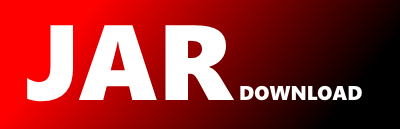
com.pulumi.gcp.dataloss.kotlin.inputs.PreventionJobTriggerInspectJobStorageConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataloss.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobStorageConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property bigQueryOptions Options defining BigQuery table and row identifiers.
* Structure is documented below.
* @property cloudStorageOptions Options defining a file or a set of files within a Google Cloud Storage bucket.
* Structure is documented below.
* @property datastoreOptions Options defining a data set within Google Cloud Datastore.
* Structure is documented below.
* @property hybridOptions Configuration to control jobs where the content being inspected is outside of Google Cloud Platform.
* Structure is documented below.
* @property timespanConfig Configuration of the timespan of the items to include in scanning
* Structure is documented below.
*/
public data class PreventionJobTriggerInspectJobStorageConfigArgs(
public val bigQueryOptions: Output? = null,
public val cloudStorageOptions: Output? = null,
public val datastoreOptions: Output? = null,
public val hybridOptions: Output? =
null,
public val timespanConfig: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobStorageConfigArgs =
com.pulumi.gcp.dataloss.inputs.PreventionJobTriggerInspectJobStorageConfigArgs.builder()
.bigQueryOptions(bigQueryOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.cloudStorageOptions(
cloudStorageOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.datastoreOptions(datastoreOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.hybridOptions(hybridOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.timespanConfig(
timespanConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [PreventionJobTriggerInspectJobStorageConfigArgs].
*/
@PulumiTagMarker
public class PreventionJobTriggerInspectJobStorageConfigArgsBuilder internal constructor() {
private var bigQueryOptions:
Output? = null
private var cloudStorageOptions:
Output? = null
private var datastoreOptions:
Output? = null
private var hybridOptions: Output? =
null
private var timespanConfig: Output? =
null
/**
* @param value Options defining BigQuery table and row identifiers.
* Structure is documented below.
*/
@JvmName("ihiltpuxeqplmbmi")
public suspend fun bigQueryOptions(`value`: Output) {
this.bigQueryOptions = value
}
/**
* @param value Options defining a file or a set of files within a Google Cloud Storage bucket.
* Structure is documented below.
*/
@JvmName("nxvgbptmshguqcue")
public suspend fun cloudStorageOptions(`value`: Output) {
this.cloudStorageOptions = value
}
/**
* @param value Options defining a data set within Google Cloud Datastore.
* Structure is documented below.
*/
@JvmName("qcmnnxwrorsdslby")
public suspend fun datastoreOptions(`value`: Output) {
this.datastoreOptions = value
}
/**
* @param value Configuration to control jobs where the content being inspected is outside of Google Cloud Platform.
* Structure is documented below.
*/
@JvmName("acvyqwwbhhfadsht")
public suspend fun hybridOptions(`value`: Output) {
this.hybridOptions = value
}
/**
* @param value Configuration of the timespan of the items to include in scanning
* Structure is documented below.
*/
@JvmName("auvesdbilufrmykw")
public suspend fun timespanConfig(`value`: Output) {
this.timespanConfig = value
}
/**
* @param value Options defining BigQuery table and row identifiers.
* Structure is documented below.
*/
@JvmName("djbylgaasxolmnqt")
public suspend fun bigQueryOptions(`value`: PreventionJobTriggerInspectJobStorageConfigBigQueryOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bigQueryOptions = mapped
}
/**
* @param argument Options defining BigQuery table and row identifiers.
* Structure is documented below.
*/
@JvmName("cucdbrvtmlahiftx")
public suspend fun bigQueryOptions(argument: suspend PreventionJobTriggerInspectJobStorageConfigBigQueryOptionsArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobStorageConfigBigQueryOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.bigQueryOptions = mapped
}
/**
* @param value Options defining a file or a set of files within a Google Cloud Storage bucket.
* Structure is documented below.
*/
@JvmName("wowyeipntarxrlvi")
public suspend fun cloudStorageOptions(`value`: PreventionJobTriggerInspectJobStorageConfigCloudStorageOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudStorageOptions = mapped
}
/**
* @param argument Options defining a file or a set of files within a Google Cloud Storage bucket.
* Structure is documented below.
*/
@JvmName("dpmiyiruxbfpvspy")
public suspend fun cloudStorageOptions(argument: suspend PreventionJobTriggerInspectJobStorageConfigCloudStorageOptionsArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobStorageConfigCloudStorageOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cloudStorageOptions = mapped
}
/**
* @param value Options defining a data set within Google Cloud Datastore.
* Structure is documented below.
*/
@JvmName("fifejwfpamsxyxnq")
public suspend fun datastoreOptions(`value`: PreventionJobTriggerInspectJobStorageConfigDatastoreOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.datastoreOptions = mapped
}
/**
* @param argument Options defining a data set within Google Cloud Datastore.
* Structure is documented below.
*/
@JvmName("bsdktlesrusqmdrc")
public suspend fun datastoreOptions(argument: suspend PreventionJobTriggerInspectJobStorageConfigDatastoreOptionsArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobStorageConfigDatastoreOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.datastoreOptions = mapped
}
/**
* @param value Configuration to control jobs where the content being inspected is outside of Google Cloud Platform.
* Structure is documented below.
*/
@JvmName("glqxjhlfusywuwyj")
public suspend fun hybridOptions(`value`: PreventionJobTriggerInspectJobStorageConfigHybridOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hybridOptions = mapped
}
/**
* @param argument Configuration to control jobs where the content being inspected is outside of Google Cloud Platform.
* Structure is documented below.
*/
@JvmName("jjcuintwtxqfqvhu")
public suspend fun hybridOptions(argument: suspend PreventionJobTriggerInspectJobStorageConfigHybridOptionsArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobStorageConfigHybridOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.hybridOptions = mapped
}
/**
* @param value Configuration of the timespan of the items to include in scanning
* Structure is documented below.
*/
@JvmName("cartywaucfdhvelw")
public suspend fun timespanConfig(`value`: PreventionJobTriggerInspectJobStorageConfigTimespanConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timespanConfig = mapped
}
/**
* @param argument Configuration of the timespan of the items to include in scanning
* Structure is documented below.
*/
@JvmName("kqjeyoiivhcoabwp")
public suspend fun timespanConfig(argument: suspend PreventionJobTriggerInspectJobStorageConfigTimespanConfigArgsBuilder.() -> Unit) {
val toBeMapped =
PreventionJobTriggerInspectJobStorageConfigTimespanConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.timespanConfig = mapped
}
internal fun build(): PreventionJobTriggerInspectJobStorageConfigArgs =
PreventionJobTriggerInspectJobStorageConfigArgs(
bigQueryOptions = bigQueryOptions,
cloudStorageOptions = cloudStorageOptions,
datastoreOptions = datastoreOptions,
hybridOptions = hybridOptions,
timespanConfig = timespanConfig,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy