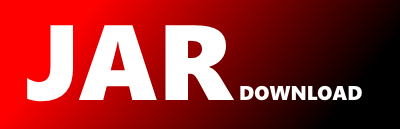
com.pulumi.gcp.dataplex.kotlin.outputs.DatascanDataQualitySpecRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataplex.kotlin.outputs
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
/**
*
* @property column The unnested column which this rule is evaluated against.
* @property description Description of the rule.
* The maximum length is 1,024 characters.
* @property dimension The dimension a rule belongs to. Results are also aggregated at the dimension level. Supported dimensions are ["COMPLETENESS", "ACCURACY", "CONSISTENCY", "VALIDITY", "UNIQUENESS", "INTEGRITY"]
* @property ignoreNull Rows with null values will automatically fail a rule, unless ignoreNull is true. In that case, such null rows are trivially considered passing. Only applicable to ColumnMap rules.
* @property name A mutable name for the rule.
* The name must contain only letters (a-z, A-Z), numbers (0-9), or hyphens (-).
* The maximum length is 63 characters.
* Must start with a letter.
* Must end with a number or a letter.
* @property nonNullExpectation ColumnMap rule which evaluates whether each column value is null.
* @property rangeExpectation ColumnMap rule which evaluates whether each column value lies between a specified range.
* Structure is documented below.
* @property regexExpectation ColumnMap rule which evaluates whether each column value matches a specified regex.
* Structure is documented below.
* @property rowConditionExpectation Table rule which evaluates whether each row passes the specified condition.
* Structure is documented below.
* @property setExpectation ColumnMap rule which evaluates whether each column value is contained by a specified set.
* Structure is documented below.
* @property statisticRangeExpectation ColumnAggregate rule which evaluates whether the column aggregate statistic lies between a specified range.
* Structure is documented below.
* @property tableConditionExpectation Table rule which evaluates whether the provided expression is true.
* Structure is documented below.
* @property threshold The minimum ratio of passing_rows / total_rows required to pass this rule, with a range of [0.0, 1.0]. 0 indicates default value (i.e. 1.0).
* @property uniquenessExpectation Row-level rule which evaluates whether each column value is unique.
*/
public data class DatascanDataQualitySpecRule(
public val column: String? = null,
public val description: String? = null,
public val dimension: String,
public val ignoreNull: Boolean? = null,
public val name: String? = null,
public val nonNullExpectation: DatascanDataQualitySpecRuleNonNullExpectation? = null,
public val rangeExpectation: DatascanDataQualitySpecRuleRangeExpectation? = null,
public val regexExpectation: DatascanDataQualitySpecRuleRegexExpectation? = null,
public val rowConditionExpectation: DatascanDataQualitySpecRuleRowConditionExpectation? = null,
public val setExpectation: DatascanDataQualitySpecRuleSetExpectation? = null,
public val statisticRangeExpectation: DatascanDataQualitySpecRuleStatisticRangeExpectation? =
null,
public val tableConditionExpectation: DatascanDataQualitySpecRuleTableConditionExpectation? =
null,
public val threshold: Double? = null,
public val uniquenessExpectation: DatascanDataQualitySpecRuleUniquenessExpectation? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.dataplex.outputs.DatascanDataQualitySpecRule): DatascanDataQualitySpecRule = DatascanDataQualitySpecRule(
column = javaType.column().map({ args0 -> args0 }).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
dimension = javaType.dimension(),
ignoreNull = javaType.ignoreNull().map({ args0 -> args0 }).orElse(null),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
nonNullExpectation = javaType.nonNullExpectation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataplex.kotlin.outputs.DatascanDataQualitySpecRuleNonNullExpectation.Companion.toKotlin(args0)
})
}).orElse(null),
rangeExpectation = javaType.rangeExpectation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataplex.kotlin.outputs.DatascanDataQualitySpecRuleRangeExpectation.Companion.toKotlin(args0)
})
}).orElse(null),
regexExpectation = javaType.regexExpectation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataplex.kotlin.outputs.DatascanDataQualitySpecRuleRegexExpectation.Companion.toKotlin(args0)
})
}).orElse(null),
rowConditionExpectation = javaType.rowConditionExpectation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataplex.kotlin.outputs.DatascanDataQualitySpecRuleRowConditionExpectation.Companion.toKotlin(args0)
})
}).orElse(null),
setExpectation = javaType.setExpectation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataplex.kotlin.outputs.DatascanDataQualitySpecRuleSetExpectation.Companion.toKotlin(args0)
})
}).orElse(null),
statisticRangeExpectation = javaType.statisticRangeExpectation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataplex.kotlin.outputs.DatascanDataQualitySpecRuleStatisticRangeExpectation.Companion.toKotlin(args0)
})
}).orElse(null),
tableConditionExpectation = javaType.tableConditionExpectation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataplex.kotlin.outputs.DatascanDataQualitySpecRuleTableConditionExpectation.Companion.toKotlin(args0)
})
}).orElse(null),
threshold = javaType.threshold().map({ args0 -> args0 }).orElse(null),
uniquenessExpectation = javaType.uniquenessExpectation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataplex.kotlin.outputs.DatascanDataQualitySpecRuleUniquenessExpectation.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy