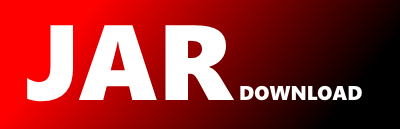
com.pulumi.gcp.dataloss.kotlin.inputs.PreventionJobTriggerInspectJobActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataloss.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobActionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property deidentify Create a de-identified copy of the requested table or files.
* Structure is documented below.
* @property jobNotificationEmails Sends an email when the job completes. The email goes to IAM project owners and technical Essential Contacts.
* @property pubSub Publish a message into a given Pub/Sub topic when the job completes.
* Structure is documented below.
* @property publishFindingsToCloudDataCatalog Publish findings of a DlpJob to Data Catalog.
* @property publishSummaryToCscc Publish the result summary of a DlpJob to the Cloud Security Command Center.
* @property publishToStackdriver Enable Stackdriver metric dlp.googleapis.com/findingCount.
* @property saveFindings If set, the detailed findings will be persisted to the specified OutputStorageConfig. Only a single instance of this action can be specified. Compatible with: Inspect, Risk
* Structure is documented below.
*/
public data class PreventionJobTriggerInspectJobActionArgs(
public val deidentify: Output? = null,
public val jobNotificationEmails: Output? = null,
public val pubSub: Output? = null,
public val publishFindingsToCloudDataCatalog: Output? = null,
public val publishSummaryToCscc: Output? = null,
public val publishToStackdriver: Output? = null,
public val saveFindings: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobActionArgs =
com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobActionArgs.builder()
.deidentify(deidentify?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.jobNotificationEmails(
jobNotificationEmails?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.pubSub(pubSub?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.publishFindingsToCloudDataCatalog(
publishFindingsToCloudDataCatalog?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.publishSummaryToCscc(
publishSummaryToCscc?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.publishToStackdriver(
publishToStackdriver?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.saveFindings(saveFindings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [PreventionJobTriggerInspectJobActionArgs].
*/
@PulumiTagMarker
public class PreventionJobTriggerInspectJobActionArgsBuilder internal constructor() {
private var deidentify: Output? = null
private var jobNotificationEmails:
Output? = null
private var pubSub: Output? = null
private var publishFindingsToCloudDataCatalog:
Output? = null
private var publishSummaryToCscc:
Output? = null
private var publishToStackdriver:
Output? = null
private var saveFindings: Output? = null
/**
* @param value Create a de-identified copy of the requested table or files.
* Structure is documented below.
*/
@JvmName("uhemtgdwdffauqga")
public suspend fun deidentify(`value`: Output) {
this.deidentify = value
}
/**
* @param value Sends an email when the job completes. The email goes to IAM project owners and technical Essential Contacts.
*/
@JvmName("nsnssbfijicuytia")
public suspend fun jobNotificationEmails(`value`: Output) {
this.jobNotificationEmails = value
}
/**
* @param value Publish a message into a given Pub/Sub topic when the job completes.
* Structure is documented below.
*/
@JvmName("pxouaccgwbpplgwe")
public suspend fun pubSub(`value`: Output) {
this.pubSub = value
}
/**
* @param value Publish findings of a DlpJob to Data Catalog.
*/
@JvmName("bsxfruquyiawyehy")
public suspend fun publishFindingsToCloudDataCatalog(`value`: Output) {
this.publishFindingsToCloudDataCatalog = value
}
/**
* @param value Publish the result summary of a DlpJob to the Cloud Security Command Center.
*/
@JvmName("iumfxdwaqquwshxx")
public suspend fun publishSummaryToCscc(`value`: Output) {
this.publishSummaryToCscc = value
}
/**
* @param value Enable Stackdriver metric dlp.googleapis.com/findingCount.
*/
@JvmName("uiejnxachekbsswx")
public suspend fun publishToStackdriver(`value`: Output) {
this.publishToStackdriver = value
}
/**
* @param value If set, the detailed findings will be persisted to the specified OutputStorageConfig. Only a single instance of this action can be specified. Compatible with: Inspect, Risk
* Structure is documented below.
*/
@JvmName("acyyvoixlejectrr")
public suspend fun saveFindings(`value`: Output) {
this.saveFindings = value
}
/**
* @param value Create a de-identified copy of the requested table or files.
* Structure is documented below.
*/
@JvmName("cecsjkhuxmbnvspu")
public suspend fun deidentify(`value`: PreventionJobTriggerInspectJobActionDeidentifyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deidentify = mapped
}
/**
* @param argument Create a de-identified copy of the requested table or files.
* Structure is documented below.
*/
@JvmName("qvevlqowcfactihh")
public suspend fun deidentify(argument: suspend PreventionJobTriggerInspectJobActionDeidentifyArgsBuilder.() -> Unit) {
val toBeMapped = PreventionJobTriggerInspectJobActionDeidentifyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.deidentify = mapped
}
/**
* @param value Sends an email when the job completes. The email goes to IAM project owners and technical Essential Contacts.
*/
@JvmName("jiowotrqkqhbbuqx")
public suspend fun jobNotificationEmails(`value`: PreventionJobTriggerInspectJobActionJobNotificationEmailsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.jobNotificationEmails = mapped
}
/**
* @param argument Sends an email when the job completes. The email goes to IAM project owners and technical Essential Contacts.
*/
@JvmName("aiuhirwvdfjwyqpj")
public suspend fun jobNotificationEmails(argument: suspend PreventionJobTriggerInspectJobActionJobNotificationEmailsArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobActionJobNotificationEmailsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.jobNotificationEmails = mapped
}
/**
* @param value Publish a message into a given Pub/Sub topic when the job completes.
* Structure is documented below.
*/
@JvmName("wvnhhhkxkkakvhws")
public suspend fun pubSub(`value`: PreventionJobTriggerInspectJobActionPubSubArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pubSub = mapped
}
/**
* @param argument Publish a message into a given Pub/Sub topic when the job completes.
* Structure is documented below.
*/
@JvmName("stsspwcganupnyla")
public suspend fun pubSub(argument: suspend PreventionJobTriggerInspectJobActionPubSubArgsBuilder.() -> Unit) {
val toBeMapped = PreventionJobTriggerInspectJobActionPubSubArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.pubSub = mapped
}
/**
* @param value Publish findings of a DlpJob to Data Catalog.
*/
@JvmName("wfqreprrocgogjxy")
public suspend fun publishFindingsToCloudDataCatalog(`value`: PreventionJobTriggerInspectJobActionPublishFindingsToCloudDataCatalogArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publishFindingsToCloudDataCatalog = mapped
}
/**
* @param argument Publish findings of a DlpJob to Data Catalog.
*/
@JvmName("jsxxawagjiaucawr")
public suspend fun publishFindingsToCloudDataCatalog(argument: suspend PreventionJobTriggerInspectJobActionPublishFindingsToCloudDataCatalogArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobActionPublishFindingsToCloudDataCatalogArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.publishFindingsToCloudDataCatalog = mapped
}
/**
* @param value Publish the result summary of a DlpJob to the Cloud Security Command Center.
*/
@JvmName("eocikiroxxpwwysc")
public suspend fun publishSummaryToCscc(`value`: PreventionJobTriggerInspectJobActionPublishSummaryToCsccArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publishSummaryToCscc = mapped
}
/**
* @param argument Publish the result summary of a DlpJob to the Cloud Security Command Center.
*/
@JvmName("xuhcnqfhgmnmtyaf")
public suspend fun publishSummaryToCscc(argument: suspend PreventionJobTriggerInspectJobActionPublishSummaryToCsccArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobActionPublishSummaryToCsccArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.publishSummaryToCscc = mapped
}
/**
* @param value Enable Stackdriver metric dlp.googleapis.com/findingCount.
*/
@JvmName("jfeyrdvmjlvqucxi")
public suspend fun publishToStackdriver(`value`: PreventionJobTriggerInspectJobActionPublishToStackdriverArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publishToStackdriver = mapped
}
/**
* @param argument Enable Stackdriver metric dlp.googleapis.com/findingCount.
*/
@JvmName("xknjinypqtphtmmp")
public suspend fun publishToStackdriver(argument: suspend PreventionJobTriggerInspectJobActionPublishToStackdriverArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobActionPublishToStackdriverArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.publishToStackdriver = mapped
}
/**
* @param value If set, the detailed findings will be persisted to the specified OutputStorageConfig. Only a single instance of this action can be specified. Compatible with: Inspect, Risk
* Structure is documented below.
*/
@JvmName("jthhcuedeemuwykl")
public suspend fun saveFindings(`value`: PreventionJobTriggerInspectJobActionSaveFindingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.saveFindings = mapped
}
/**
* @param argument If set, the detailed findings will be persisted to the specified OutputStorageConfig. Only a single instance of this action can be specified. Compatible with: Inspect, Risk
* Structure is documented below.
*/
@JvmName("gyihmdykvcyfiytw")
public suspend fun saveFindings(argument: suspend PreventionJobTriggerInspectJobActionSaveFindingsArgsBuilder.() -> Unit) {
val toBeMapped = PreventionJobTriggerInspectJobActionSaveFindingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.saveFindings = mapped
}
internal fun build(): PreventionJobTriggerInspectJobActionArgs =
PreventionJobTriggerInspectJobActionArgs(
deidentify = deidentify,
jobNotificationEmails = jobNotificationEmails,
pubSub = pubSub,
publishFindingsToCloudDataCatalog = publishFindingsToCloudDataCatalog,
publishSummaryToCscc = publishSummaryToCscc,
publishToStackdriver = publishToStackdriver,
saveFindings = saveFindings,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy