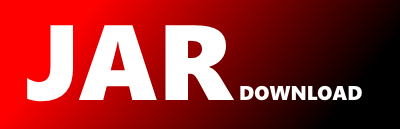
com.pulumi.gcp.dataloss.kotlin.inputs.PreventionDiscoveryConfigTargetBigQueryTargetConditionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataloss.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataloss.inputs.PreventionDiscoveryConfigTargetBigQueryTargetConditionsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property createdAfter A timestamp in RFC3339 UTC "Zulu" format with nanosecond resolution and upto nine fractional digits.
* @property orConditions At least one of the conditions must be true for a table to be scanned.
* Structure is documented below.
* @property typeCollection Restrict discovery to categories of table types. Currently view, materialized view, snapshot and non-biglake external tables are supported.
* Possible values are: `BIG_QUERY_COLLECTION_ALL_TYPES`, `BIG_QUERY_COLLECTION_ONLY_SUPPORTED_TYPES`.
* @property types Data profiles will only be generated for the database resource types specified in this field. If not specified, defaults to [DATABASE_RESOURCE_TYPE_ALL_SUPPORTED_TYPES].
* Each value may be one of: `DATABASE_RESOURCE_TYPE_ALL_SUPPORTED_TYPES`, `DATABASE_RESOURCE_TYPE_TABLE`.
*/
public data class PreventionDiscoveryConfigTargetBigQueryTargetConditionsArgs(
public val createdAfter: Output? = null,
public val orConditions: Output? = null,
public val typeCollection: Output? = null,
public val types: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataloss.inputs.PreventionDiscoveryConfigTargetBigQueryTargetConditionsArgs =
com.pulumi.gcp.dataloss.inputs.PreventionDiscoveryConfigTargetBigQueryTargetConditionsArgs.builder()
.createdAfter(createdAfter?.applyValue({ args0 -> args0 }))
.orConditions(orConditions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.typeCollection(typeCollection?.applyValue({ args0 -> args0 }))
.types(types?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [PreventionDiscoveryConfigTargetBigQueryTargetConditionsArgs].
*/
@PulumiTagMarker
public class PreventionDiscoveryConfigTargetBigQueryTargetConditionsArgsBuilder internal constructor() {
private var createdAfter: Output? = null
private var orConditions:
Output? = null
private var typeCollection: Output? = null
private var types: Output? =
null
/**
* @param value A timestamp in RFC3339 UTC "Zulu" format with nanosecond resolution and upto nine fractional digits.
*/
@JvmName("ljyyeaoeggjhjewb")
public suspend fun createdAfter(`value`: Output) {
this.createdAfter = value
}
/**
* @param value At least one of the conditions must be true for a table to be scanned.
* Structure is documented below.
*/
@JvmName("dcogonxwhskrgnlc")
public suspend fun orConditions(`value`: Output) {
this.orConditions = value
}
/**
* @param value Restrict discovery to categories of table types. Currently view, materialized view, snapshot and non-biglake external tables are supported.
* Possible values are: `BIG_QUERY_COLLECTION_ALL_TYPES`, `BIG_QUERY_COLLECTION_ONLY_SUPPORTED_TYPES`.
*/
@JvmName("ixjjavqlgluxymaw")
public suspend fun typeCollection(`value`: Output) {
this.typeCollection = value
}
/**
* @param value Data profiles will only be generated for the database resource types specified in this field. If not specified, defaults to [DATABASE_RESOURCE_TYPE_ALL_SUPPORTED_TYPES].
* Each value may be one of: `DATABASE_RESOURCE_TYPE_ALL_SUPPORTED_TYPES`, `DATABASE_RESOURCE_TYPE_TABLE`.
*/
@JvmName("shgrxxwpwurwabmt")
public suspend fun types(`value`: Output) {
this.types = value
}
/**
* @param value A timestamp in RFC3339 UTC "Zulu" format with nanosecond resolution and upto nine fractional digits.
*/
@JvmName("qgnbvaqnnrvjlsvu")
public suspend fun createdAfter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.createdAfter = mapped
}
/**
* @param value At least one of the conditions must be true for a table to be scanned.
* Structure is documented below.
*/
@JvmName("glifuhoqimioxeym")
public suspend fun orConditions(`value`: PreventionDiscoveryConfigTargetBigQueryTargetConditionsOrConditionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.orConditions = mapped
}
/**
* @param argument At least one of the conditions must be true for a table to be scanned.
* Structure is documented below.
*/
@JvmName("aauvbnvaapwvwice")
public suspend fun orConditions(argument: suspend PreventionDiscoveryConfigTargetBigQueryTargetConditionsOrConditionsArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionDiscoveryConfigTargetBigQueryTargetConditionsOrConditionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.orConditions = mapped
}
/**
* @param value Restrict discovery to categories of table types. Currently view, materialized view, snapshot and non-biglake external tables are supported.
* Possible values are: `BIG_QUERY_COLLECTION_ALL_TYPES`, `BIG_QUERY_COLLECTION_ONLY_SUPPORTED_TYPES`.
*/
@JvmName("lwmwitpdegpwngwc")
public suspend fun typeCollection(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.typeCollection = mapped
}
/**
* @param value Data profiles will only be generated for the database resource types specified in this field. If not specified, defaults to [DATABASE_RESOURCE_TYPE_ALL_SUPPORTED_TYPES].
* Each value may be one of: `DATABASE_RESOURCE_TYPE_ALL_SUPPORTED_TYPES`, `DATABASE_RESOURCE_TYPE_TABLE`.
*/
@JvmName("ebmvvnojjwvxkkpe")
public suspend fun types(`value`: PreventionDiscoveryConfigTargetBigQueryTargetConditionsTypesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.types = mapped
}
/**
* @param argument Data profiles will only be generated for the database resource types specified in this field. If not specified, defaults to [DATABASE_RESOURCE_TYPE_ALL_SUPPORTED_TYPES].
* Each value may be one of: `DATABASE_RESOURCE_TYPE_ALL_SUPPORTED_TYPES`, `DATABASE_RESOURCE_TYPE_TABLE`.
*/
@JvmName("qsoniaurrjvssulb")
public suspend fun types(argument: suspend PreventionDiscoveryConfigTargetBigQueryTargetConditionsTypesArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionDiscoveryConfigTargetBigQueryTargetConditionsTypesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.types = mapped
}
internal fun build(): PreventionDiscoveryConfigTargetBigQueryTargetConditionsArgs =
PreventionDiscoveryConfigTargetBigQueryTargetConditionsArgs(
createdAfter = createdAfter,
orConditions = orConditions,
typeCollection = typeCollection,
types = types,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy