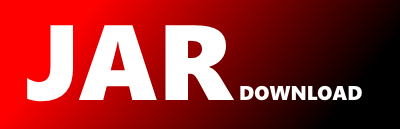
com.pulumi.gcp.dataproc.kotlin.inputs.ClusterVirtualClusterConfigKubernetesClusterConfigGkeClusterConfigNodePoolTargetNodePoolConfigConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataproc.inputs.ClusterVirtualClusterConfigKubernetesClusterConfigGkeClusterConfigNodePoolTargetNodePoolConfigConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property localSsdCount The number of local SSD disks to attach to the node,
* which is limited by the maximum number of disks allowable per zone.
* @property machineType The name of a Compute Engine machine type.
* @property minCpuPlatform Minimum CPU platform to be used by this instance.
* The instance may be scheduled on the specified or a newer CPU platform.
* Specify the friendly names of CPU platforms, such as "Intel Haswell" or "Intel Sandy Bridge".
* @property preemptible Whether the nodes are created as preemptible VM instances.
* Preemptible nodes cannot be used in a node pool with the CONTROLLER role or in the DEFAULT node pool if the
* CONTROLLER role is not assigned (the DEFAULT node pool will assume the CONTROLLER role).
* @property spot Spot flag for enabling Spot VM, which is a rebrand of the existing preemptible flag.
*/
public data class
ClusterVirtualClusterConfigKubernetesClusterConfigGkeClusterConfigNodePoolTargetNodePoolConfigConfigArgs(
public val localSsdCount: Output? = null,
public val machineType: Output? = null,
public val minCpuPlatform: Output? = null,
public val preemptible: Output? = null,
public val spot: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataproc.inputs.ClusterVirtualClusterConfigKubernetesClusterConfigGkeClusterConfigNodePoolTargetNodePoolConfigConfigArgs =
com.pulumi.gcp.dataproc.inputs.ClusterVirtualClusterConfigKubernetesClusterConfigGkeClusterConfigNodePoolTargetNodePoolConfigConfigArgs.builder()
.localSsdCount(localSsdCount?.applyValue({ args0 -> args0 }))
.machineType(machineType?.applyValue({ args0 -> args0 }))
.minCpuPlatform(minCpuPlatform?.applyValue({ args0 -> args0 }))
.preemptible(preemptible?.applyValue({ args0 -> args0 }))
.spot(spot?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterVirtualClusterConfigKubernetesClusterConfigGkeClusterConfigNodePoolTargetNodePoolConfigConfigArgs].
*/
@PulumiTagMarker
public class
ClusterVirtualClusterConfigKubernetesClusterConfigGkeClusterConfigNodePoolTargetNodePoolConfigConfigArgsBuilder
internal constructor() {
private var localSsdCount: Output? = null
private var machineType: Output? = null
private var minCpuPlatform: Output? = null
private var preemptible: Output? = null
private var spot: Output? = null
/**
* @param value The number of local SSD disks to attach to the node,
* which is limited by the maximum number of disks allowable per zone.
*/
@JvmName("jcrbbyrpsxewdixq")
public suspend fun localSsdCount(`value`: Output) {
this.localSsdCount = value
}
/**
* @param value The name of a Compute Engine machine type.
*/
@JvmName("geaqdwijhaxniilg")
public suspend fun machineType(`value`: Output) {
this.machineType = value
}
/**
* @param value Minimum CPU platform to be used by this instance.
* The instance may be scheduled on the specified or a newer CPU platform.
* Specify the friendly names of CPU platforms, such as "Intel Haswell" or "Intel Sandy Bridge".
*/
@JvmName("sfepkeyfjixkgaxo")
public suspend fun minCpuPlatform(`value`: Output) {
this.minCpuPlatform = value
}
/**
* @param value Whether the nodes are created as preemptible VM instances.
* Preemptible nodes cannot be used in a node pool with the CONTROLLER role or in the DEFAULT node pool if the
* CONTROLLER role is not assigned (the DEFAULT node pool will assume the CONTROLLER role).
*/
@JvmName("rwyajqejbpwkirub")
public suspend fun preemptible(`value`: Output) {
this.preemptible = value
}
/**
* @param value Spot flag for enabling Spot VM, which is a rebrand of the existing preemptible flag.
*/
@JvmName("kdljnmjcjefmnhwq")
public suspend fun spot(`value`: Output) {
this.spot = value
}
/**
* @param value The number of local SSD disks to attach to the node,
* which is limited by the maximum number of disks allowable per zone.
*/
@JvmName("oawkppjclcsfoucg")
public suspend fun localSsdCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.localSsdCount = mapped
}
/**
* @param value The name of a Compute Engine machine type.
*/
@JvmName("wguovhmxbqycrufo")
public suspend fun machineType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.machineType = mapped
}
/**
* @param value Minimum CPU platform to be used by this instance.
* The instance may be scheduled on the specified or a newer CPU platform.
* Specify the friendly names of CPU platforms, such as "Intel Haswell" or "Intel Sandy Bridge".
*/
@JvmName("opwfvbtwdijothul")
public suspend fun minCpuPlatform(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minCpuPlatform = mapped
}
/**
* @param value Whether the nodes are created as preemptible VM instances.
* Preemptible nodes cannot be used in a node pool with the CONTROLLER role or in the DEFAULT node pool if the
* CONTROLLER role is not assigned (the DEFAULT node pool will assume the CONTROLLER role).
*/
@JvmName("ycvgrldardwpmklw")
public suspend fun preemptible(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preemptible = mapped
}
/**
* @param value Spot flag for enabling Spot VM, which is a rebrand of the existing preemptible flag.
*/
@JvmName("bgtenlpqypqdyvve")
public suspend fun spot(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.spot = mapped
}
internal fun build(): ClusterVirtualClusterConfigKubernetesClusterConfigGkeClusterConfigNodePoolTargetNodePoolConfigConfigArgs =
ClusterVirtualClusterConfigKubernetesClusterConfigGkeClusterConfigNodePoolTargetNodePoolConfigConfigArgs(
localSsdCount = localSsdCount,
machineType = machineType,
minCpuPlatform = minCpuPlatform,
preemptible = preemptible,
spot = spot,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy