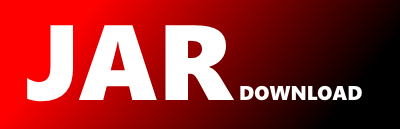
com.pulumi.gcp.dataloss.kotlin.inputs.PreventionDeidentifyTemplateDeidentifyConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataloss.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataloss.inputs.PreventionDeidentifyTemplateDeidentifyConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property imageTransformations Treat the dataset as an image and redact.
* Structure is documented below.
* @property infoTypeTransformations Treat the dataset as free-form text and apply the same free text transformation everywhere
* Structure is documented below.
* @property recordTransformations Treat the dataset as structured. Transformations can be applied to specific locations within structured datasets, such as transforming a column within a table.
* Structure is documented below.
*/
public data class PreventionDeidentifyTemplateDeidentifyConfigArgs(
public val imageTransformations: Output? = null,
public val infoTypeTransformations: Output? = null,
public val recordTransformations: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataloss.inputs.PreventionDeidentifyTemplateDeidentifyConfigArgs =
com.pulumi.gcp.dataloss.inputs.PreventionDeidentifyTemplateDeidentifyConfigArgs.builder()
.imageTransformations(
imageTransformations?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.infoTypeTransformations(
infoTypeTransformations?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.recordTransformations(
recordTransformations?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [PreventionDeidentifyTemplateDeidentifyConfigArgs].
*/
@PulumiTagMarker
public class PreventionDeidentifyTemplateDeidentifyConfigArgsBuilder internal constructor() {
private var imageTransformations:
Output? = null
private var infoTypeTransformations:
Output? = null
private var recordTransformations:
Output? = null
/**
* @param value Treat the dataset as an image and redact.
* Structure is documented below.
*/
@JvmName("hgrjmnqvshukaove")
public suspend fun imageTransformations(`value`: Output) {
this.imageTransformations = value
}
/**
* @param value Treat the dataset as free-form text and apply the same free text transformation everywhere
* Structure is documented below.
*/
@JvmName("sayjbnexpmseyinx")
public suspend fun infoTypeTransformations(`value`: Output) {
this.infoTypeTransformations = value
}
/**
* @param value Treat the dataset as structured. Transformations can be applied to specific locations within structured datasets, such as transforming a column within a table.
* Structure is documented below.
*/
@JvmName("xpoglcaokjbbmyqf")
public suspend fun recordTransformations(`value`: Output) {
this.recordTransformations = value
}
/**
* @param value Treat the dataset as an image and redact.
* Structure is documented below.
*/
@JvmName("pxgqfkorvbtnbkha")
public suspend fun imageTransformations(`value`: PreventionDeidentifyTemplateDeidentifyConfigImageTransformationsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.imageTransformations = mapped
}
/**
* @param argument Treat the dataset as an image and redact.
* Structure is documented below.
*/
@JvmName("hgmafctkxgqltkbj")
public suspend fun imageTransformations(argument: suspend PreventionDeidentifyTemplateDeidentifyConfigImageTransformationsArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionDeidentifyTemplateDeidentifyConfigImageTransformationsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.imageTransformations = mapped
}
/**
* @param value Treat the dataset as free-form text and apply the same free text transformation everywhere
* Structure is documented below.
*/
@JvmName("ahpyeadcfkcqixgx")
public suspend fun infoTypeTransformations(`value`: PreventionDeidentifyTemplateDeidentifyConfigInfoTypeTransformationsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.infoTypeTransformations = mapped
}
/**
* @param argument Treat the dataset as free-form text and apply the same free text transformation everywhere
* Structure is documented below.
*/
@JvmName("reiixjynebgnbhgr")
public suspend fun infoTypeTransformations(argument: suspend PreventionDeidentifyTemplateDeidentifyConfigInfoTypeTransformationsArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionDeidentifyTemplateDeidentifyConfigInfoTypeTransformationsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.infoTypeTransformations = mapped
}
/**
* @param value Treat the dataset as structured. Transformations can be applied to specific locations within structured datasets, such as transforming a column within a table.
* Structure is documented below.
*/
@JvmName("xfkphtnqfhtdflmh")
public suspend fun recordTransformations(`value`: PreventionDeidentifyTemplateDeidentifyConfigRecordTransformationsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recordTransformations = mapped
}
/**
* @param argument Treat the dataset as structured. Transformations can be applied to specific locations within structured datasets, such as transforming a column within a table.
* Structure is documented below.
*/
@JvmName("uwecehrldbbcbfbi")
public suspend fun recordTransformations(argument: suspend PreventionDeidentifyTemplateDeidentifyConfigRecordTransformationsArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionDeidentifyTemplateDeidentifyConfigRecordTransformationsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.recordTransformations = mapped
}
internal fun build(): PreventionDeidentifyTemplateDeidentifyConfigArgs =
PreventionDeidentifyTemplateDeidentifyConfigArgs(
imageTransformations = imageTransformations,
infoTypeTransformations = infoTypeTransformations,
recordTransformations = recordTransformations,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy