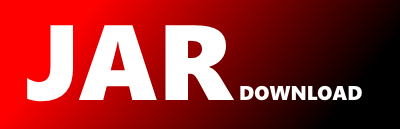
com.pulumi.gcp.dataplex.kotlin.inputs.DatascanDataQualitySpecRuleRangeExpectationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataplex.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataplex.inputs.DatascanDataQualitySpecRuleRangeExpectationArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property maxValue The maximum column value allowed for a row to pass this validation. At least one of minValue and maxValue need to be provided.
* @property minValue The minimum column value allowed for a row to pass this validation. At least one of minValue and maxValue need to be provided.
* @property strictMaxEnabled Whether each value needs to be strictly lesser than ('<') the maximum, or if equality is allowed.
* Only relevant if a maxValue has been defined. Default = false.
* @property strictMinEnabled Whether each value needs to be strictly greater than ('>') the minimum, or if equality is allowed.
* Only relevant if a minValue has been defined. Default = false.
*/
public data class DatascanDataQualitySpecRuleRangeExpectationArgs(
public val maxValue: Output? = null,
public val minValue: Output? = null,
public val strictMaxEnabled: Output? = null,
public val strictMinEnabled: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataplex.inputs.DatascanDataQualitySpecRuleRangeExpectationArgs =
com.pulumi.gcp.dataplex.inputs.DatascanDataQualitySpecRuleRangeExpectationArgs.builder()
.maxValue(maxValue?.applyValue({ args0 -> args0 }))
.minValue(minValue?.applyValue({ args0 -> args0 }))
.strictMaxEnabled(strictMaxEnabled?.applyValue({ args0 -> args0 }))
.strictMinEnabled(strictMinEnabled?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DatascanDataQualitySpecRuleRangeExpectationArgs].
*/
@PulumiTagMarker
public class DatascanDataQualitySpecRuleRangeExpectationArgsBuilder internal constructor() {
private var maxValue: Output? = null
private var minValue: Output? = null
private var strictMaxEnabled: Output? = null
private var strictMinEnabled: Output? = null
/**
* @param value The maximum column value allowed for a row to pass this validation. At least one of minValue and maxValue need to be provided.
*/
@JvmName("paipxoayqlhcuhqd")
public suspend fun maxValue(`value`: Output) {
this.maxValue = value
}
/**
* @param value The minimum column value allowed for a row to pass this validation. At least one of minValue and maxValue need to be provided.
*/
@JvmName("nqnsadwtkurxbnwk")
public suspend fun minValue(`value`: Output) {
this.minValue = value
}
/**
* @param value Whether each value needs to be strictly lesser than ('<') the maximum, or if equality is allowed.
* Only relevant if a maxValue has been defined. Default = false.
*/
@JvmName("bmcbwgsumabwctqs")
public suspend fun strictMaxEnabled(`value`: Output) {
this.strictMaxEnabled = value
}
/**
* @param value Whether each value needs to be strictly greater than ('>') the minimum, or if equality is allowed.
* Only relevant if a minValue has been defined. Default = false.
*/
@JvmName("bdvuccctvhonhbsj")
public suspend fun strictMinEnabled(`value`: Output) {
this.strictMinEnabled = value
}
/**
* @param value The maximum column value allowed for a row to pass this validation. At least one of minValue and maxValue need to be provided.
*/
@JvmName("vhbbnihlimsgvmce")
public suspend fun maxValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxValue = mapped
}
/**
* @param value The minimum column value allowed for a row to pass this validation. At least one of minValue and maxValue need to be provided.
*/
@JvmName("vyglpsrppifbmpnl")
public suspend fun minValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minValue = mapped
}
/**
* @param value Whether each value needs to be strictly lesser than ('<') the maximum, or if equality is allowed.
* Only relevant if a maxValue has been defined. Default = false.
*/
@JvmName("ornjxrwucdgesygs")
public suspend fun strictMaxEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.strictMaxEnabled = mapped
}
/**
* @param value Whether each value needs to be strictly greater than ('>') the minimum, or if equality is allowed.
* Only relevant if a minValue has been defined. Default = false.
*/
@JvmName("jcafdwwmcjwdanbu")
public suspend fun strictMinEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.strictMinEnabled = mapped
}
internal fun build(): DatascanDataQualitySpecRuleRangeExpectationArgs =
DatascanDataQualitySpecRuleRangeExpectationArgs(
maxValue = maxValue,
minValue = minValue,
strictMaxEnabled = strictMaxEnabled,
strictMinEnabled = strictMinEnabled,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy