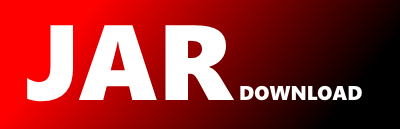
com.pulumi.gcp.dataflow.kotlin.inputs.PipelineWorkloadDataflowLaunchTemplateRequestArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataflow.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataflow.inputs.PipelineWorkloadDataflowLaunchTemplateRequestArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property gcsPath A Cloud Storage path to the template from which to create the job. Must be a valid Cloud Storage URL, beginning with 'gs://'.
* @property launchParameters The parameters of the template to launch. This should be part of the body of the POST request.
* https://cloud.google.com/dataflow/docs/reference/data-pipelines/rest/v1/projects.locations.pipelines#launchtemplateparameters
* Structure is documented below.
* @property location The regional endpoint to which to direct the request.
* @property projectId The ID of the Cloud Platform project that the job belongs to.
* @property validateOnly (Optional)
*/
public data class PipelineWorkloadDataflowLaunchTemplateRequestArgs(
public val gcsPath: Output? = null,
public val launchParameters: Output? = null,
public val location: Output? = null,
public val projectId: Output,
public val validateOnly: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataflow.inputs.PipelineWorkloadDataflowLaunchTemplateRequestArgs =
com.pulumi.gcp.dataflow.inputs.PipelineWorkloadDataflowLaunchTemplateRequestArgs.builder()
.gcsPath(gcsPath?.applyValue({ args0 -> args0 }))
.launchParameters(launchParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.location(location?.applyValue({ args0 -> args0 }))
.projectId(projectId.applyValue({ args0 -> args0 }))
.validateOnly(validateOnly?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PipelineWorkloadDataflowLaunchTemplateRequestArgs].
*/
@PulumiTagMarker
public class PipelineWorkloadDataflowLaunchTemplateRequestArgsBuilder internal constructor() {
private var gcsPath: Output? = null
private var launchParameters:
Output? = null
private var location: Output? = null
private var projectId: Output? = null
private var validateOnly: Output? = null
/**
* @param value A Cloud Storage path to the template from which to create the job. Must be a valid Cloud Storage URL, beginning with 'gs://'.
*/
@JvmName("sjvfdoqrowkvnsdq")
public suspend fun gcsPath(`value`: Output) {
this.gcsPath = value
}
/**
* @param value The parameters of the template to launch. This should be part of the body of the POST request.
* https://cloud.google.com/dataflow/docs/reference/data-pipelines/rest/v1/projects.locations.pipelines#launchtemplateparameters
* Structure is documented below.
*/
@JvmName("rtbojusuusfxdcav")
public suspend fun launchParameters(`value`: Output) {
this.launchParameters = value
}
/**
* @param value The regional endpoint to which to direct the request.
*/
@JvmName("kymegqlydcaigose")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The ID of the Cloud Platform project that the job belongs to.
*/
@JvmName("gjjcufuxdohcwpxr")
public suspend fun projectId(`value`: Output) {
this.projectId = value
}
/**
* @param value (Optional)
*/
@JvmName("swntudmydlpvhjwx")
public suspend fun validateOnly(`value`: Output) {
this.validateOnly = value
}
/**
* @param value A Cloud Storage path to the template from which to create the job. Must be a valid Cloud Storage URL, beginning with 'gs://'.
*/
@JvmName("pdnhrpvwcukqtnrb")
public suspend fun gcsPath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcsPath = mapped
}
/**
* @param value The parameters of the template to launch. This should be part of the body of the POST request.
* https://cloud.google.com/dataflow/docs/reference/data-pipelines/rest/v1/projects.locations.pipelines#launchtemplateparameters
* Structure is documented below.
*/
@JvmName("qyyaiwkidduunftu")
public suspend fun launchParameters(`value`: PipelineWorkloadDataflowLaunchTemplateRequestLaunchParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.launchParameters = mapped
}
/**
* @param argument The parameters of the template to launch. This should be part of the body of the POST request.
* https://cloud.google.com/dataflow/docs/reference/data-pipelines/rest/v1/projects.locations.pipelines#launchtemplateparameters
* Structure is documented below.
*/
@JvmName("qgaoxyvpjnytulcv")
public suspend fun launchParameters(argument: suspend PipelineWorkloadDataflowLaunchTemplateRequestLaunchParametersArgsBuilder.() -> Unit) {
val toBeMapped =
PipelineWorkloadDataflowLaunchTemplateRequestLaunchParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.launchParameters = mapped
}
/**
* @param value The regional endpoint to which to direct the request.
*/
@JvmName("oexahiblxapvudvm")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value The ID of the Cloud Platform project that the job belongs to.
*/
@JvmName("gwbiskffagbnvdpb")
public suspend fun projectId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.projectId = mapped
}
/**
* @param value (Optional)
*/
@JvmName("asipoxnemqcvxjjk")
public suspend fun validateOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validateOnly = mapped
}
internal fun build(): PipelineWorkloadDataflowLaunchTemplateRequestArgs =
PipelineWorkloadDataflowLaunchTemplateRequestArgs(
gcsPath = gcsPath,
launchParameters = launchParameters,
location = location,
projectId = projectId ?: throw PulumiNullFieldException("projectId"),
validateOnly = validateOnly,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy