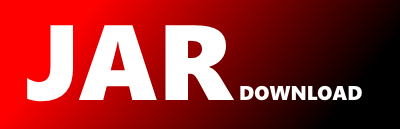
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property autoscalingConfig The autoscaling policy config associated with the cluster.
* Note that once set, if `autoscaling_config` is the only field set in `cluster_config`, it can
* only be removed by setting `policy_uri = ""`, rather than removing the whole block.
* Structure defined below.
* @property auxiliaryNodeGroups A Dataproc NodeGroup resource is a group of Dataproc cluster nodes that execute an assigned role.
* Structure defined below.
* @property bucket The name of the cloud storage bucket ultimately used to house the staging data
* for the cluster. If `staging_bucket` is specified, it will contain this value, otherwise
* it will be the auto generated name.
* @property dataprocMetricConfig The Compute Engine accelerator (GPU) configuration for these instances. Can be specified multiple times.
* Structure defined below.
* @property encryptionConfig The Customer managed encryption keys settings for the cluster.
* Structure defined below.
* @property endpointConfig The config settings for port access on the cluster.
* Structure defined below.
* @property gceClusterConfig Common config settings for resources of Google Compute Engine cluster
* instances, applicable to all instances in the cluster. Structure defined below.
* @property initializationActions Commands to execute on each node after config is completed.
* You can specify multiple versions of these. Structure defined below.
* @property lifecycleConfig The settings for auto deletion cluster schedule.
* Structure defined below.
* @property masterConfig The Google Compute Engine config settings for the master instances
* in a cluster. Structure defined below.
* @property metastoreConfig The config setting for metastore service with the cluster.
* Structure defined below.
* - - -
* @property preemptibleWorkerConfig The Google Compute Engine config settings for the additional
* instances in a cluster. Structure defined below.
* * **NOTE** : `preemptible_worker_config` is
* an alias for the api's [secondaryWorkerConfig](https://cloud.google.com/dataproc/docs/reference/rest/v1/ClusterConfig#InstanceGroupConfig). The name doesn't necessarily mean it is preemptible and is named as
* such for legacy/compatibility reasons.
* @property securityConfig Security related configuration. Structure defined below.
* @property softwareConfig The config settings for software inside the cluster.
* Structure defined below.
* @property stagingBucket The Cloud Storage staging bucket used to stage files,
* such as Hadoop jars, between client machines and the cluster.
* Note: If you don't explicitly specify a `staging_bucket`
* then GCP will auto create / assign one for you. However, you are not guaranteed
* an auto generated bucket which is solely dedicated to your cluster; it may be shared
* with other clusters in the same region/zone also choosing to use the auto generation
* option.
* @property tempBucket The Cloud Storage temp bucket used to store ephemeral cluster
* and jobs data, such as Spark and MapReduce history files.
* Note: If you don't explicitly specify a `temp_bucket` then GCP will auto create / assign one for you.
* @property workerConfig The Google Compute Engine config settings for the worker instances
* in a cluster. Structure defined below.
*/
public data class ClusterClusterConfig(
public val autoscalingConfig: ClusterClusterConfigAutoscalingConfig? = null,
public val auxiliaryNodeGroups: List? = null,
public val bucket: String? = null,
public val dataprocMetricConfig: ClusterClusterConfigDataprocMetricConfig? = null,
public val encryptionConfig: ClusterClusterConfigEncryptionConfig? = null,
public val endpointConfig: ClusterClusterConfigEndpointConfig? = null,
public val gceClusterConfig: ClusterClusterConfigGceClusterConfig? = null,
public val initializationActions: List? = null,
public val lifecycleConfig: ClusterClusterConfigLifecycleConfig? = null,
public val masterConfig: ClusterClusterConfigMasterConfig? = null,
public val metastoreConfig: ClusterClusterConfigMetastoreConfig? = null,
public val preemptibleWorkerConfig: ClusterClusterConfigPreemptibleWorkerConfig? = null,
public val securityConfig: ClusterClusterConfigSecurityConfig? = null,
public val softwareConfig: ClusterClusterConfigSoftwareConfig? = null,
public val stagingBucket: String? = null,
public val tempBucket: String? = null,
public val workerConfig: ClusterClusterConfigWorkerConfig? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.dataproc.outputs.ClusterClusterConfig): ClusterClusterConfig = ClusterClusterConfig(
autoscalingConfig = javaType.autoscalingConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigAutoscalingConfig.Companion.toKotlin(args0)
})
}).orElse(null),
auxiliaryNodeGroups = javaType.auxiliaryNodeGroups().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigAuxiliaryNodeGroup.Companion.toKotlin(args0)
})
}),
bucket = javaType.bucket().map({ args0 -> args0 }).orElse(null),
dataprocMetricConfig = javaType.dataprocMetricConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigDataprocMetricConfig.Companion.toKotlin(args0)
})
}).orElse(null),
encryptionConfig = javaType.encryptionConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigEncryptionConfig.Companion.toKotlin(args0)
})
}).orElse(null),
endpointConfig = javaType.endpointConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigEndpointConfig.Companion.toKotlin(args0)
})
}).orElse(null),
gceClusterConfig = javaType.gceClusterConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigGceClusterConfig.Companion.toKotlin(args0)
})
}).orElse(null),
initializationActions = javaType.initializationActions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigInitializationAction.Companion.toKotlin(args0)
})
}),
lifecycleConfig = javaType.lifecycleConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigLifecycleConfig.Companion.toKotlin(args0)
})
}).orElse(null),
masterConfig = javaType.masterConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigMasterConfig.Companion.toKotlin(args0)
})
}).orElse(null),
metastoreConfig = javaType.metastoreConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigMetastoreConfig.Companion.toKotlin(args0)
})
}).orElse(null),
preemptibleWorkerConfig = javaType.preemptibleWorkerConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigPreemptibleWorkerConfig.Companion.toKotlin(args0)
})
}).orElse(null),
securityConfig = javaType.securityConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigSecurityConfig.Companion.toKotlin(args0)
})
}).orElse(null),
softwareConfig = javaType.softwareConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigSoftwareConfig.Companion.toKotlin(args0)
})
}).orElse(null),
stagingBucket = javaType.stagingBucket().map({ args0 -> args0 }).orElse(null),
tempBucket = javaType.tempBucket().map({ args0 -> args0 }).orElse(null),
workerConfig = javaType.workerConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigWorkerConfig.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy