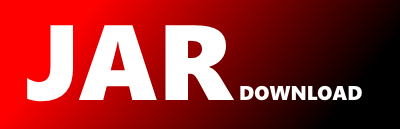
com.pulumi.gcp.dataloss.kotlin.inputs.PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataloss.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property hotwordRegex Regular expression pattern defining what qualifies as a hotword.
* Structure is documented below.
* @property likelihoodAdjustment Likelihood adjustment to apply to all matching findings.
* Structure is documented below.
* @property proximity Proximity of the finding within which the entire hotword must reside. The total length of the window cannot
* exceed 1000 characters. Note that the finding itself will be included in the window, so that hotwords may be
* used to match substrings of the finding itself. For example, the certainty of a phone number regex
* `(\d{3}) \d{3}-\d{4}` could be adjusted upwards if the area code is known to be the local area code of a company
* office using the hotword regex `(xxx)`, where `xxx` is the area code in question.
* Structure is documented below.
*/
public data class PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleArgs(
public val hotwordRegex: Output? =
null,
public val likelihoodAdjustment: Output? =
null,
public val proximity: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleArgs =
com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleArgs.builder()
.hotwordRegex(hotwordRegex?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.likelihoodAdjustment(
likelihoodAdjustment?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.proximity(proximity?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleArgs].
*/
@PulumiTagMarker
public class PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleArgsBuilder internal constructor() {
private var hotwordRegex:
Output? =
null
private var likelihoodAdjustment:
Output? =
null
private var proximity:
Output? = null
/**
* @param value Regular expression pattern defining what qualifies as a hotword.
* Structure is documented below.
*/
@JvmName("phkxghokbewxywbx")
public suspend fun hotwordRegex(`value`: Output) {
this.hotwordRegex = value
}
/**
* @param value Likelihood adjustment to apply to all matching findings.
* Structure is documented below.
*/
@JvmName("prammsauoxnnkvvc")
public suspend fun likelihoodAdjustment(`value`: Output) {
this.likelihoodAdjustment = value
}
/**
* @param value Proximity of the finding within which the entire hotword must reside. The total length of the window cannot
* exceed 1000 characters. Note that the finding itself will be included in the window, so that hotwords may be
* used to match substrings of the finding itself. For example, the certainty of a phone number regex
* `(\d{3}) \d{3}-\d{4}` could be adjusted upwards if the area code is known to be the local area code of a company
* office using the hotword regex `(xxx)`, where `xxx` is the area code in question.
* Structure is documented below.
*/
@JvmName("nvyhsogntcyihmfm")
public suspend fun proximity(`value`: Output) {
this.proximity = value
}
/**
* @param value Regular expression pattern defining what qualifies as a hotword.
* Structure is documented below.
*/
@JvmName("fevnasayvehlmwxr")
public suspend fun hotwordRegex(`value`: PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleHotwordRegexArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hotwordRegex = mapped
}
/**
* @param argument Regular expression pattern defining what qualifies as a hotword.
* Structure is documented below.
*/
@JvmName("nlskncaxferouhxi")
public suspend fun hotwordRegex(argument: suspend PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleHotwordRegexArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleHotwordRegexArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.hotwordRegex = mapped
}
/**
* @param value Likelihood adjustment to apply to all matching findings.
* Structure is documented below.
*/
@JvmName("wodrjimverbnpmft")
public suspend fun likelihoodAdjustment(`value`: PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleLikelihoodAdjustmentArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.likelihoodAdjustment = mapped
}
/**
* @param argument Likelihood adjustment to apply to all matching findings.
* Structure is documented below.
*/
@JvmName("vhalorghgvoropsg")
public suspend fun likelihoodAdjustment(argument: suspend PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleLikelihoodAdjustmentArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleLikelihoodAdjustmentArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.likelihoodAdjustment = mapped
}
/**
* @param value Proximity of the finding within which the entire hotword must reside. The total length of the window cannot
* exceed 1000 characters. Note that the finding itself will be included in the window, so that hotwords may be
* used to match substrings of the finding itself. For example, the certainty of a phone number regex
* `(\d{3}) \d{3}-\d{4}` could be adjusted upwards if the area code is known to be the local area code of a company
* office using the hotword regex `(xxx)`, where `xxx` is the area code in question.
* Structure is documented below.
*/
@JvmName("ybopyudbxjoikpds")
public suspend fun proximity(`value`: PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleProximityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.proximity = mapped
}
/**
* @param argument Proximity of the finding within which the entire hotword must reside. The total length of the window cannot
* exceed 1000 characters. Note that the finding itself will be included in the window, so that hotwords may be
* used to match substrings of the finding itself. For example, the certainty of a phone number regex
* `(\d{3}) \d{3}-\d{4}` could be adjusted upwards if the area code is known to be the local area code of a company
* office using the hotword regex `(xxx)`, where `xxx` is the area code in question.
* Structure is documented below.
*/
@JvmName("axijkyvqpgtwcgaf")
public suspend fun proximity(argument: suspend PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleProximityArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleProximityArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.proximity = mapped
}
internal fun build(): PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleArgs =
PreventionJobTriggerInspectJobInspectConfigRuleSetRuleHotwordRuleArgs(
hotwordRegex = hotwordRegex,
likelihoodAdjustment = likelihoodAdjustment,
proximity = proximity,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy