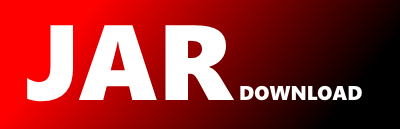
com.pulumi.gcp.dataproc.kotlin.inputs.WorkflowTemplatePlacementManagedClusterConfigSecurityConfigKerberosConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataproc.inputs.WorkflowTemplatePlacementManagedClusterConfigSecurityConfigKerberosConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property crossRealmTrustAdminServer The admin server (IP or hostname) for the remote trusted realm in a cross realm trust relationship.
* @property crossRealmTrustKdc The KDC (IP or hostname) for the remote trusted realm in a cross realm trust relationship.
* @property crossRealmTrustRealm The remote realm the Dataproc on-cluster KDC will trust, should the user enable cross realm trust.
* @property crossRealmTrustSharedPassword The Cloud Storage URI of a KMS encrypted file containing the shared password between the on-cluster Kerberos realm and the remote trusted realm, in a cross realm trust relationship.
* @property enableKerberos Flag to indicate whether to Kerberize the cluster (default: false). Set this field to true to enable Kerberos on a cluster.
* @property kdcDbKey The Cloud Storage URI of a KMS encrypted file containing the master key of the KDC database.
* @property keyPassword The Cloud Storage URI of a KMS encrypted file containing the password to the user provided key. For the self-signed certificate, this password is generated by Dataproc.
* @property keystore The Cloud Storage URI of the keystore file used for SSL encryption. If not provided, Dataproc will provide a self-signed certificate.
* @property keystorePassword The Cloud Storage URI of a KMS encrypted file containing the password to the user provided keystore. For the self-signed certificate, this password is generated by Dataproc.
* @property kmsKey The uri of the KMS key used to encrypt various sensitive files.
* @property realm The name of the on-cluster Kerberos realm. If not specified, the uppercased domain of hostnames will be the realm.
* @property rootPrincipalPassword The Cloud Storage URI of a KMS encrypted file containing the root principal password.
* @property tgtLifetimeHours The lifetime of the ticket granting ticket, in hours. If not specified, or user specifies 0, then default value 10 will be used.
* @property truststore The Cloud Storage URI of the truststore file used for SSL encryption. If not provided, Dataproc will provide a self-signed certificate.
* @property truststorePassword The Cloud Storage URI of a KMS encrypted file containing the password to the user provided truststore. For the self-signed certificate, this password is generated by Dataproc.
*/
public data class WorkflowTemplatePlacementManagedClusterConfigSecurityConfigKerberosConfigArgs(
public val crossRealmTrustAdminServer: Output? = null,
public val crossRealmTrustKdc: Output? = null,
public val crossRealmTrustRealm: Output? = null,
public val crossRealmTrustSharedPassword: Output? = null,
public val enableKerberos: Output? = null,
public val kdcDbKey: Output? = null,
public val keyPassword: Output? = null,
public val keystore: Output? = null,
public val keystorePassword: Output? = null,
public val kmsKey: Output? = null,
public val realm: Output? = null,
public val rootPrincipalPassword: Output? = null,
public val tgtLifetimeHours: Output? = null,
public val truststore: Output? = null,
public val truststorePassword: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dataproc.inputs.WorkflowTemplatePlacementManagedClusterConfigSecurityConfigKerberosConfigArgs =
com.pulumi.gcp.dataproc.inputs.WorkflowTemplatePlacementManagedClusterConfigSecurityConfigKerberosConfigArgs.builder()
.crossRealmTrustAdminServer(crossRealmTrustAdminServer?.applyValue({ args0 -> args0 }))
.crossRealmTrustKdc(crossRealmTrustKdc?.applyValue({ args0 -> args0 }))
.crossRealmTrustRealm(crossRealmTrustRealm?.applyValue({ args0 -> args0 }))
.crossRealmTrustSharedPassword(crossRealmTrustSharedPassword?.applyValue({ args0 -> args0 }))
.enableKerberos(enableKerberos?.applyValue({ args0 -> args0 }))
.kdcDbKey(kdcDbKey?.applyValue({ args0 -> args0 }))
.keyPassword(keyPassword?.applyValue({ args0 -> args0 }))
.keystore(keystore?.applyValue({ args0 -> args0 }))
.keystorePassword(keystorePassword?.applyValue({ args0 -> args0 }))
.kmsKey(kmsKey?.applyValue({ args0 -> args0 }))
.realm(realm?.applyValue({ args0 -> args0 }))
.rootPrincipalPassword(rootPrincipalPassword?.applyValue({ args0 -> args0 }))
.tgtLifetimeHours(tgtLifetimeHours?.applyValue({ args0 -> args0 }))
.truststore(truststore?.applyValue({ args0 -> args0 }))
.truststorePassword(truststorePassword?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WorkflowTemplatePlacementManagedClusterConfigSecurityConfigKerberosConfigArgs].
*/
@PulumiTagMarker
public class WorkflowTemplatePlacementManagedClusterConfigSecurityConfigKerberosConfigArgsBuilder
internal constructor() {
private var crossRealmTrustAdminServer: Output? = null
private var crossRealmTrustKdc: Output? = null
private var crossRealmTrustRealm: Output? = null
private var crossRealmTrustSharedPassword: Output? = null
private var enableKerberos: Output? = null
private var kdcDbKey: Output? = null
private var keyPassword: Output? = null
private var keystore: Output? = null
private var keystorePassword: Output? = null
private var kmsKey: Output? = null
private var realm: Output? = null
private var rootPrincipalPassword: Output? = null
private var tgtLifetimeHours: Output? = null
private var truststore: Output? = null
private var truststorePassword: Output? = null
/**
* @param value The admin server (IP or hostname) for the remote trusted realm in a cross realm trust relationship.
*/
@JvmName("pcvwmoyjgedqdlri")
public suspend fun crossRealmTrustAdminServer(`value`: Output) {
this.crossRealmTrustAdminServer = value
}
/**
* @param value The KDC (IP or hostname) for the remote trusted realm in a cross realm trust relationship.
*/
@JvmName("pgamkkyoedcnphmv")
public suspend fun crossRealmTrustKdc(`value`: Output) {
this.crossRealmTrustKdc = value
}
/**
* @param value The remote realm the Dataproc on-cluster KDC will trust, should the user enable cross realm trust.
*/
@JvmName("wxeiygjccahbuoqv")
public suspend fun crossRealmTrustRealm(`value`: Output) {
this.crossRealmTrustRealm = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the shared password between the on-cluster Kerberos realm and the remote trusted realm, in a cross realm trust relationship.
*/
@JvmName("qpqnsqgjpinvwngs")
public suspend fun crossRealmTrustSharedPassword(`value`: Output) {
this.crossRealmTrustSharedPassword = value
}
/**
* @param value Flag to indicate whether to Kerberize the cluster (default: false). Set this field to true to enable Kerberos on a cluster.
*/
@JvmName("jhngwwuwquihxnkt")
public suspend fun enableKerberos(`value`: Output) {
this.enableKerberos = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the master key of the KDC database.
*/
@JvmName("mbdylimrnucnohqo")
public suspend fun kdcDbKey(`value`: Output) {
this.kdcDbKey = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the password to the user provided key. For the self-signed certificate, this password is generated by Dataproc.
*/
@JvmName("bkuoptwjdbtvsrtq")
public suspend fun keyPassword(`value`: Output) {
this.keyPassword = value
}
/**
* @param value The Cloud Storage URI of the keystore file used for SSL encryption. If not provided, Dataproc will provide a self-signed certificate.
*/
@JvmName("etbuwicogwxduerp")
public suspend fun keystore(`value`: Output) {
this.keystore = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the password to the user provided keystore. For the self-signed certificate, this password is generated by Dataproc.
*/
@JvmName("abmjulpechgdwown")
public suspend fun keystorePassword(`value`: Output) {
this.keystorePassword = value
}
/**
* @param value The uri of the KMS key used to encrypt various sensitive files.
*/
@JvmName("kgduykjqevurgpfv")
public suspend fun kmsKey(`value`: Output) {
this.kmsKey = value
}
/**
* @param value The name of the on-cluster Kerberos realm. If not specified, the uppercased domain of hostnames will be the realm.
*/
@JvmName("kigpjytbmcgkyyjj")
public suspend fun realm(`value`: Output) {
this.realm = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the root principal password.
*/
@JvmName("bivlxahwpqhweqxq")
public suspend fun rootPrincipalPassword(`value`: Output) {
this.rootPrincipalPassword = value
}
/**
* @param value The lifetime of the ticket granting ticket, in hours. If not specified, or user specifies 0, then default value 10 will be used.
*/
@JvmName("qcqpigudgggsdqix")
public suspend fun tgtLifetimeHours(`value`: Output) {
this.tgtLifetimeHours = value
}
/**
* @param value The Cloud Storage URI of the truststore file used for SSL encryption. If not provided, Dataproc will provide a self-signed certificate.
*/
@JvmName("qioqfksegjsckpeg")
public suspend fun truststore(`value`: Output) {
this.truststore = value
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the password to the user provided truststore. For the self-signed certificate, this password is generated by Dataproc.
*/
@JvmName("rpleenjecctfejmr")
public suspend fun truststorePassword(`value`: Output) {
this.truststorePassword = value
}
/**
* @param value The admin server (IP or hostname) for the remote trusted realm in a cross realm trust relationship.
*/
@JvmName("nowbpgvhehxukjlb")
public suspend fun crossRealmTrustAdminServer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossRealmTrustAdminServer = mapped
}
/**
* @param value The KDC (IP or hostname) for the remote trusted realm in a cross realm trust relationship.
*/
@JvmName("lcvokelevvjgfbvr")
public suspend fun crossRealmTrustKdc(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossRealmTrustKdc = mapped
}
/**
* @param value The remote realm the Dataproc on-cluster KDC will trust, should the user enable cross realm trust.
*/
@JvmName("exowlrbmhkcflaxs")
public suspend fun crossRealmTrustRealm(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossRealmTrustRealm = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the shared password between the on-cluster Kerberos realm and the remote trusted realm, in a cross realm trust relationship.
*/
@JvmName("eyxtihhshyegwwoq")
public suspend fun crossRealmTrustSharedPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crossRealmTrustSharedPassword = mapped
}
/**
* @param value Flag to indicate whether to Kerberize the cluster (default: false). Set this field to true to enable Kerberos on a cluster.
*/
@JvmName("bhjiouutpjirtsdu")
public suspend fun enableKerberos(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableKerberos = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the master key of the KDC database.
*/
@JvmName("ngbjqtdrybymdbyx")
public suspend fun kdcDbKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kdcDbKey = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the password to the user provided key. For the self-signed certificate, this password is generated by Dataproc.
*/
@JvmName("qsfdkloijlfowgeq")
public suspend fun keyPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyPassword = mapped
}
/**
* @param value The Cloud Storage URI of the keystore file used for SSL encryption. If not provided, Dataproc will provide a self-signed certificate.
*/
@JvmName("pyakoeujqptdetxw")
public suspend fun keystore(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keystore = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the password to the user provided keystore. For the self-signed certificate, this password is generated by Dataproc.
*/
@JvmName("miedvbcnlelpsxfa")
public suspend fun keystorePassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keystorePassword = mapped
}
/**
* @param value The uri of the KMS key used to encrypt various sensitive files.
*/
@JvmName("vbjsihgbqukboaig")
public suspend fun kmsKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKey = mapped
}
/**
* @param value The name of the on-cluster Kerberos realm. If not specified, the uppercased domain of hostnames will be the realm.
*/
@JvmName("hlmxufjaqgsfvahl")
public suspend fun realm(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.realm = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the root principal password.
*/
@JvmName("hgwehkewnkiaunql")
public suspend fun rootPrincipalPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rootPrincipalPassword = mapped
}
/**
* @param value The lifetime of the ticket granting ticket, in hours. If not specified, or user specifies 0, then default value 10 will be used.
*/
@JvmName("tuugbpvuqwbeeknf")
public suspend fun tgtLifetimeHours(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tgtLifetimeHours = mapped
}
/**
* @param value The Cloud Storage URI of the truststore file used for SSL encryption. If not provided, Dataproc will provide a self-signed certificate.
*/
@JvmName("btpsubquijmsomlk")
public suspend fun truststore(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.truststore = mapped
}
/**
* @param value The Cloud Storage URI of a KMS encrypted file containing the password to the user provided truststore. For the self-signed certificate, this password is generated by Dataproc.
*/
@JvmName("nybfemgmcurlqsya")
public suspend fun truststorePassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.truststorePassword = mapped
}
internal fun build(): WorkflowTemplatePlacementManagedClusterConfigSecurityConfigKerberosConfigArgs =
WorkflowTemplatePlacementManagedClusterConfigSecurityConfigKerberosConfigArgs(
crossRealmTrustAdminServer = crossRealmTrustAdminServer,
crossRealmTrustKdc = crossRealmTrustKdc,
crossRealmTrustRealm = crossRealmTrustRealm,
crossRealmTrustSharedPassword = crossRealmTrustSharedPassword,
enableKerberos = enableKerberos,
kdcDbKey = kdcDbKey,
keyPassword = keyPassword,
keystore = keystore,
keystorePassword = keystorePassword,
kmsKey = kmsKey,
realm = realm,
rootPrincipalPassword = rootPrincipalPassword,
tgtLifetimeHours = tgtLifetimeHours,
truststore = truststore,
truststorePassword = truststorePassword,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy