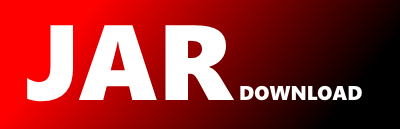
com.pulumi.gcp.dataproc.kotlin.inputs.WorkflowTemplatePlacementManagedClusterConfigSoftwareConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dataproc.inputs.WorkflowTemplatePlacementManagedClusterConfigSoftwareConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property imageVersion The version of software inside the cluster. It must be one of the supported [Dataproc Versions](https://cloud.google.com/dataproc/docs/concepts/versioning/dataproc-versions#supported_dataproc_versions), such as "1.2" (including a subminor version, such as "1.2.29"), or the ["preview" version](https://cloud.google.com/dataproc/docs/concepts/versioning/dataproc-versions#other_versions). If unspecified, it defaults to the latest Debian version.
* @property optionalComponents The set of components to activate on the cluster.
* @property properties The properties to set on daemon config files.
* Property keys are specified in `prefix:property` format, for example `core:hadoop.tmp.dir`. The following are supported prefixes and their mappings:
* * capacity-scheduler: `capacity-scheduler.xml`
* * core: `core-site.xml`
* * distcp: `distcp-default.xml`
* * hdfs: `hdfs-site.xml`
* * hive: `hive-site.xml`
* * mapred: `mapred-site.xml`
* * pig: `pig.properties`
* * spark: `spark-defaults.conf`
* * yarn: `yarn-site.xml`
* For more information, see [Cluster properties](https://cloud.google.com/dataproc/docs/concepts/cluster-properties).
*/
public data class WorkflowTemplatePlacementManagedClusterConfigSoftwareConfigArgs(
public val imageVersion: Output? = null,
public val optionalComponents: Output>? = null,
public val properties: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy