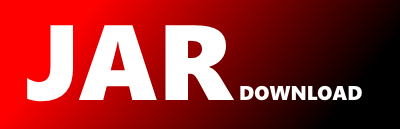
com.pulumi.gcp.dataproc.kotlin.MetastoreFederationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataproc.MetastoreFederationArgs.builder
import com.pulumi.gcp.dataproc.kotlin.inputs.MetastoreFederationBackendMetastoreArgs
import com.pulumi.gcp.dataproc.kotlin.inputs.MetastoreFederationBackendMetastoreArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A managed metastore federation.
* ## Example Usage
* ### Dataproc Metastore Federation Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultMetastoreService = new gcp.dataproc.MetastoreService("default", {
* serviceId: "",
* location: "us-central1",
* tier: "DEVELOPER",
* hiveMetastoreConfig: {
* version: "3.1.2",
* endpointProtocol: "GRPC",
* },
* });
* const _default = new gcp.dataproc.MetastoreFederation("default", {
* location: "us-central1",
* federationId: "",
* version: "3.1.2",
* backendMetastores: [{
* rank: "1",
* name: defaultMetastoreService.id,
* metastoreType: "DATAPROC_METASTORE",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_metastore_service = gcp.dataproc.MetastoreService("default",
* service_id="",
* location="us-central1",
* tier="DEVELOPER",
* hive_metastore_config=gcp.dataproc.MetastoreServiceHiveMetastoreConfigArgs(
* version="3.1.2",
* endpoint_protocol="GRPC",
* ))
* default = gcp.dataproc.MetastoreFederation("default",
* location="us-central1",
* federation_id="",
* version="3.1.2",
* backend_metastores=[gcp.dataproc.MetastoreFederationBackendMetastoreArgs(
* rank="1",
* name=default_metastore_service.id,
* metastore_type="DATAPROC_METASTORE",
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultMetastoreService = new Gcp.Dataproc.MetastoreService("default", new()
* {
* ServiceId = "",
* Location = "us-central1",
* Tier = "DEVELOPER",
* HiveMetastoreConfig = new Gcp.Dataproc.Inputs.MetastoreServiceHiveMetastoreConfigArgs
* {
* Version = "3.1.2",
* EndpointProtocol = "GRPC",
* },
* });
* var @default = new Gcp.Dataproc.MetastoreFederation("default", new()
* {
* Location = "us-central1",
* FederationId = "",
* Version = "3.1.2",
* BackendMetastores = new[]
* {
* new Gcp.Dataproc.Inputs.MetastoreFederationBackendMetastoreArgs
* {
* Rank = "1",
* Name = defaultMetastoreService.Id,
* MetastoreType = "DATAPROC_METASTORE",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/dataproc"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultMetastoreService, err := dataproc.NewMetastoreService(ctx, "default", &dataproc.MetastoreServiceArgs{
* ServiceId: pulumi.String(""),
* Location: pulumi.String("us-central1"),
* Tier: pulumi.String("DEVELOPER"),
* HiveMetastoreConfig: &dataproc.MetastoreServiceHiveMetastoreConfigArgs{
* Version: pulumi.String("3.1.2"),
* EndpointProtocol: pulumi.String("GRPC"),
* },
* })
* if err != nil {
* return err
* }
* _, err = dataproc.NewMetastoreFederation(ctx, "default", &dataproc.MetastoreFederationArgs{
* Location: pulumi.String("us-central1"),
* FederationId: pulumi.String(""),
* Version: pulumi.String("3.1.2"),
* BackendMetastores: dataproc.MetastoreFederationBackendMetastoreArray{
* &dataproc.MetastoreFederationBackendMetastoreArgs{
* Rank: pulumi.String("1"),
* Name: defaultMetastoreService.ID(),
* MetastoreType: pulumi.String("DATAPROC_METASTORE"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.dataproc.MetastoreService;
* import com.pulumi.gcp.dataproc.MetastoreServiceArgs;
* import com.pulumi.gcp.dataproc.inputs.MetastoreServiceHiveMetastoreConfigArgs;
* import com.pulumi.gcp.dataproc.MetastoreFederation;
* import com.pulumi.gcp.dataproc.MetastoreFederationArgs;
* import com.pulumi.gcp.dataproc.inputs.MetastoreFederationBackendMetastoreArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultMetastoreService = new MetastoreService("defaultMetastoreService", MetastoreServiceArgs.builder()
* .serviceId("")
* .location("us-central1")
* .tier("DEVELOPER")
* .hiveMetastoreConfig(MetastoreServiceHiveMetastoreConfigArgs.builder()
* .version("3.1.2")
* .endpointProtocol("GRPC")
* .build())
* .build());
* var default_ = new MetastoreFederation("default", MetastoreFederationArgs.builder()
* .location("us-central1")
* .federationId("")
* .version("3.1.2")
* .backendMetastores(MetastoreFederationBackendMetastoreArgs.builder()
* .rank("1")
* .name(defaultMetastoreService.id())
* .metastoreType("DATAPROC_METASTORE")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:dataproc:MetastoreFederation
* properties:
* location: us-central1
* federationId:
* version: 3.1.2
* backendMetastores:
* - rank: '1'
* name: ${defaultMetastoreService.id}
* metastoreType: DATAPROC_METASTORE
* defaultMetastoreService:
* type: gcp:dataproc:MetastoreService
* name: default
* properties:
* serviceId:
* location: us-central1
* tier: DEVELOPER
* hiveMetastoreConfig:
* version: 3.1.2
* endpointProtocol: GRPC
* ```
*
* ### Dataproc Metastore Federation Bigquery
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultMetastoreService = new gcp.dataproc.MetastoreService("default", {
* serviceId: "",
* location: "us-central1",
* tier: "DEVELOPER",
* hiveMetastoreConfig: {
* version: "3.1.2",
* endpointProtocol: "GRPC",
* },
* });
* const project = gcp.organizations.getProject({});
* const _default = new gcp.dataproc.MetastoreFederation("default", {
* location: "us-central1",
* federationId: "",
* version: "3.1.2",
* backendMetastores: [
* {
* rank: "2",
* name: project.then(project => project.id),
* metastoreType: "BIGQUERY",
* },
* {
* rank: "1",
* name: defaultMetastoreService.id,
* metastoreType: "DATAPROC_METASTORE",
* },
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_metastore_service = gcp.dataproc.MetastoreService("default",
* service_id="",
* location="us-central1",
* tier="DEVELOPER",
* hive_metastore_config=gcp.dataproc.MetastoreServiceHiveMetastoreConfigArgs(
* version="3.1.2",
* endpoint_protocol="GRPC",
* ))
* project = gcp.organizations.get_project()
* default = gcp.dataproc.MetastoreFederation("default",
* location="us-central1",
* federation_id="",
* version="3.1.2",
* backend_metastores=[
* gcp.dataproc.MetastoreFederationBackendMetastoreArgs(
* rank="2",
* name=project.id,
* metastore_type="BIGQUERY",
* ),
* gcp.dataproc.MetastoreFederationBackendMetastoreArgs(
* rank="1",
* name=default_metastore_service.id,
* metastore_type="DATAPROC_METASTORE",
* ),
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultMetastoreService = new Gcp.Dataproc.MetastoreService("default", new()
* {
* ServiceId = "",
* Location = "us-central1",
* Tier = "DEVELOPER",
* HiveMetastoreConfig = new Gcp.Dataproc.Inputs.MetastoreServiceHiveMetastoreConfigArgs
* {
* Version = "3.1.2",
* EndpointProtocol = "GRPC",
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var @default = new Gcp.Dataproc.MetastoreFederation("default", new()
* {
* Location = "us-central1",
* FederationId = "",
* Version = "3.1.2",
* BackendMetastores = new[]
* {
* new Gcp.Dataproc.Inputs.MetastoreFederationBackendMetastoreArgs
* {
* Rank = "2",
* Name = project.Apply(getProjectResult => getProjectResult.Id),
* MetastoreType = "BIGQUERY",
* },
* new Gcp.Dataproc.Inputs.MetastoreFederationBackendMetastoreArgs
* {
* Rank = "1",
* Name = defaultMetastoreService.Id,
* MetastoreType = "DATAPROC_METASTORE",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/dataproc"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultMetastoreService, err := dataproc.NewMetastoreService(ctx, "default", &dataproc.MetastoreServiceArgs{
* ServiceId: pulumi.String(""),
* Location: pulumi.String("us-central1"),
* Tier: pulumi.String("DEVELOPER"),
* HiveMetastoreConfig: &dataproc.MetastoreServiceHiveMetastoreConfigArgs{
* Version: pulumi.String("3.1.2"),
* EndpointProtocol: pulumi.String("GRPC"),
* },
* })
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = dataproc.NewMetastoreFederation(ctx, "default", &dataproc.MetastoreFederationArgs{
* Location: pulumi.String("us-central1"),
* FederationId: pulumi.String(""),
* Version: pulumi.String("3.1.2"),
* BackendMetastores: dataproc.MetastoreFederationBackendMetastoreArray{
* &dataproc.MetastoreFederationBackendMetastoreArgs{
* Rank: pulumi.String("2"),
* Name: pulumi.String(project.Id),
* MetastoreType: pulumi.String("BIGQUERY"),
* },
* &dataproc.MetastoreFederationBackendMetastoreArgs{
* Rank: pulumi.String("1"),
* Name: defaultMetastoreService.ID(),
* MetastoreType: pulumi.String("DATAPROC_METASTORE"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.dataproc.MetastoreService;
* import com.pulumi.gcp.dataproc.MetastoreServiceArgs;
* import com.pulumi.gcp.dataproc.inputs.MetastoreServiceHiveMetastoreConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.dataproc.MetastoreFederation;
* import com.pulumi.gcp.dataproc.MetastoreFederationArgs;
* import com.pulumi.gcp.dataproc.inputs.MetastoreFederationBackendMetastoreArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultMetastoreService = new MetastoreService("defaultMetastoreService", MetastoreServiceArgs.builder()
* .serviceId("")
* .location("us-central1")
* .tier("DEVELOPER")
* .hiveMetastoreConfig(MetastoreServiceHiveMetastoreConfigArgs.builder()
* .version("3.1.2")
* .endpointProtocol("GRPC")
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var default_ = new MetastoreFederation("default", MetastoreFederationArgs.builder()
* .location("us-central1")
* .federationId("")
* .version("3.1.2")
* .backendMetastores(
* MetastoreFederationBackendMetastoreArgs.builder()
* .rank("2")
* .name(project.applyValue(getProjectResult -> getProjectResult.id()))
* .metastoreType("BIGQUERY")
* .build(),
* MetastoreFederationBackendMetastoreArgs.builder()
* .rank("1")
* .name(defaultMetastoreService.id())
* .metastoreType("DATAPROC_METASTORE")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:dataproc:MetastoreFederation
* properties:
* location: us-central1
* federationId:
* version: 3.1.2
* backendMetastores:
* - rank: '2'
* name: ${project.id}
* metastoreType: BIGQUERY
* - rank: '1'
* name: ${defaultMetastoreService.id}
* metastoreType: DATAPROC_METASTORE
* defaultMetastoreService:
* type: gcp:dataproc:MetastoreService
* name: default
* properties:
* serviceId:
* location: us-central1
* tier: DEVELOPER
* hiveMetastoreConfig:
* version: 3.1.2
* endpointProtocol: GRPC
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* Federation can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/federations/{{federation_id}}`
* * `{{project}}/{{location}}/{{federation_id}}`
* * `{{location}}/{{federation_id}}`
* When using the `pulumi import` command, Federation can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:dataproc/metastoreFederation:MetastoreFederation default projects/{{project}}/locations/{{location}}/federations/{{federation_id}}
* ```
* ```sh
* $ pulumi import gcp:dataproc/metastoreFederation:MetastoreFederation default {{project}}/{{location}}/{{federation_id}}
* ```
* ```sh
* $ pulumi import gcp:dataproc/metastoreFederation:MetastoreFederation default {{location}}/{{federation_id}}
* ```
* @property backendMetastores A map from BackendMetastore rank to BackendMetastores from which the federation service serves metadata at query time. The map key represents the order in which BackendMetastores should be evaluated to resolve database names at query time and should be greater than or equal to zero. A BackendMetastore with a lower number will be evaluated before a BackendMetastore with a higher number.
* Structure is documented below.
* @property federationId The ID of the metastore federation. The id must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). Cannot begin or end with underscore or hyphen. Must consist of between
* 3 and 63 characters.
* @property labels User-defined labels for the metastore federation. **Note**: This field is non-authoritative, and will only manage the
* labels present in your configuration. Please refer to the field 'effective_labels' for all of the labels present on the
* resource.
* @property location The location where the metastore federation should reside.
* @property project
* @property version The Apache Hive metastore version of the federation. All backend metastore versions must be compatible with the federation version.
*/
public data class MetastoreFederationArgs(
public val backendMetastores: Output>? = null,
public val federationId: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy