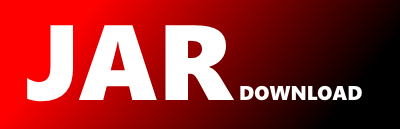
com.pulumi.gcp.dataplex.kotlin.inputs.DatascanDataQualitySpecRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataplex.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataplex.inputs.DatascanDataQualitySpecRuleArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property column The unnested column which this rule is evaluated against.
* @property description Description of the rule.
* The maximum length is 1,024 characters.
* @property dimension The dimension a rule belongs to. Results are also aggregated at the dimension level. Supported dimensions are ["COMPLETENESS", "ACCURACY", "CONSISTENCY", "VALIDITY", "UNIQUENESS", "INTEGRITY"]
* @property ignoreNull Rows with null values will automatically fail a rule, unless ignoreNull is true. In that case, such null rows are trivially considered passing. Only applicable to ColumnMap rules.
* @property name A mutable name for the rule.
* The name must contain only letters (a-z, A-Z), numbers (0-9), or hyphens (-).
* The maximum length is 63 characters.
* Must start with a letter.
* Must end with a number or a letter.
* @property nonNullExpectation ColumnMap rule which evaluates whether each column value is null.
* @property rangeExpectation ColumnMap rule which evaluates whether each column value lies between a specified range.
* Structure is documented below.
* @property regexExpectation ColumnMap rule which evaluates whether each column value matches a specified regex.
* Structure is documented below.
* @property rowConditionExpectation Table rule which evaluates whether each row passes the specified condition.
* Structure is documented below.
* @property setExpectation ColumnMap rule which evaluates whether each column value is contained by a specified set.
* Structure is documented below.
* @property statisticRangeExpectation ColumnAggregate rule which evaluates whether the column aggregate statistic lies between a specified range.
* Structure is documented below.
* @property tableConditionExpectation Table rule which evaluates whether the provided expression is true.
* Structure is documented below.
* @property threshold The minimum ratio of passing_rows / total_rows required to pass this rule, with a range of [0.0, 1.0]. 0 indicates default value (i.e. 1.0).
* @property uniquenessExpectation Row-level rule which evaluates whether each column value is unique.
*/
public data class DatascanDataQualitySpecRuleArgs(
public val column: Output? = null,
public val description: Output? = null,
public val dimension: Output,
public val ignoreNull: Output? = null,
public val name: Output? = null,
public val nonNullExpectation: Output? = null,
public val rangeExpectation: Output? = null,
public val regexExpectation: Output? = null,
public val rowConditionExpectation: Output? = null,
public val setExpectation: Output? = null,
public val statisticRangeExpectation: Output? = null,
public val tableConditionExpectation: Output? = null,
public val threshold: Output? = null,
public val uniquenessExpectation: Output? =
null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataplex.inputs.DatascanDataQualitySpecRuleArgs =
com.pulumi.gcp.dataplex.inputs.DatascanDataQualitySpecRuleArgs.builder()
.column(column?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.dimension(dimension.applyValue({ args0 -> args0 }))
.ignoreNull(ignoreNull?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.nonNullExpectation(
nonNullExpectation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.rangeExpectation(rangeExpectation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.regexExpectation(regexExpectation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.rowConditionExpectation(
rowConditionExpectation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.setExpectation(setExpectation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.statisticRangeExpectation(
statisticRangeExpectation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.tableConditionExpectation(
tableConditionExpectation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.threshold(threshold?.applyValue({ args0 -> args0 }))
.uniquenessExpectation(
uniquenessExpectation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DatascanDataQualitySpecRuleArgs].
*/
@PulumiTagMarker
public class DatascanDataQualitySpecRuleArgsBuilder internal constructor() {
private var column: Output? = null
private var description: Output? = null
private var dimension: Output? = null
private var ignoreNull: Output? = null
private var name: Output? = null
private var nonNullExpectation: Output? = null
private var rangeExpectation: Output? = null
private var regexExpectation: Output? = null
private var rowConditionExpectation:
Output? = null
private var setExpectation: Output? = null
private var statisticRangeExpectation:
Output? = null
private var tableConditionExpectation:
Output? = null
private var threshold: Output? = null
private var uniquenessExpectation: Output? =
null
/**
* @param value The unnested column which this rule is evaluated against.
*/
@JvmName("wwdiawqwoaqfhfdj")
public suspend fun column(`value`: Output) {
this.column = value
}
/**
* @param value Description of the rule.
* The maximum length is 1,024 characters.
*/
@JvmName("imgqhumsttuqkrve")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The dimension a rule belongs to. Results are also aggregated at the dimension level. Supported dimensions are ["COMPLETENESS", "ACCURACY", "CONSISTENCY", "VALIDITY", "UNIQUENESS", "INTEGRITY"]
*/
@JvmName("omltjwpwvbmhiati")
public suspend fun dimension(`value`: Output) {
this.dimension = value
}
/**
* @param value Rows with null values will automatically fail a rule, unless ignoreNull is true. In that case, such null rows are trivially considered passing. Only applicable to ColumnMap rules.
*/
@JvmName("bomhisgiwfcaxpip")
public suspend fun ignoreNull(`value`: Output) {
this.ignoreNull = value
}
/**
* @param value A mutable name for the rule.
* The name must contain only letters (a-z, A-Z), numbers (0-9), or hyphens (-).
* The maximum length is 63 characters.
* Must start with a letter.
* Must end with a number or a letter.
*/
@JvmName("qanfvpmcygvyixnt")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value ColumnMap rule which evaluates whether each column value is null.
*/
@JvmName("nhxsburggpracbvj")
public suspend fun nonNullExpectation(`value`: Output) {
this.nonNullExpectation = value
}
/**
* @param value ColumnMap rule which evaluates whether each column value lies between a specified range.
* Structure is documented below.
*/
@JvmName("ktjkbkqgctdtgajt")
public suspend fun rangeExpectation(`value`: Output) {
this.rangeExpectation = value
}
/**
* @param value ColumnMap rule which evaluates whether each column value matches a specified regex.
* Structure is documented below.
*/
@JvmName("aekqtnurbqvhygba")
public suspend fun regexExpectation(`value`: Output) {
this.regexExpectation = value
}
/**
* @param value Table rule which evaluates whether each row passes the specified condition.
* Structure is documented below.
*/
@JvmName("vfofvofhkgshimqy")
public suspend fun rowConditionExpectation(`value`: Output) {
this.rowConditionExpectation = value
}
/**
* @param value ColumnMap rule which evaluates whether each column value is contained by a specified set.
* Structure is documented below.
*/
@JvmName("htdtyphdilcsbrdb")
public suspend fun setExpectation(`value`: Output) {
this.setExpectation = value
}
/**
* @param value ColumnAggregate rule which evaluates whether the column aggregate statistic lies between a specified range.
* Structure is documented below.
*/
@JvmName("fkbwpvssakpqctkc")
public suspend fun statisticRangeExpectation(`value`: Output) {
this.statisticRangeExpectation = value
}
/**
* @param value Table rule which evaluates whether the provided expression is true.
* Structure is documented below.
*/
@JvmName("frefbourpnahokot")
public suspend fun tableConditionExpectation(`value`: Output) {
this.tableConditionExpectation = value
}
/**
* @param value The minimum ratio of passing_rows / total_rows required to pass this rule, with a range of [0.0, 1.0]. 0 indicates default value (i.e. 1.0).
*/
@JvmName("tidjgwlaxqecmbke")
public suspend fun threshold(`value`: Output) {
this.threshold = value
}
/**
* @param value Row-level rule which evaluates whether each column value is unique.
*/
@JvmName("dufrfacnwfkpkyie")
public suspend fun uniquenessExpectation(`value`: Output) {
this.uniquenessExpectation = value
}
/**
* @param value The unnested column which this rule is evaluated against.
*/
@JvmName("rjrppuapxymdebho")
public suspend fun column(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.column = mapped
}
/**
* @param value Description of the rule.
* The maximum length is 1,024 characters.
*/
@JvmName("yuwbyhtfbhebnlbx")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The dimension a rule belongs to. Results are also aggregated at the dimension level. Supported dimensions are ["COMPLETENESS", "ACCURACY", "CONSISTENCY", "VALIDITY", "UNIQUENESS", "INTEGRITY"]
*/
@JvmName("kenufiljdxyaadqg")
public suspend fun dimension(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.dimension = mapped
}
/**
* @param value Rows with null values will automatically fail a rule, unless ignoreNull is true. In that case, such null rows are trivially considered passing. Only applicable to ColumnMap rules.
*/
@JvmName("filqmaflcbilobkc")
public suspend fun ignoreNull(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ignoreNull = mapped
}
/**
* @param value A mutable name for the rule.
* The name must contain only letters (a-z, A-Z), numbers (0-9), or hyphens (-).
* The maximum length is 63 characters.
* Must start with a letter.
* Must end with a number or a letter.
*/
@JvmName("wttrxmujsaevjtrk")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value ColumnMap rule which evaluates whether each column value is null.
*/
@JvmName("puahwuqcqatsgcwd")
public suspend fun nonNullExpectation(`value`: DatascanDataQualitySpecRuleNonNullExpectationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nonNullExpectation = mapped
}
/**
* @param argument ColumnMap rule which evaluates whether each column value is null.
*/
@JvmName("fbmwonhvrjpmfgdt")
public suspend fun nonNullExpectation(argument: suspend DatascanDataQualitySpecRuleNonNullExpectationArgsBuilder.() -> Unit) {
val toBeMapped = DatascanDataQualitySpecRuleNonNullExpectationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.nonNullExpectation = mapped
}
/**
* @param value ColumnMap rule which evaluates whether each column value lies between a specified range.
* Structure is documented below.
*/
@JvmName("kmhxfsayejccemde")
public suspend fun rangeExpectation(`value`: DatascanDataQualitySpecRuleRangeExpectationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rangeExpectation = mapped
}
/**
* @param argument ColumnMap rule which evaluates whether each column value lies between a specified range.
* Structure is documented below.
*/
@JvmName("cgalndmoncgjeffp")
public suspend fun rangeExpectation(argument: suspend DatascanDataQualitySpecRuleRangeExpectationArgsBuilder.() -> Unit) {
val toBeMapped = DatascanDataQualitySpecRuleRangeExpectationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rangeExpectation = mapped
}
/**
* @param value ColumnMap rule which evaluates whether each column value matches a specified regex.
* Structure is documented below.
*/
@JvmName("xscjduudkpwsruxj")
public suspend fun regexExpectation(`value`: DatascanDataQualitySpecRuleRegexExpectationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.regexExpectation = mapped
}
/**
* @param argument ColumnMap rule which evaluates whether each column value matches a specified regex.
* Structure is documented below.
*/
@JvmName("uhokrgseqdupjtfj")
public suspend fun regexExpectation(argument: suspend DatascanDataQualitySpecRuleRegexExpectationArgsBuilder.() -> Unit) {
val toBeMapped = DatascanDataQualitySpecRuleRegexExpectationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.regexExpectation = mapped
}
/**
* @param value Table rule which evaluates whether each row passes the specified condition.
* Structure is documented below.
*/
@JvmName("kalktdyqldrnhrev")
public suspend fun rowConditionExpectation(`value`: DatascanDataQualitySpecRuleRowConditionExpectationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rowConditionExpectation = mapped
}
/**
* @param argument Table rule which evaluates whether each row passes the specified condition.
* Structure is documented below.
*/
@JvmName("eusipbylxtwixaun")
public suspend fun rowConditionExpectation(argument: suspend DatascanDataQualitySpecRuleRowConditionExpectationArgsBuilder.() -> Unit) {
val toBeMapped = DatascanDataQualitySpecRuleRowConditionExpectationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rowConditionExpectation = mapped
}
/**
* @param value ColumnMap rule which evaluates whether each column value is contained by a specified set.
* Structure is documented below.
*/
@JvmName("blgkwfqwlisjuada")
public suspend fun setExpectation(`value`: DatascanDataQualitySpecRuleSetExpectationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.setExpectation = mapped
}
/**
* @param argument ColumnMap rule which evaluates whether each column value is contained by a specified set.
* Structure is documented below.
*/
@JvmName("xelcqdtcqafvdbpn")
public suspend fun setExpectation(argument: suspend DatascanDataQualitySpecRuleSetExpectationArgsBuilder.() -> Unit) {
val toBeMapped = DatascanDataQualitySpecRuleSetExpectationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.setExpectation = mapped
}
/**
* @param value ColumnAggregate rule which evaluates whether the column aggregate statistic lies between a specified range.
* Structure is documented below.
*/
@JvmName("oguydfstsueltwkh")
public suspend fun statisticRangeExpectation(`value`: DatascanDataQualitySpecRuleStatisticRangeExpectationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.statisticRangeExpectation = mapped
}
/**
* @param argument ColumnAggregate rule which evaluates whether the column aggregate statistic lies between a specified range.
* Structure is documented below.
*/
@JvmName("scwyjopabanxmevh")
public suspend fun statisticRangeExpectation(argument: suspend DatascanDataQualitySpecRuleStatisticRangeExpectationArgsBuilder.() -> Unit) {
val toBeMapped = DatascanDataQualitySpecRuleStatisticRangeExpectationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.statisticRangeExpectation = mapped
}
/**
* @param value Table rule which evaluates whether the provided expression is true.
* Structure is documented below.
*/
@JvmName("jkbtlprsaxmyxiuj")
public suspend fun tableConditionExpectation(`value`: DatascanDataQualitySpecRuleTableConditionExpectationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tableConditionExpectation = mapped
}
/**
* @param argument Table rule which evaluates whether the provided expression is true.
* Structure is documented below.
*/
@JvmName("uvmbntivckgigwln")
public suspend fun tableConditionExpectation(argument: suspend DatascanDataQualitySpecRuleTableConditionExpectationArgsBuilder.() -> Unit) {
val toBeMapped = DatascanDataQualitySpecRuleTableConditionExpectationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.tableConditionExpectation = mapped
}
/**
* @param value The minimum ratio of passing_rows / total_rows required to pass this rule, with a range of [0.0, 1.0]. 0 indicates default value (i.e. 1.0).
*/
@JvmName("orwhwltcjwlicmdb")
public suspend fun threshold(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.threshold = mapped
}
/**
* @param value Row-level rule which evaluates whether each column value is unique.
*/
@JvmName("tepgtwqwriqmpcig")
public suspend fun uniquenessExpectation(`value`: DatascanDataQualitySpecRuleUniquenessExpectationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uniquenessExpectation = mapped
}
/**
* @param argument Row-level rule which evaluates whether each column value is unique.
*/
@JvmName("prblfhqubuuawgtw")
public suspend fun uniquenessExpectation(argument: suspend DatascanDataQualitySpecRuleUniquenessExpectationArgsBuilder.() -> Unit) {
val toBeMapped = DatascanDataQualitySpecRuleUniquenessExpectationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.uniquenessExpectation = mapped
}
internal fun build(): DatascanDataQualitySpecRuleArgs = DatascanDataQualitySpecRuleArgs(
column = column,
description = description,
dimension = dimension ?: throw PulumiNullFieldException("dimension"),
ignoreNull = ignoreNull,
name = name,
nonNullExpectation = nonNullExpectation,
rangeExpectation = rangeExpectation,
regexExpectation = regexExpectation,
rowConditionExpectation = rowConditionExpectation,
setExpectation = setExpectation,
statisticRangeExpectation = statisticRangeExpectation,
tableConditionExpectation = tableConditionExpectation,
threshold = threshold,
uniquenessExpectation = uniquenessExpectation,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy