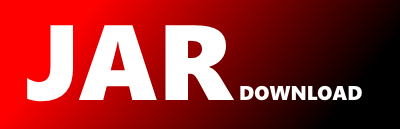
com.pulumi.gcp.dataloss.kotlin.inputs.PreventionJobTriggerInspectJobActionDeidentifyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataloss.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobActionDeidentifyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property cloudStorageOutput User settable Cloud Storage bucket and folders to store de-identified files.
* This field must be set for cloud storage deidentification.
* The output Cloud Storage bucket must be different from the input bucket.
* De-identified files will overwrite files in the output path.
* Form of: gs://bucket/folder/ or gs://bucket
* @property fileTypesToTransforms List of user-specified file type groups to transform. If specified, only the files with these filetypes will be transformed.
* If empty, all supported files will be transformed. Supported types may be automatically added over time.
* If a file type is set in this field that isn't supported by the Deidentify action then the job will fail and will not be successfully created/started.
* Each value may be one of: `IMAGE`, `TEXT_FILE`, `CSV`, `TSV`.
* @property transformationConfig User specified deidentify templates and configs for structured, unstructured, and image files.
* Structure is documented below.
* @property transformationDetailsStorageConfig Config for storing transformation details.
* Structure is documented below.
*/
public data class PreventionJobTriggerInspectJobActionDeidentifyArgs(
public val cloudStorageOutput: Output,
public val fileTypesToTransforms: Output>? = null,
public val transformationConfig: Output? = null,
public val transformationDetailsStorageConfig: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobActionDeidentifyArgs =
com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobActionDeidentifyArgs.builder()
.cloudStorageOutput(cloudStorageOutput.applyValue({ args0 -> args0 }))
.fileTypesToTransforms(fileTypesToTransforms?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.transformationConfig(
transformationConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.transformationDetailsStorageConfig(
transformationDetailsStorageConfig?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
).build()
}
/**
* Builder for [PreventionJobTriggerInspectJobActionDeidentifyArgs].
*/
@PulumiTagMarker
public class PreventionJobTriggerInspectJobActionDeidentifyArgsBuilder internal constructor() {
private var cloudStorageOutput: Output? = null
private var fileTypesToTransforms: Output>? = null
private var transformationConfig:
Output? = null
private var transformationDetailsStorageConfig:
Output? =
null
/**
* @param value User settable Cloud Storage bucket and folders to store de-identified files.
* This field must be set for cloud storage deidentification.
* The output Cloud Storage bucket must be different from the input bucket.
* De-identified files will overwrite files in the output path.
* Form of: gs://bucket/folder/ or gs://bucket
*/
@JvmName("ngvyvppconwnefhb")
public suspend fun cloudStorageOutput(`value`: Output) {
this.cloudStorageOutput = value
}
/**
* @param value List of user-specified file type groups to transform. If specified, only the files with these filetypes will be transformed.
* If empty, all supported files will be transformed. Supported types may be automatically added over time.
* If a file type is set in this field that isn't supported by the Deidentify action then the job will fail and will not be successfully created/started.
* Each value may be one of: `IMAGE`, `TEXT_FILE`, `CSV`, `TSV`.
*/
@JvmName("snabvbdjlognxtmc")
public suspend fun fileTypesToTransforms(`value`: Output>) {
this.fileTypesToTransforms = value
}
@JvmName("vqridylpemtrdmxw")
public suspend fun fileTypesToTransforms(vararg values: Output) {
this.fileTypesToTransforms = Output.all(values.asList())
}
/**
* @param values List of user-specified file type groups to transform. If specified, only the files with these filetypes will be transformed.
* If empty, all supported files will be transformed. Supported types may be automatically added over time.
* If a file type is set in this field that isn't supported by the Deidentify action then the job will fail and will not be successfully created/started.
* Each value may be one of: `IMAGE`, `TEXT_FILE`, `CSV`, `TSV`.
*/
@JvmName("qdfvbjmggliegmkb")
public suspend fun fileTypesToTransforms(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy