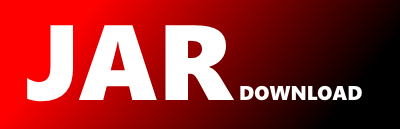
com.pulumi.gcp.datacatalog.kotlin.EntryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.datacatalog.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.datacatalog.EntryArgs.builder
import com.pulumi.gcp.datacatalog.kotlin.inputs.EntryGcsFilesetSpecArgs
import com.pulumi.gcp.datacatalog.kotlin.inputs.EntryGcsFilesetSpecArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Entry Metadata. A Data Catalog Entry resource represents another resource in Google Cloud Platform
* (such as a BigQuery dataset or a Pub/Sub topic) or outside of Google Cloud Platform. Clients can use
* the linkedResource field in the Entry resource to refer to the original resource ID of the source system.
* An Entry resource contains resource details, such as its schema. An Entry can also be used to attach
* flexible metadata, such as a Tag.
* To get more information about Entry, see:
* * [API documentation](https://cloud.google.com/data-catalog/docs/reference/rest/v1/projects.locations.entryGroups.entries)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/data-catalog/docs)
* ## Example Usage
* ### Data Catalog Entry Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const entryGroup = new gcp.datacatalog.EntryGroup("entry_group", {entryGroupId: "my_group"});
* const basicEntry = new gcp.datacatalog.Entry("basic_entry", {
* entryGroup: entryGroup.id,
* entryId: "my_entry",
* userSpecifiedType: "my_custom_type",
* userSpecifiedSystem: "SomethingExternal",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* entry_group = gcp.datacatalog.EntryGroup("entry_group", entry_group_id="my_group")
* basic_entry = gcp.datacatalog.Entry("basic_entry",
* entry_group=entry_group.id,
* entry_id="my_entry",
* user_specified_type="my_custom_type",
* user_specified_system="SomethingExternal")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var entryGroup = new Gcp.DataCatalog.EntryGroup("entry_group", new()
* {
* EntryGroupId = "my_group",
* });
* var basicEntry = new Gcp.DataCatalog.Entry("basic_entry", new()
* {
* EntryGroup = entryGroup.Id,
* EntryId = "my_entry",
* UserSpecifiedType = "my_custom_type",
* UserSpecifiedSystem = "SomethingExternal",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/datacatalog"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* entryGroup, err := datacatalog.NewEntryGroup(ctx, "entry_group", &datacatalog.EntryGroupArgs{
* EntryGroupId: pulumi.String("my_group"),
* })
* if err != nil {
* return err
* }
* _, err = datacatalog.NewEntry(ctx, "basic_entry", &datacatalog.EntryArgs{
* EntryGroup: entryGroup.ID(),
* EntryId: pulumi.String("my_entry"),
* UserSpecifiedType: pulumi.String("my_custom_type"),
* UserSpecifiedSystem: pulumi.String("SomethingExternal"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.datacatalog.EntryGroup;
* import com.pulumi.gcp.datacatalog.EntryGroupArgs;
* import com.pulumi.gcp.datacatalog.Entry;
* import com.pulumi.gcp.datacatalog.EntryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var entryGroup = new EntryGroup("entryGroup", EntryGroupArgs.builder()
* .entryGroupId("my_group")
* .build());
* var basicEntry = new Entry("basicEntry", EntryArgs.builder()
* .entryGroup(entryGroup.id())
* .entryId("my_entry")
* .userSpecifiedType("my_custom_type")
* .userSpecifiedSystem("SomethingExternal")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* basicEntry:
* type: gcp:datacatalog:Entry
* name: basic_entry
* properties:
* entryGroup: ${entryGroup.id}
* entryId: my_entry
* userSpecifiedType: my_custom_type
* userSpecifiedSystem: SomethingExternal
* entryGroup:
* type: gcp:datacatalog:EntryGroup
* name: entry_group
* properties:
* entryGroupId: my_group
* ```
*
* ### Data Catalog Entry Fileset
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const entryGroup = new gcp.datacatalog.EntryGroup("entry_group", {entryGroupId: "my_group"});
* const basicEntry = new gcp.datacatalog.Entry("basic_entry", {
* entryGroup: entryGroup.id,
* entryId: "my_entry",
* type: "FILESET",
* gcsFilesetSpec: {
* filePatterns: ["gs://fake_bucket/dir/*"],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* entry_group = gcp.datacatalog.EntryGroup("entry_group", entry_group_id="my_group")
* basic_entry = gcp.datacatalog.Entry("basic_entry",
* entry_group=entry_group.id,
* entry_id="my_entry",
* type="FILESET",
* gcs_fileset_spec=gcp.datacatalog.EntryGcsFilesetSpecArgs(
* file_patterns=["gs://fake_bucket/dir/*"],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var entryGroup = new Gcp.DataCatalog.EntryGroup("entry_group", new()
* {
* EntryGroupId = "my_group",
* });
* var basicEntry = new Gcp.DataCatalog.Entry("basic_entry", new()
* {
* EntryGroup = entryGroup.Id,
* EntryId = "my_entry",
* Type = "FILESET",
* GcsFilesetSpec = new Gcp.DataCatalog.Inputs.EntryGcsFilesetSpecArgs
* {
* FilePatterns = new[]
* {
* "gs://fake_bucket/dir/*",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/datacatalog"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* entryGroup, err := datacatalog.NewEntryGroup(ctx, "entry_group", &datacatalog.EntryGroupArgs{
* EntryGroupId: pulumi.String("my_group"),
* })
* if err != nil {
* return err
* }
* _, err = datacatalog.NewEntry(ctx, "basic_entry", &datacatalog.EntryArgs{
* EntryGroup: entryGroup.ID(),
* EntryId: pulumi.String("my_entry"),
* Type: pulumi.String("FILESET"),
* GcsFilesetSpec: &datacatalog.EntryGcsFilesetSpecArgs{
* FilePatterns: pulumi.StringArray{
* pulumi.String("gs://fake_bucket/dir/*"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.datacatalog.EntryGroup;
* import com.pulumi.gcp.datacatalog.EntryGroupArgs;
* import com.pulumi.gcp.datacatalog.Entry;
* import com.pulumi.gcp.datacatalog.EntryArgs;
* import com.pulumi.gcp.datacatalog.inputs.EntryGcsFilesetSpecArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var entryGroup = new EntryGroup("entryGroup", EntryGroupArgs.builder()
* .entryGroupId("my_group")
* .build());
* var basicEntry = new Entry("basicEntry", EntryArgs.builder()
* .entryGroup(entryGroup.id())
* .entryId("my_entry")
* .type("FILESET")
* .gcsFilesetSpec(EntryGcsFilesetSpecArgs.builder()
* .filePatterns("gs://fake_bucket/dir/*")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* basicEntry:
* type: gcp:datacatalog:Entry
* name: basic_entry
* properties:
* entryGroup: ${entryGroup.id}
* entryId: my_entry
* type: FILESET
* gcsFilesetSpec:
* filePatterns:
* - gs://fake_bucket/dir/*
* entryGroup:
* type: gcp:datacatalog:EntryGroup
* name: entry_group
* properties:
* entryGroupId: my_group
* ```
*
* ### Data Catalog Entry Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const entryGroup = new gcp.datacatalog.EntryGroup("entry_group", {entryGroupId: "my_group"});
* const basicEntry = new gcp.datacatalog.Entry("basic_entry", {
* entryGroup: entryGroup.id,
* entryId: "my_entry",
* userSpecifiedType: "my_user_specified_type",
* userSpecifiedSystem: "Something_custom",
* linkedResource: "my/linked/resource",
* displayName: "my custom type entry",
* description: "a custom type entry for a user specified system",
* schema: `{
* "columns": [
* {
* "column": "first_name",
* "description": "First name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "last_name",
* "description": "Last name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "address",
* "description": "Address",
* "mode": "REPEATED",
* "subcolumns": [
* {
* "column": "city",
* "description": "City",
* "mode": "NULLABLE",
* "type": "STRING"
* },
* {
* "column": "state",
* "description": "State",
* "mode": "NULLABLE",
* "type": "STRING"
* }
* ],
* "type": "RECORD"
* }
* ]
* }
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* entry_group = gcp.datacatalog.EntryGroup("entry_group", entry_group_id="my_group")
* basic_entry = gcp.datacatalog.Entry("basic_entry",
* entry_group=entry_group.id,
* entry_id="my_entry",
* user_specified_type="my_user_specified_type",
* user_specified_system="Something_custom",
* linked_resource="my/linked/resource",
* display_name="my custom type entry",
* description="a custom type entry for a user specified system",
* schema="""{
* "columns": [
* {
* "column": "first_name",
* "description": "First name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "last_name",
* "description": "Last name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "address",
* "description": "Address",
* "mode": "REPEATED",
* "subcolumns": [
* {
* "column": "city",
* "description": "City",
* "mode": "NULLABLE",
* "type": "STRING"
* },
* {
* "column": "state",
* "description": "State",
* "mode": "NULLABLE",
* "type": "STRING"
* }
* ],
* "type": "RECORD"
* }
* ]
* }
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var entryGroup = new Gcp.DataCatalog.EntryGroup("entry_group", new()
* {
* EntryGroupId = "my_group",
* });
* var basicEntry = new Gcp.DataCatalog.Entry("basic_entry", new()
* {
* EntryGroup = entryGroup.Id,
* EntryId = "my_entry",
* UserSpecifiedType = "my_user_specified_type",
* UserSpecifiedSystem = "Something_custom",
* LinkedResource = "my/linked/resource",
* DisplayName = "my custom type entry",
* Description = "a custom type entry for a user specified system",
* Schema = @"{
* ""columns"": [
* {
* ""column"": ""first_name"",
* ""description"": ""First name"",
* ""mode"": ""REQUIRED"",
* ""type"": ""STRING""
* },
* {
* ""column"": ""last_name"",
* ""description"": ""Last name"",
* ""mode"": ""REQUIRED"",
* ""type"": ""STRING""
* },
* {
* ""column"": ""address"",
* ""description"": ""Address"",
* ""mode"": ""REPEATED"",
* ""subcolumns"": [
* {
* ""column"": ""city"",
* ""description"": ""City"",
* ""mode"": ""NULLABLE"",
* ""type"": ""STRING""
* },
* {
* ""column"": ""state"",
* ""description"": ""State"",
* ""mode"": ""NULLABLE"",
* ""type"": ""STRING""
* }
* ],
* ""type"": ""RECORD""
* }
* ]
* }
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/datacatalog"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* entryGroup, err := datacatalog.NewEntryGroup(ctx, "entry_group", &datacatalog.EntryGroupArgs{
* EntryGroupId: pulumi.String("my_group"),
* })
* if err != nil {
* return err
* }
* _, err = datacatalog.NewEntry(ctx, "basic_entry", &datacatalog.EntryArgs{
* EntryGroup: entryGroup.ID(),
* EntryId: pulumi.String("my_entry"),
* UserSpecifiedType: pulumi.String("my_user_specified_type"),
* UserSpecifiedSystem: pulumi.String("Something_custom"),
* LinkedResource: pulumi.String("my/linked/resource"),
* DisplayName: pulumi.String("my custom type entry"),
* Description: pulumi.String("a custom type entry for a user specified system"),
* Schema: pulumi.String(`{
* "columns": [
* {
* "column": "first_name",
* "description": "First name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "last_name",
* "description": "Last name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "address",
* "description": "Address",
* "mode": "REPEATED",
* "subcolumns": [
* {
* "column": "city",
* "description": "City",
* "mode": "NULLABLE",
* "type": "STRING"
* },
* {
* "column": "state",
* "description": "State",
* "mode": "NULLABLE",
* "type": "STRING"
* }
* ],
* "type": "RECORD"
* }
* ]
* }
* `),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.datacatalog.EntryGroup;
* import com.pulumi.gcp.datacatalog.EntryGroupArgs;
* import com.pulumi.gcp.datacatalog.Entry;
* import com.pulumi.gcp.datacatalog.EntryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var entryGroup = new EntryGroup("entryGroup", EntryGroupArgs.builder()
* .entryGroupId("my_group")
* .build());
* var basicEntry = new Entry("basicEntry", EntryArgs.builder()
* .entryGroup(entryGroup.id())
* .entryId("my_entry")
* .userSpecifiedType("my_user_specified_type")
* .userSpecifiedSystem("Something_custom")
* .linkedResource("my/linked/resource")
* .displayName("my custom type entry")
* .description("a custom type entry for a user specified system")
* .schema("""
* {
* "columns": [
* {
* "column": "first_name",
* "description": "First name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "last_name",
* "description": "Last name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "address",
* "description": "Address",
* "mode": "REPEATED",
* "subcolumns": [
* {
* "column": "city",
* "description": "City",
* "mode": "NULLABLE",
* "type": "STRING"
* },
* {
* "column": "state",
* "description": "State",
* "mode": "NULLABLE",
* "type": "STRING"
* }
* ],
* "type": "RECORD"
* }
* ]
* }
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* basicEntry:
* type: gcp:datacatalog:Entry
* name: basic_entry
* properties:
* entryGroup: ${entryGroup.id}
* entryId: my_entry
* userSpecifiedType: my_user_specified_type
* userSpecifiedSystem: Something_custom
* linkedResource: my/linked/resource
* displayName: my custom type entry
* description: a custom type entry for a user specified system
* schema: |
* {
* "columns": [
* {
* "column": "first_name",
* "description": "First name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "last_name",
* "description": "Last name",
* "mode": "REQUIRED",
* "type": "STRING"
* },
* {
* "column": "address",
* "description": "Address",
* "mode": "REPEATED",
* "subcolumns": [
* {
* "column": "city",
* "description": "City",
* "mode": "NULLABLE",
* "type": "STRING"
* },
* {
* "column": "state",
* "description": "State",
* "mode": "NULLABLE",
* "type": "STRING"
* }
* ],
* "type": "RECORD"
* }
* ]
* }
* entryGroup:
* type: gcp:datacatalog:EntryGroup
* name: entry_group
* properties:
* entryGroupId: my_group
* ```
*
* ## Import
* Entry can be imported using any of these accepted formats:
* * `{{name}}`
* When using the `pulumi import` command, Entry can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:datacatalog/entry:Entry default {{name}}
* ```
* @property description Entry description, which can consist of several sentences or paragraphs that describe entry contents.
* @property displayName Display information such as title and description. A short name to identify the entry,
* for example, "Analytics Data - Jan 2011".
* @property entryGroup The name of the entry group this entry is in.
* @property entryId The id of the entry to create.
* - - -
* @property gcsFilesetSpec Specification that applies to a Cloud Storage fileset. This is only valid on entries of type FILESET.
* Structure is documented below.
* @property linkedResource The resource this metadata entry refers to.
* For Google Cloud Platform resources, linkedResource is the full name of the resource.
* For example, the linkedResource for a table resource from BigQuery is:
* //bigquery.googleapis.com/projects/projectId/datasets/datasetId/tables/tableId
* Output only when Entry is of type in the EntryType enum. For entries with userSpecifiedType,
* this field is optional and defaults to an empty string.
* @property schema Schema of the entry (e.g. BigQuery, GoogleSQL, Avro schema), as a json string. An entry might not have any schema
* attached to it. See
* https://cloud.google.com/data-catalog/docs/reference/rest/v1/projects.locations.entryGroups.entries#schema
* for what fields this schema can contain.
* @property type The type of the entry. Only used for Entries with types in the EntryType enum.
* Currently, only FILESET enum value is allowed. All other entries created through Data Catalog must use userSpecifiedType.
* Possible values are: `FILESET`.
* @property userSpecifiedSystem This field indicates the entry's source system that Data Catalog does not integrate with.
* userSpecifiedSystem strings must begin with a letter or underscore and can only contain letters, numbers,
* and underscores; are case insensitive; must be at least 1 character and at most 64 characters long.
* @property userSpecifiedType Entry type if it does not fit any of the input-allowed values listed in EntryType enum above.
* When creating an entry, users should check the enum values first, if nothing matches the entry
* to be created, then provide a custom value, for example "my_special_type".
* userSpecifiedType strings must begin with a letter or underscore and can only contain letters,
* numbers, and underscores; are case insensitive; must be at least 1 character and at most 64 characters long.
* */*/*/*/*/*/
*/
public data class EntryArgs(
public val description: Output? = null,
public val displayName: Output? = null,
public val entryGroup: Output? = null,
public val entryId: Output? = null,
public val gcsFilesetSpec: Output? = null,
public val linkedResource: Output? = null,
public val schema: Output? = null,
public val type: Output? = null,
public val userSpecifiedSystem: Output? = null,
public val userSpecifiedType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.datacatalog.EntryArgs =
com.pulumi.gcp.datacatalog.EntryArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.entryGroup(entryGroup?.applyValue({ args0 -> args0 }))
.entryId(entryId?.applyValue({ args0 -> args0 }))
.gcsFilesetSpec(gcsFilesetSpec?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.linkedResource(linkedResource?.applyValue({ args0 -> args0 }))
.schema(schema?.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 }))
.userSpecifiedSystem(userSpecifiedSystem?.applyValue({ args0 -> args0 }))
.userSpecifiedType(userSpecifiedType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EntryArgs].
*/
@PulumiTagMarker
public class EntryArgsBuilder internal constructor() {
private var description: Output? = null
private var displayName: Output? = null
private var entryGroup: Output? = null
private var entryId: Output? = null
private var gcsFilesetSpec: Output? = null
private var linkedResource: Output? = null
private var schema: Output? = null
private var type: Output? = null
private var userSpecifiedSystem: Output? = null
private var userSpecifiedType: Output? = null
/**
* @param value Entry description, which can consist of several sentences or paragraphs that describe entry contents.
*/
@JvmName("tihiqssdunduurnt")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Display information such as title and description. A short name to identify the entry,
* for example, "Analytics Data - Jan 2011".
*/
@JvmName("bdvrypfennhlbojf")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value The name of the entry group this entry is in.
*/
@JvmName("qutplpjchtblarsh")
public suspend fun entryGroup(`value`: Output) {
this.entryGroup = value
}
/**
* @param value The id of the entry to create.
* - - -
*/
@JvmName("ocrthicmyvvnrdud")
public suspend fun entryId(`value`: Output) {
this.entryId = value
}
/**
* @param value Specification that applies to a Cloud Storage fileset. This is only valid on entries of type FILESET.
* Structure is documented below.
*/
@JvmName("dcitkmugtowftjsw")
public suspend fun gcsFilesetSpec(`value`: Output) {
this.gcsFilesetSpec = value
}
/**
* @param value The resource this metadata entry refers to.
* For Google Cloud Platform resources, linkedResource is the full name of the resource.
* For example, the linkedResource for a table resource from BigQuery is:
* //bigquery.googleapis.com/projects/projectId/datasets/datasetId/tables/tableId
* Output only when Entry is of type in the EntryType enum. For entries with userSpecifiedType,
* this field is optional and defaults to an empty string.
*/
@JvmName("scwapjfqcjpnbfkk")
public suspend fun linkedResource(`value`: Output) {
this.linkedResource = value
}
/**
* @param value Schema of the entry (e.g. BigQuery, GoogleSQL, Avro schema), as a json string. An entry might not have any schema
* attached to it. See
* https://cloud.google.com/data-catalog/docs/reference/rest/v1/projects.locations.entryGroups.entries#schema
* for what fields this schema can contain.
*/
@JvmName("ddbtbowpokcewthd")
public suspend fun schema(`value`: Output) {
this.schema = value
}
/**
* @param value The type of the entry. Only used for Entries with types in the EntryType enum.
* Currently, only FILESET enum value is allowed. All other entries created through Data Catalog must use userSpecifiedType.
* Possible values are: `FILESET`.
*/
@JvmName("jnkgjkswmwlcsfux")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value This field indicates the entry's source system that Data Catalog does not integrate with.
* userSpecifiedSystem strings must begin with a letter or underscore and can only contain letters, numbers,
* and underscores; are case insensitive; must be at least 1 character and at most 64 characters long.
*/
@JvmName("nysutfhsadedhvgu")
public suspend fun userSpecifiedSystem(`value`: Output) {
this.userSpecifiedSystem = value
}
/**
* @param value Entry type if it does not fit any of the input-allowed values listed in EntryType enum above.
* When creating an entry, users should check the enum values first, if nothing matches the entry
* to be created, then provide a custom value, for example "my_special_type".
* userSpecifiedType strings must begin with a letter or underscore and can only contain letters,
* numbers, and underscores; are case insensitive; must be at least 1 character and at most 64 characters long.
*/
@JvmName("irdbbhkjsmavaddj")
public suspend fun userSpecifiedType(`value`: Output) {
this.userSpecifiedType = value
}
/**
* @param value Entry description, which can consist of several sentences or paragraphs that describe entry contents.
*/
@JvmName("lhvoxffcunlwugwu")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Display information such as title and description. A short name to identify the entry,
* for example, "Analytics Data - Jan 2011".
*/
@JvmName("hncgqsndjemlthik")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value The name of the entry group this entry is in.
*/
@JvmName("sevsehkljiixatwl")
public suspend fun entryGroup(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entryGroup = mapped
}
/**
* @param value The id of the entry to create.
* - - -
*/
@JvmName("ljaittsxuxauryhw")
public suspend fun entryId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entryId = mapped
}
/**
* @param value Specification that applies to a Cloud Storage fileset. This is only valid on entries of type FILESET.
* Structure is documented below.
*/
@JvmName("koonofeaibiycrik")
public suspend fun gcsFilesetSpec(`value`: EntryGcsFilesetSpecArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcsFilesetSpec = mapped
}
/**
* @param argument Specification that applies to a Cloud Storage fileset. This is only valid on entries of type FILESET.
* Structure is documented below.
*/
@JvmName("hxxylocgddcrelik")
public suspend fun gcsFilesetSpec(argument: suspend EntryGcsFilesetSpecArgsBuilder.() -> Unit) {
val toBeMapped = EntryGcsFilesetSpecArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.gcsFilesetSpec = mapped
}
/**
* @param value The resource this metadata entry refers to.
* For Google Cloud Platform resources, linkedResource is the full name of the resource.
* For example, the linkedResource for a table resource from BigQuery is:
* //bigquery.googleapis.com/projects/projectId/datasets/datasetId/tables/tableId
* Output only when Entry is of type in the EntryType enum. For entries with userSpecifiedType,
* this field is optional and defaults to an empty string.
*/
@JvmName("kjmuvncniavxllup")
public suspend fun linkedResource(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.linkedResource = mapped
}
/**
* @param value Schema of the entry (e.g. BigQuery, GoogleSQL, Avro schema), as a json string. An entry might not have any schema
* attached to it. See
* https://cloud.google.com/data-catalog/docs/reference/rest/v1/projects.locations.entryGroups.entries#schema
* for what fields this schema can contain.
*/
@JvmName("ndxxvxvtgsjtewli")
public suspend fun schema(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.schema = mapped
}
/**
* @param value The type of the entry. Only used for Entries with types in the EntryType enum.
* Currently, only FILESET enum value is allowed. All other entries created through Data Catalog must use userSpecifiedType.
* Possible values are: `FILESET`.
*/
@JvmName("ilnltbcwrgpnuyhe")
public suspend fun type(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
/**
* @param value This field indicates the entry's source system that Data Catalog does not integrate with.
* userSpecifiedSystem strings must begin with a letter or underscore and can only contain letters, numbers,
* and underscores; are case insensitive; must be at least 1 character and at most 64 characters long.
*/
@JvmName("fgbifxyeshajxnwk")
public suspend fun userSpecifiedSystem(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userSpecifiedSystem = mapped
}
/**
* @param value Entry type if it does not fit any of the input-allowed values listed in EntryType enum above.
* When creating an entry, users should check the enum values first, if nothing matches the entry
* to be created, then provide a custom value, for example "my_special_type".
* userSpecifiedType strings must begin with a letter or underscore and can only contain letters,
* numbers, and underscores; are case insensitive; must be at least 1 character and at most 64 characters long.
*/
@JvmName("qdtthjutllxpfwkn")
public suspend fun userSpecifiedType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userSpecifiedType = mapped
}
internal fun build(): EntryArgs = EntryArgs(
description = description,
displayName = displayName,
entryGroup = entryGroup,
entryId = entryId,
gcsFilesetSpec = gcsFilesetSpec,
linkedResource = linkedResource,
schema = schema,
type = type,
userSpecifiedSystem = userSpecifiedSystem,
userSpecifiedType = userSpecifiedType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy