbwwliitrftjt")
public suspend fun databaseService(`value`: Output) {
this.databaseService = value
}
/**
* @param value SSL configuration for the destination to connect to the source database.
* Structure is documented below.
*/
@JvmName("auhnhjgmvnoiwywy")
public suspend fun forwardSshConnectivity(`value`: Output) {
this.forwardSshConnectivity = value
}
/**
* @param value Required. The IP or hostname of the source Oracle database.
*/
@JvmName("bmghwnlnjgnfftbi")
public suspend fun host(`value`: Output) {
this.host = value
}
/**
* @param value Required. Input only. The password for the user that Database Migration Service will be using to connect to the database.
* This field is not returned on request, and the value is encrypted when stored in Database Migration Service.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("vhpmifbvsjukurmp")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value (Output)
* Output only. Indicates If this connection profile password is stored.
*/
@JvmName("euadlwjpibfnkwgy")
public suspend fun passwordSet(`value`: Output) {
this.passwordSet = value
}
/**
* @param value Required. The network port of the source Oracle database.
*/
@JvmName("gqfnvcvwphjdmvjg")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value Configuration for using a private network to communicate with the source database
* Structure is documented below.
*/
@JvmName("syddphrnklodeuev")
public suspend fun privateConnectivity(`value`: Output) {
this.privateConnectivity = value
}
/**
* @param value SSL configuration for the destination to connect to the source database.
* Structure is documented below.
*/
@JvmName("ikjcmdmbtvrrbkga")
public suspend fun ssl(`value`: Output) {
this.ssl = value
}
/**
* @param value This object has no nested fields.
* Static IP address connectivity configured on service project.
*/
@JvmName("ebmudqltyysjwpgg")
public suspend fun staticServiceIpConnectivity(`value`: Output) {
this.staticServiceIpConnectivity = value
}
/**
* @param value Required. The username that Database Migration Service will use to connect to the database. The value is encrypted when stored in Database Migration Service.
*/
@JvmName("qxsrvmoayjkjfcrf")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value Required. Database service for the Oracle connection.
*/
@JvmName("rdxathhggaorlkxi")
public suspend fun databaseService(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.databaseService = mapped
}
/**
* @param value SSL configuration for the destination to connect to the source database.
* Structure is documented below.
*/
@JvmName("npncsyasotijbnbp")
public suspend fun forwardSshConnectivity(`value`: ConnectionProfileOracleForwardSshConnectivityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forwardSshConnectivity = mapped
}
/**
* @param argument SSL configuration for the destination to connect to the source database.
* Structure is documented below.
*/
@JvmName("ektgrkinpyskuwja")
public suspend fun forwardSshConnectivity(argument: suspend ConnectionProfileOracleForwardSshConnectivityArgsBuilder.() -> Unit) {
val toBeMapped = ConnectionProfileOracleForwardSshConnectivityArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.forwardSshConnectivity = mapped
}
/**
* @param value Required. The IP or hostname of the source Oracle database.
*/
@JvmName("bgglwssbspwuouwd")
public suspend fun host(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.host = mapped
}
/**
* @param value Required. Input only. The password for the user that Database Migration Service will be using to connect to the database.
* This field is not returned on request, and the value is encrypted when stored in Database Migration Service.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("gnrbrwhwlnjmvyrm")
public suspend fun password(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value (Output)
* Output only. Indicates If this connection profile password is stored.
*/
@JvmName("mdcbslhplqtrmuqy")
public suspend fun passwordSet(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.passwordSet = mapped
}
/**
* @param value Required. The network port of the source Oracle database.
*/
@JvmName("outgovoivyjveace")
public suspend fun port(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.port = mapped
}
/**
* @param value Configuration for using a private network to communicate with the source database
* Structure is documented below.
*/
@JvmName("nxrkncvfvygyfvsg")
public suspend fun privateConnectivity(`value`: ConnectionProfileOraclePrivateConnectivityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateConnectivity = mapped
}
/**
* @param argument Configuration for using a private network to communicate with the source database
* Structure is documented below.
*/
@JvmName("lpwvjnitisxwianx")
public suspend fun privateConnectivity(argument: suspend ConnectionProfileOraclePrivateConnectivityArgsBuilder.() -> Unit) {
val toBeMapped = ConnectionProfileOraclePrivateConnectivityArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.privateConnectivity = mapped
}
/**
* @param value SSL configuration for the destination to connect to the source database.
* Structure is documented below.
*/
@JvmName("mpojmvmpvlhvbklf")
public suspend fun ssl(`value`: ConnectionProfileOracleSslArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ssl = mapped
}
/**
* @param argument SSL configuration for the destination to connect to the source database.
* Structure is documented below.
*/
@JvmName("hxolcgxuaichtaju")
public suspend fun ssl(argument: suspend ConnectionProfileOracleSslArgsBuilder.() -> Unit) {
val toBeMapped = ConnectionProfileOracleSslArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.ssl = mapped
}
/**
* @param value This object has no nested fields.
* Static IP address connectivity configured on service project.
*/
@JvmName("tvqmidqohwrfabas")
public suspend fun staticServiceIpConnectivity(`value`: ConnectionProfileOracleStaticServiceIpConnectivityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.staticServiceIpConnectivity = mapped
}
/**
* @param argument This object has no nested fields.
* Static IP address connectivity configured on service project.
*/
@JvmName("eueibcbshfcbiewm")
public suspend fun staticServiceIpConnectivity(argument: suspend ConnectionProfileOracleStaticServiceIpConnectivityArgsBuilder.() -> Unit) {
val toBeMapped = ConnectionProfileOracleStaticServiceIpConnectivityArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.staticServiceIpConnectivity = mapped
}
/**
* @param value Required. The username that Database Migration Service will use to connect to the database. The value is encrypted when stored in Database Migration Service.
*/
@JvmName("iqshehatytdvwcmq")
public suspend fun username(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.username = mapped
}
internal fun build(): ConnectionProfileOracleArgs = ConnectionProfileOracleArgs(
databaseService = databaseService ?: throw PulumiNullFieldException("databaseService"),
forwardSshConnectivity = forwardSshConnectivity,
host = host ?: throw PulumiNullFieldException("host"),
password = password ?: throw PulumiNullFieldException("password"),
passwordSet = passwordSet,
port = port ?: throw PulumiNullFieldException("port"),
privateConnectivity = privateConnectivity,
ssl = ssl,
staticServiceIpConnectivity = staticServiceIpConnectivity,
username = username ?: throw PulumiNullFieldException("username"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy