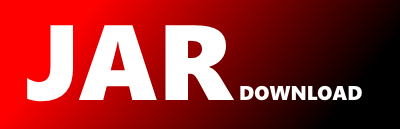
com.pulumi.gcp.dataloss.kotlin.PreventionDiscoveryConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataloss.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataloss.PreventionDiscoveryConfigArgs.builder
import com.pulumi.gcp.dataloss.kotlin.inputs.PreventionDiscoveryConfigActionArgs
import com.pulumi.gcp.dataloss.kotlin.inputs.PreventionDiscoveryConfigActionArgsBuilder
import com.pulumi.gcp.dataloss.kotlin.inputs.PreventionDiscoveryConfigOrgConfigArgs
import com.pulumi.gcp.dataloss.kotlin.inputs.PreventionDiscoveryConfigOrgConfigArgsBuilder
import com.pulumi.gcp.dataloss.kotlin.inputs.PreventionDiscoveryConfigTargetArgs
import com.pulumi.gcp.dataloss.kotlin.inputs.PreventionDiscoveryConfigTargetArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Configuration for discovery to scan resources for profile generation. Only one discovery configuration may exist per organization, folder, or project.
* To get more information about DiscoveryConfig, see:
* * [API documentation](https://cloud.google.com/dlp/docs/reference/rest/v2/projects.locations.discoveryConfigs)
* * How-to Guides
* * [Schedule inspection scan](https://cloud.google.com/dlp/docs/schedule-inspection-scan)
* ## Example Usage
* ## Import
* DiscoveryConfig can be imported using any of these accepted formats:
* * `{{parent}}/discoveryConfigs/{{name}}`
* * `{{parent}}/{{name}}`
* When using the `pulumi import` command, DiscoveryConfig can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:dataloss/preventionDiscoveryConfig:PreventionDiscoveryConfig default {{parent}}/discoveryConfigs/{{name}}
* ```
* ```sh
* $ pulumi import gcp:dataloss/preventionDiscoveryConfig:PreventionDiscoveryConfig default {{parent}}/{{name}}
* ```
* @property actions Actions to execute at the completion of scanning
* Structure is documented below.
* @property displayName Display Name (max 1000 Chars)
* @property inspectTemplates Detection logic for profile generation
* @property location Location to create the discovery config in.
* - - -
* @property orgConfig A nested object resource
* Structure is documented below.
* @property parent The parent of the discovery config in any of the following formats:
* * `projects/{{project}}/locations/{{location}}`
* * `organizations/{{organization_id}}/locations/{{location}}`
* @property status Required. A status for this configuration
* Possible values are: `RUNNING`, `PAUSED`.
* @property targets Target to match against for determining what to scan and how frequently
* Structure is documented below.
*/
public data class PreventionDiscoveryConfigArgs(
public val actions: Output>? = null,
public val displayName: Output? = null,
public val inspectTemplates: Output>? = null,
public val location: Output? = null,
public val orgConfig: Output? = null,
public val parent: Output? = null,
public val status: Output? = null,
public val targets: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataloss.PreventionDiscoveryConfigArgs =
com.pulumi.gcp.dataloss.PreventionDiscoveryConfigArgs.builder()
.actions(
actions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.displayName(displayName?.applyValue({ args0 -> args0 }))
.inspectTemplates(inspectTemplates?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.location(location?.applyValue({ args0 -> args0 }))
.orgConfig(orgConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.parent(parent?.applyValue({ args0 -> args0 }))
.status(status?.applyValue({ args0 -> args0 }))
.targets(
targets?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [PreventionDiscoveryConfigArgs].
*/
@PulumiTagMarker
public class PreventionDiscoveryConfigArgsBuilder internal constructor() {
private var actions: Output>? = null
private var displayName: Output? = null
private var inspectTemplates: Output>? = null
private var location: Output? = null
private var orgConfig: Output? = null
private var parent: Output? = null
private var status: Output? = null
private var targets: Output>? = null
/**
* @param value Actions to execute at the completion of scanning
* Structure is documented below.
*/
@JvmName("cmnlgktrtuhlwmit")
public suspend fun actions(`value`: Output>) {
this.actions = value
}
@JvmName("ftpfmcuiqganqdcw")
public suspend fun actions(vararg values: Output) {
this.actions = Output.all(values.asList())
}
/**
* @param values Actions to execute at the completion of scanning
* Structure is documented below.
*/
@JvmName("sgpscisuyhlrblms")
public suspend fun actions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy