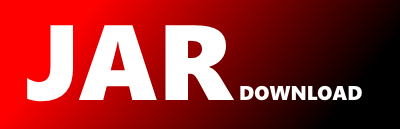
com.pulumi.gcp.dataproc.kotlin.inputs.ClusterClusterConfigSecurityConfigKerberosConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataproc.inputs.ClusterClusterConfigSecurityConfigKerberosConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property crossRealmTrustAdminServer The admin server (IP or hostname) for the
* remote trusted realm in a cross realm trust relationship.
* @property crossRealmTrustKdc The KDC (IP or hostname) for the
* remote trusted realm in a cross realm trust relationship.
* @property crossRealmTrustRealm The remote realm the Dataproc on-cluster KDC will
* trust, should the user enable cross realm trust.
* @property crossRealmTrustSharedPasswordUri The Cloud Storage URI of a KMS
* encrypted file containing the shared password between the on-cluster Kerberos realm
* and the remote trusted realm, in a cross realm trust relationship.
* @property enableKerberos Flag to indicate whether to Kerberize the cluster.
* @property kdcDbKeyUri The Cloud Storage URI of a KMS encrypted file containing
* the master key of the KDC database.
* @property keyPasswordUri The Cloud Storage URI of a KMS encrypted file containing
* the password to the user provided key. For the self-signed certificate, this password
* is generated by Dataproc.
* @property keystorePasswordUri The Cloud Storage URI of a KMS encrypted file containing
* the password to the user provided keystore. For the self-signed certificated, the password
* is generated by Dataproc.
* @property keystoreUri The Cloud Storage URI of the keystore file used for SSL encryption.
* If not provided, Dataproc will provide a self-signed certificate.
* @property kmsKeyUri The URI of the KMS key used to encrypt various sensitive files.
* @property realm The name of the on-cluster Kerberos realm. If not specified, the
* uppercased domain of hostnames will be the realm.
* @property rootPrincipalPasswordUri The Cloud Storage URI of a KMS encrypted file
* containing the root principal password.
* @property tgtLifetimeHours The lifetime of the ticket granting ticket, in hours.
* @property truststorePasswordUri The Cloud Storage URI of a KMS encrypted file
* containing the password to the user provided truststore. For the self-signed
* certificate, this password is generated by Dataproc.
* @property truststoreUri The Cloud Storage URI of the truststore file used for
* SSL encryption. If not provided, Dataproc will provide a self-signed certificate.
* - - -
*/
public data class ClusterClusterConfigSecurityConfigKerberosConfigArgs(
public val crossRealmTrustAdminServer: Output? = null,
public val crossRealmTrustKdc: Output? = null,
public val crossRealmTrustRealm: Output? = null,
public val crossRealmTrustSharedPasswordUri: Output? = null,
public val enableKerberos: Output? = null,
public val kdcDbKeyUri: Output? = null,
public val keyPasswordUri: Output? = null,
public val keystorePasswordUri: Output? = null,
public val keystoreUri: Output? = null,
public val kmsKeyUri: Output,
public val realm: Output? = null,
public val rootPrincipalPasswordUri: Output,
public val tgtLifetimeHours: Output? = null,
public val truststorePasswordUri: Output? = null,
public val truststoreUri: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataproc.inputs.ClusterClusterConfigSecurityConfigKerberosConfigArgs =
com.pulumi.gcp.dataproc.inputs.ClusterClusterConfigSecurityConfigKerberosConfigArgs.builder()
.crossRealmTrustAdminServer(crossRealmTrustAdminServer?.applyValue({ args0 -> args0 }))
.crossRealmTrustKdc(crossRealmTrustKdc?.applyValue({ args0 -> args0 }))
.crossRealmTrustRealm(crossRealmTrustRealm?.applyValue({ args0 -> args0 }))
.crossRealmTrustSharedPasswordUri(crossRealmTrustSharedPasswordUri?.applyValue({ args0 -> args0 }))
.enableKerberos(enableKerberos?.applyValue({ args0 -> args0 }))
.kdcDbKeyUri(kdcDbKeyUri?.applyValue({ args0 -> args0 }))
.keyPasswordUri(keyPasswordUri?.applyValue({ args0 -> args0 }))
.keystorePasswordUri(keystorePasswordUri?.applyValue({ args0 -> args0 }))
.keystoreUri(keystoreUri?.applyValue({ args0 -> args0 }))
.kmsKeyUri(kmsKeyUri.applyValue({ args0 -> args0 }))
.realm(realm?.applyValue({ args0 -> args0 }))
.rootPrincipalPasswordUri(rootPrincipalPasswordUri.applyValue({ args0 -> args0 }))
.tgtLifetimeHours(tgtLifetimeHours?.applyValue({ args0 -> args0 }))
.truststorePasswordUri(truststorePasswordUri?.applyValue({ args0 -> args0 }))
.truststoreUri(truststoreUri?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterClusterConfigSecurityConfigKerberosConfigArgs].
*/
@PulumiTagMarker
public class ClusterClusterConfigSecurityConfigKerberosConfigArgsBuilder internal constructor() {
private var crossRealmTrustAdminServer: Output? = null
private var crossRealmTrustKdc: Output? = null
private var crossRealmTrustRealm: Output? = null
private var crossRealmTrustSharedPasswordUri: Output? = null
private var enableKerberos: Output? = null
private var kdcDbKeyUri: Output? = null
private var keyPasswordUri: Output? = null
private var keystorePasswordUri: Output? = null
private var keystoreUri: Output? = null
private var kmsKeyUri: Output? = null
private var realm: Output? = null
private var rootPrincipalPasswordUri: Output? = null
private var tgtLifetimeHours: Output? = null
private var truststorePasswordUri: Output? = null
private var truststoreUri: Output? = null
/**
* @param value The admin server (IP or hostname) for the
* remote trusted realm in a cross realm trust relationship.
*/
@JvmName("mvenelkebrqjltdc")
public suspend fun crossRealmTrustAdminServer(`value`: Output) {
this.crossRealmTrustAdminServer = value
}
/**
* @param value The KDC (IP or hostname) for the
* remote trusted realm in a cross realm trust relationship.
*/
@JvmName("xkixbwcnqekivheu")
public suspend fun crossRealmTrustKdc(`value`: Output) {
this.crossRealmTrustKdc = value
}
/**
* @param value The remote realm the Dataproc on-cluster KDC will
* trust, should the user enable cross realm trust.
*/
@JvmName("flambbenmkfqvlmo")
public suspend fun crossRealmTrustRealm(`value`: Output) {
this.crossRealmTrustRealm = value
}
/**
* @param value The Cloud Storage URI of a KMS
* encrypted file containing the shared password between the on-cluster Kerberos realm
* and the remote trusted realm, in a cross realm trust relationship.
*/
@JvmName("uqduamerfqqbepun")
public suspend fun crossRealmTrustSharedPasswordUri(`value`: Output) {
this.crossRealmTrustSharedPasswordUri = value
}
/**
* @param value Flag to indicate whether to Kerberize the cluster.
*/
@JvmName("ovmvgomxlbpfkfqp")
public suspend fun enableKerberos(`value`: Output) {
this.enableKerberos = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing
* the master key of the KDC database.
*/
@JvmName("qlvoqykyfmnxwfig")
public suspend fun kdcDbKeyUri(`value`: Output) {
this.kdcDbKeyUri = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing
* the password to the user provided key. For the self-signed certificate, this password
* is generated by Dataproc.
*/
@JvmName("rhippghirnfckddg")
public suspend fun keyPasswordUri(`value`: Output) {
this.keyPasswordUri = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing
* the password to the user provided keystore. For the self-signed certificated, the password
* is generated by Dataproc.
*/
@JvmName("idljgyaarvuxsqcp")
public suspend fun keystorePasswordUri(`value`: Output) {
this.keystorePasswordUri = value
}
/**
* @param value The Cloud Storage URI of the keystore file used for SSL encryption.
* If not provided, Dataproc will provide a self-signed certificate.
*/
@JvmName("wdraisbbcravmyat")
public suspend fun keystoreUri(`value`: Output) {
this.keystoreUri = value
}
/**
* @param value The URI of the KMS key used to encrypt various sensitive files.
*/
@JvmName("mfggrldmdlhrjmir")
public suspend fun kmsKeyUri(`value`: Output) {
this.kmsKeyUri = value
}
/**
* @param value The name of the on-cluster Kerberos realm. If not specified, the
* uppercased domain of hostnames will be the realm.
*/
@JvmName("nvvxspertaaojtao")
public suspend fun realm(`value`: Output) {
this.realm = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file
* containing the root principal password.
*/
@JvmName("qlyphrknqjdjlvrc")
public suspend fun rootPrincipalPasswordUri(`value`: Output) {
this.rootPrincipalPasswordUri = value
}
/**
* @param value The lifetime of the ticket granting ticket, in hours.
*/
@JvmName("duhynlnohftxrndd")
public suspend fun tgtLifetimeHours(`value`: Output) {
this.tgtLifetimeHours = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file
* containing the password to the user provided truststore. For the self-signed
* certificate, this password is generated by Dataproc.
*/
@JvmName("dwprrqfxjykdlanb")
public suspend fun truststorePasswordUri(`value`: Output) {
this.truststorePasswordUri = value
}
/**
* @param value The Cloud Storage URI of the truststore file used for
* SSL encryption. If not provided, Dataproc will provide a self-signed certificate.
* - - -
*/
@JvmName("yjqyferxciaulcnu")
public suspend fun truststoreUri(`value`: Output) {
this.truststoreUri = value
}
/**
* @param value The admin server (IP or hostname) for the
* remote trusted realm in a cross realm trust relationship.
*/
@JvmName("leglaipwvmyujxpa")
public suspend fun crossRealmTrustAdminServer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossRealmTrustAdminServer = mapped
}
/**
* @param value The KDC (IP or hostname) for the
* remote trusted realm in a cross realm trust relationship.
*/
@JvmName("ddbtyknmrjraovrb")
public suspend fun crossRealmTrustKdc(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossRealmTrustKdc = mapped
}
/**
* @param value The remote realm the Dataproc on-cluster KDC will
* trust, should the user enable cross realm trust.
*/
@JvmName("ahjtyocxrwtpwhfj")
public suspend fun crossRealmTrustRealm(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossRealmTrustRealm = mapped
}
/**
* @param value The Cloud Storage URI of a KMS
* encrypted file containing the shared password between the on-cluster Kerberos realm
* and the remote trusted realm, in a cross realm trust relationship.
*/
@JvmName("jjedunudkdtfgqhn")
public suspend fun crossRealmTrustSharedPasswordUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossRealmTrustSharedPasswordUri = mapped
}
/**
* @param value Flag to indicate whether to Kerberize the cluster.
*/
@JvmName("lmuntugoiwbnhmtf")
public suspend fun enableKerberos(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableKerberos = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing
* the master key of the KDC database.
*/
@JvmName("pjtssigljkxpcxkm")
public suspend fun kdcDbKeyUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kdcDbKeyUri = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing
* the password to the user provided key. For the self-signed certificate, this password
* is generated by Dataproc.
*/
@JvmName("vfayvkrrrfyepbma")
public suspend fun keyPasswordUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyPasswordUri = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing
* the password to the user provided keystore. For the self-signed certificated, the password
* is generated by Dataproc.
*/
@JvmName("odjmfqfifxsigpdt")
public suspend fun keystorePasswordUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keystorePasswordUri = mapped
}
/**
* @param value The Cloud Storage URI of the keystore file used for SSL encryption.
* If not provided, Dataproc will provide a self-signed certificate.
*/
@JvmName("nthvpjsqigpmkwad")
public suspend fun keystoreUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keystoreUri = mapped
}
/**
* @param value The URI of the KMS key used to encrypt various sensitive files.
*/
@JvmName("jcxtcovvjmoldcwb")
public suspend fun kmsKeyUri(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kmsKeyUri = mapped
}
/**
* @param value The name of the on-cluster Kerberos realm. If not specified, the
* uppercased domain of hostnames will be the realm.
*/
@JvmName("iwogsscpodsakbxc")
public suspend fun realm(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.realm = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file
* containing the root principal password.
*/
@JvmName("lcyxtbvhyhfwaovs")
public suspend fun rootPrincipalPasswordUri(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.rootPrincipalPasswordUri = mapped
}
/**
* @param value The lifetime of the ticket granting ticket, in hours.
*/
@JvmName("faauokubuwgugpwi")
public suspend fun tgtLifetimeHours(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tgtLifetimeHours = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file
* containing the password to the user provided truststore. For the self-signed
* certificate, this password is generated by Dataproc.
*/
@JvmName("cvfdrdhooxinnyul")
public suspend fun truststorePasswordUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.truststorePasswordUri = mapped
}
/**
* @param value The Cloud Storage URI of the truststore file used for
* SSL encryption. If not provided, Dataproc will provide a self-signed certificate.
* - - -
*/
@JvmName("nnxauimbbbnwkqdt")
public suspend fun truststoreUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.truststoreUri = mapped
}
internal fun build(): ClusterClusterConfigSecurityConfigKerberosConfigArgs =
ClusterClusterConfigSecurityConfigKerberosConfigArgs(
crossRealmTrustAdminServer = crossRealmTrustAdminServer,
crossRealmTrustKdc = crossRealmTrustKdc,
crossRealmTrustRealm = crossRealmTrustRealm,
crossRealmTrustSharedPasswordUri = crossRealmTrustSharedPasswordUri,
enableKerberos = enableKerberos,
kdcDbKeyUri = kdcDbKeyUri,
keyPasswordUri = keyPasswordUri,
keystorePasswordUri = keystorePasswordUri,
keystoreUri = keystoreUri,
kmsKeyUri = kmsKeyUri ?: throw PulumiNullFieldException("kmsKeyUri"),
realm = realm,
rootPrincipalPasswordUri = rootPrincipalPasswordUri ?: throw
PulumiNullFieldException("rootPrincipalPasswordUri"),
tgtLifetimeHours = tgtLifetimeHours,
truststorePasswordUri = truststorePasswordUri,
truststoreUri = truststoreUri,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy