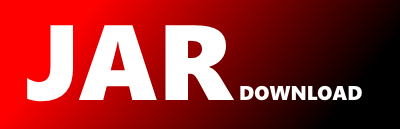
com.pulumi.gcp.dataloss.kotlin.PreventionDiscoveryConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataloss.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.dataloss.kotlin.outputs.PreventionDiscoveryConfigAction
import com.pulumi.gcp.dataloss.kotlin.outputs.PreventionDiscoveryConfigError
import com.pulumi.gcp.dataloss.kotlin.outputs.PreventionDiscoveryConfigOrgConfig
import com.pulumi.gcp.dataloss.kotlin.outputs.PreventionDiscoveryConfigTarget
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gcp.dataloss.kotlin.outputs.PreventionDiscoveryConfigAction.Companion.toKotlin as preventionDiscoveryConfigActionToKotlin
import com.pulumi.gcp.dataloss.kotlin.outputs.PreventionDiscoveryConfigError.Companion.toKotlin as preventionDiscoveryConfigErrorToKotlin
import com.pulumi.gcp.dataloss.kotlin.outputs.PreventionDiscoveryConfigOrgConfig.Companion.toKotlin as preventionDiscoveryConfigOrgConfigToKotlin
import com.pulumi.gcp.dataloss.kotlin.outputs.PreventionDiscoveryConfigTarget.Companion.toKotlin as preventionDiscoveryConfigTargetToKotlin
/**
* Builder for [PreventionDiscoveryConfig].
*/
@PulumiTagMarker
public class PreventionDiscoveryConfigResourceBuilder internal constructor() {
public var name: String? = null
public var args: PreventionDiscoveryConfigArgs = PreventionDiscoveryConfigArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend PreventionDiscoveryConfigArgsBuilder.() -> Unit) {
val builder = PreventionDiscoveryConfigArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): PreventionDiscoveryConfig {
val builtJavaResource =
com.pulumi.gcp.dataloss.PreventionDiscoveryConfig(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return PreventionDiscoveryConfig(builtJavaResource)
}
}
/**
* Configuration for discovery to scan resources for profile generation. Only one discovery configuration may exist per organization, folder, or project.
* To get more information about DiscoveryConfig, see:
* * [API documentation](https://cloud.google.com/dlp/docs/reference/rest/v2/projects.locations.discoveryConfigs)
* * How-to Guides
* * [Schedule inspection scan](https://cloud.google.com/dlp/docs/schedule-inspection-scan)
* ## Example Usage
* ## Import
* DiscoveryConfig can be imported using any of these accepted formats:
* * `{{parent}}/discoveryConfigs/{{name}}`
* * `{{parent}}/{{name}}`
* When using the `pulumi import` command, DiscoveryConfig can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:dataloss/preventionDiscoveryConfig:PreventionDiscoveryConfig default {{parent}}/discoveryConfigs/{{name}}
* ```
* ```sh
* $ pulumi import gcp:dataloss/preventionDiscoveryConfig:PreventionDiscoveryConfig default {{parent}}/{{name}}
* ```
*/
public class PreventionDiscoveryConfig internal constructor(
override val javaResource: com.pulumi.gcp.dataloss.PreventionDiscoveryConfig,
) : KotlinCustomResource(javaResource, PreventionDiscoveryConfigMapper) {
/**
* Actions to execute at the completion of scanning
* Structure is documented below.
*/
public val actions: Output>?
get() = javaResource.actions().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> preventionDiscoveryConfigActionToKotlin(args0) })
})
}).orElse(null)
})
/**
* Output only. The creation timestamp of a DiscoveryConfig.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* Display Name (max 1000 Chars)
*/
public val displayName: Output?
get() = javaResource.displayName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Output only. A stream of errors encountered when the config was activated. Repeated errors may result in the config automatically being paused. Output only field. Will return the last 100 errors. Whenever the config is modified this list will be cleared.
* Structure is documented below.
*/
public val errors: Output>
get() = javaResource.errors().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
preventionDiscoveryConfigErrorToKotlin(args0)
})
})
})
/**
* Detection logic for profile generation
*/
public val inspectTemplates: Output>?
get() = javaResource.inspectTemplates().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Output only. The timestamp of the last time this config was executed
*/
public val lastRunTime: Output
get() = javaResource.lastRunTime().applyValue({ args0 -> args0 })
/**
* Location to create the discovery config in.
* - - -
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Unique resource name for the DiscoveryConfig, assigned by the service when the DiscoveryConfig is created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* A nested object resource
* Structure is documented below.
*/
public val orgConfig: Output?
get() = javaResource.orgConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
preventionDiscoveryConfigOrgConfigToKotlin(args0)
})
}).orElse(null)
})
/**
* The parent of the discovery config in any of the following formats:
* * `projects/{{project}}/locations/{{location}}`
* * `organizations/{{organization_id}}/locations/{{location}}`
*/
public val parent: Output
get() = javaResource.parent().applyValue({ args0 -> args0 })
/**
* Required. A status for this configuration
* Possible values are: `RUNNING`, `PAUSED`.
*/
public val status: Output?
get() = javaResource.status().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Target to match against for determining what to scan and how frequently
* Structure is documented below.
*/
public val targets: Output>?
get() = javaResource.targets().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> preventionDiscoveryConfigTargetToKotlin(args0) })
})
}).orElse(null)
})
/**
* Output only. The last update timestamp of a DiscoveryConfig.
*/
public val updateTime: Output
get() = javaResource.updateTime().applyValue({ args0 -> args0 })
}
public object PreventionDiscoveryConfigMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.dataloss.PreventionDiscoveryConfig::class == javaResource::class
override fun map(javaResource: Resource): PreventionDiscoveryConfig =
PreventionDiscoveryConfig(javaResource as com.pulumi.gcp.dataloss.PreventionDiscoveryConfig)
}
/**
* @see [PreventionDiscoveryConfig].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [PreventionDiscoveryConfig].
*/
public suspend fun preventionDiscoveryConfig(
name: String,
block: suspend PreventionDiscoveryConfigResourceBuilder.() -> Unit,
): PreventionDiscoveryConfig {
val builder = PreventionDiscoveryConfigResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [PreventionDiscoveryConfig].
* @param name The _unique_ name of the resulting resource.
*/
public fun preventionDiscoveryConfig(name: String): PreventionDiscoveryConfig {
val builder = PreventionDiscoveryConfigResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy