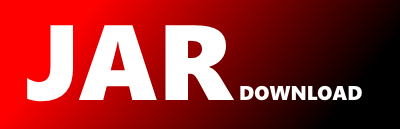
com.pulumi.gcp.dataproc.kotlin.inputs.AutoscalingPolicyBasicAlgorithmYarnConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataproc.inputs.AutoscalingPolicyBasicAlgorithmYarnConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property gracefulDecommissionTimeout Timeout for YARN graceful decommissioning of Node Managers. Specifies the
* duration to wait for jobs to complete before forcefully removing workers
* (and potentially interrupting jobs). Only applicable to downscaling operations.
* Bounds: [0s, 1d].
* @property scaleDownFactor Fraction of average pending memory in the last cooldown period for which to
* remove workers. A scale-down factor of 1 will result in scaling down so that there
* is no available memory remaining after the update (more aggressive scaling).
* A scale-down factor of 0 disables removing workers, which can be beneficial for
* autoscaling a single job.
* Bounds: [0.0, 1.0].
* @property scaleDownMinWorkerFraction Minimum scale-down threshold as a fraction of total cluster size before scaling occurs.
* For example, in a 20-worker cluster, a threshold of 0.1 means the autoscaler must
* recommend at least a 2 worker scale-down for the cluster to scale. A threshold of 0
* means the autoscaler will scale down on any recommended change.
* Bounds: [0.0, 1.0]. Default: 0.0.
* @property scaleUpFactor Fraction of average pending memory in the last cooldown period for which to
* add workers. A scale-up factor of 1.0 will result in scaling up so that there
* is no pending memory remaining after the update (more aggressive scaling).
* A scale-up factor closer to 0 will result in a smaller magnitude of scaling up
* (less aggressive scaling).
* Bounds: [0.0, 1.0].
* @property scaleUpMinWorkerFraction Minimum scale-up threshold as a fraction of total cluster size before scaling
* occurs. For example, in a 20-worker cluster, a threshold of 0.1 means the autoscaler
* must recommend at least a 2-worker scale-up for the cluster to scale. A threshold of
* 0 means the autoscaler will scale up on any recommended change.
* Bounds: [0.0, 1.0]. Default: 0.0.
*/
public data class AutoscalingPolicyBasicAlgorithmYarnConfigArgs(
public val gracefulDecommissionTimeout: Output,
public val scaleDownFactor: Output,
public val scaleDownMinWorkerFraction: Output? = null,
public val scaleUpFactor: Output,
public val scaleUpMinWorkerFraction: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataproc.inputs.AutoscalingPolicyBasicAlgorithmYarnConfigArgs =
com.pulumi.gcp.dataproc.inputs.AutoscalingPolicyBasicAlgorithmYarnConfigArgs.builder()
.gracefulDecommissionTimeout(gracefulDecommissionTimeout.applyValue({ args0 -> args0 }))
.scaleDownFactor(scaleDownFactor.applyValue({ args0 -> args0 }))
.scaleDownMinWorkerFraction(scaleDownMinWorkerFraction?.applyValue({ args0 -> args0 }))
.scaleUpFactor(scaleUpFactor.applyValue({ args0 -> args0 }))
.scaleUpMinWorkerFraction(scaleUpMinWorkerFraction?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AutoscalingPolicyBasicAlgorithmYarnConfigArgs].
*/
@PulumiTagMarker
public class AutoscalingPolicyBasicAlgorithmYarnConfigArgsBuilder internal constructor() {
private var gracefulDecommissionTimeout: Output? = null
private var scaleDownFactor: Output? = null
private var scaleDownMinWorkerFraction: Output? = null
private var scaleUpFactor: Output? = null
private var scaleUpMinWorkerFraction: Output? = null
/**
* @param value Timeout for YARN graceful decommissioning of Node Managers. Specifies the
* duration to wait for jobs to complete before forcefully removing workers
* (and potentially interrupting jobs). Only applicable to downscaling operations.
* Bounds: [0s, 1d].
*/
@JvmName("djrikvutfewlvcjv")
public suspend fun gracefulDecommissionTimeout(`value`: Output) {
this.gracefulDecommissionTimeout = value
}
/**
* @param value Fraction of average pending memory in the last cooldown period for which to
* remove workers. A scale-down factor of 1 will result in scaling down so that there
* is no available memory remaining after the update (more aggressive scaling).
* A scale-down factor of 0 disables removing workers, which can be beneficial for
* autoscaling a single job.
* Bounds: [0.0, 1.0].
*/
@JvmName("eglifgqagylbridc")
public suspend fun scaleDownFactor(`value`: Output) {
this.scaleDownFactor = value
}
/**
* @param value Minimum scale-down threshold as a fraction of total cluster size before scaling occurs.
* For example, in a 20-worker cluster, a threshold of 0.1 means the autoscaler must
* recommend at least a 2 worker scale-down for the cluster to scale. A threshold of 0
* means the autoscaler will scale down on any recommended change.
* Bounds: [0.0, 1.0]. Default: 0.0.
*/
@JvmName("spmtakdqxbibofrc")
public suspend fun scaleDownMinWorkerFraction(`value`: Output) {
this.scaleDownMinWorkerFraction = value
}
/**
* @param value Fraction of average pending memory in the last cooldown period for which to
* add workers. A scale-up factor of 1.0 will result in scaling up so that there
* is no pending memory remaining after the update (more aggressive scaling).
* A scale-up factor closer to 0 will result in a smaller magnitude of scaling up
* (less aggressive scaling).
* Bounds: [0.0, 1.0].
*/
@JvmName("pcstroioogpshyoh")
public suspend fun scaleUpFactor(`value`: Output) {
this.scaleUpFactor = value
}
/**
* @param value Minimum scale-up threshold as a fraction of total cluster size before scaling
* occurs. For example, in a 20-worker cluster, a threshold of 0.1 means the autoscaler
* must recommend at least a 2-worker scale-up for the cluster to scale. A threshold of
* 0 means the autoscaler will scale up on any recommended change.
* Bounds: [0.0, 1.0]. Default: 0.0.
*/
@JvmName("nqwcebmatabuxfik")
public suspend fun scaleUpMinWorkerFraction(`value`: Output) {
this.scaleUpMinWorkerFraction = value
}
/**
* @param value Timeout for YARN graceful decommissioning of Node Managers. Specifies the
* duration to wait for jobs to complete before forcefully removing workers
* (and potentially interrupting jobs). Only applicable to downscaling operations.
* Bounds: [0s, 1d].
*/
@JvmName("xybfbufvtcaxjjkq")
public suspend fun gracefulDecommissionTimeout(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.gracefulDecommissionTimeout = mapped
}
/**
* @param value Fraction of average pending memory in the last cooldown period for which to
* remove workers. A scale-down factor of 1 will result in scaling down so that there
* is no available memory remaining after the update (more aggressive scaling).
* A scale-down factor of 0 disables removing workers, which can be beneficial for
* autoscaling a single job.
* Bounds: [0.0, 1.0].
*/
@JvmName("vhvktcctqfwfsdbo")
public suspend fun scaleDownFactor(`value`: Double) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.scaleDownFactor = mapped
}
/**
* @param value Minimum scale-down threshold as a fraction of total cluster size before scaling occurs.
* For example, in a 20-worker cluster, a threshold of 0.1 means the autoscaler must
* recommend at least a 2 worker scale-down for the cluster to scale. A threshold of 0
* means the autoscaler will scale down on any recommended change.
* Bounds: [0.0, 1.0]. Default: 0.0.
*/
@JvmName("yigehnvhgxefvtlh")
public suspend fun scaleDownMinWorkerFraction(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scaleDownMinWorkerFraction = mapped
}
/**
* @param value Fraction of average pending memory in the last cooldown period for which to
* add workers. A scale-up factor of 1.0 will result in scaling up so that there
* is no pending memory remaining after the update (more aggressive scaling).
* A scale-up factor closer to 0 will result in a smaller magnitude of scaling up
* (less aggressive scaling).
* Bounds: [0.0, 1.0].
*/
@JvmName("hmegvygwoydlicdn")
public suspend fun scaleUpFactor(`value`: Double) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.scaleUpFactor = mapped
}
/**
* @param value Minimum scale-up threshold as a fraction of total cluster size before scaling
* occurs. For example, in a 20-worker cluster, a threshold of 0.1 means the autoscaler
* must recommend at least a 2-worker scale-up for the cluster to scale. A threshold of
* 0 means the autoscaler will scale up on any recommended change.
* Bounds: [0.0, 1.0]. Default: 0.0.
*/
@JvmName("rfdbykmvrhosvrie")
public suspend fun scaleUpMinWorkerFraction(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scaleUpMinWorkerFraction = mapped
}
internal fun build(): AutoscalingPolicyBasicAlgorithmYarnConfigArgs =
AutoscalingPolicyBasicAlgorithmYarnConfigArgs(
gracefulDecommissionTimeout = gracefulDecommissionTimeout ?: throw
PulumiNullFieldException("gracefulDecommissionTimeout"),
scaleDownFactor = scaleDownFactor ?: throw PulumiNullFieldException("scaleDownFactor"),
scaleDownMinWorkerFraction = scaleDownMinWorkerFraction,
scaleUpFactor = scaleUpFactor ?: throw PulumiNullFieldException("scaleUpFactor"),
scaleUpMinWorkerFraction = scaleUpMinWorkerFraction,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy