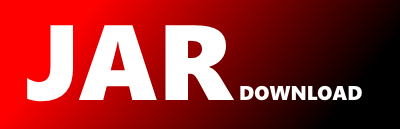
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigGceClusterConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property internalIpOnly By default, clusters are not restricted to internal IP addresses,
* and will have ephemeral external IP addresses assigned to each instance. If set to true, all
* instances in the cluster will only have internal IP addresses. Note: Private Google Access
* (also known as `privateIpGoogleAccess`) must be enabled on the subnetwork that the cluster
* will be launched in.
* @property metadata A map of the Compute Engine metadata entries to add to all instances
* (see [Project and instance metadata](https://cloud.google.com/compute/docs/storing-retrieving-metadata#project_and_instance_metadata)).
* @property network The name or self_link of the Google Compute Engine
* network to the cluster will be part of. Conflicts with `subnetwork`.
* If neither is specified, this defaults to the "default" network.
* @property nodeGroupAffinity Node Group Affinity for sole-tenant clusters.
* @property reservationAffinity Reservation Affinity for consuming zonal reservation.
* @property serviceAccount The service account to be used by the Node VMs.
* If not specified, the "default" service account is used.
* @property serviceAccountScopes The set of Google API scopes
* to be made available on all of the node VMs under the `service_account`
* specified. Both OAuth2 URLs and gcloud
* short names are supported. To allow full access to all Cloud APIs, use the
* `cloud-platform` scope. See a complete list of scopes [here](https://cloud.google.com/sdk/gcloud/reference/alpha/compute/instances/set-scopes#--scopes).
* @property shieldedInstanceConfig Shielded Instance Config for clusters using [Compute Engine Shielded VMs](https://cloud.google.com/security/shielded-cloud/shielded-vm).
* - - -
* @property subnetwork The name or self_link of the Google Compute Engine
* subnetwork the cluster will be part of. Conflicts with `network`.
* @property tags The list of instance tags applied to instances in the cluster.
* Tags are used to identify valid sources or targets for network firewalls.
* @property zone The GCP zone where your data is stored and used (i.e. where
* the master and the worker nodes will be created in). If `region` is set to 'global' (default)
* then `zone` is mandatory, otherwise GCP is able to make use of [Auto Zone Placement](https://cloud.google.com/dataproc/docs/concepts/auto-zone)
* to determine this automatically for you.
* Note: This setting additionally determines and restricts
* which computing resources are available for use with other configs such as
* `cluster_config.master_config.machine_type` and `cluster_config.worker_config.machine_type`.
*/
public data class ClusterClusterConfigGceClusterConfig(
public val internalIpOnly: Boolean? = null,
public val metadata: Map? = null,
public val network: String? = null,
public val nodeGroupAffinity: ClusterClusterConfigGceClusterConfigNodeGroupAffinity? = null,
public val reservationAffinity: ClusterClusterConfigGceClusterConfigReservationAffinity? = null,
public val serviceAccount: String? = null,
public val serviceAccountScopes: List? = null,
public val shieldedInstanceConfig: ClusterClusterConfigGceClusterConfigShieldedInstanceConfig? =
null,
public val subnetwork: String? = null,
public val tags: List? = null,
public val zone: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.dataproc.outputs.ClusterClusterConfigGceClusterConfig): ClusterClusterConfigGceClusterConfig = ClusterClusterConfigGceClusterConfig(
internalIpOnly = javaType.internalIpOnly().map({ args0 -> args0 }).orElse(null),
metadata = javaType.metadata().map({ args0 -> args0.key.to(args0.value) }).toMap(),
network = javaType.network().map({ args0 -> args0 }).orElse(null),
nodeGroupAffinity = javaType.nodeGroupAffinity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigGceClusterConfigNodeGroupAffinity.Companion.toKotlin(args0)
})
}).orElse(null),
reservationAffinity = javaType.reservationAffinity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigGceClusterConfigReservationAffinity.Companion.toKotlin(args0)
})
}).orElse(null),
serviceAccount = javaType.serviceAccount().map({ args0 -> args0 }).orElse(null),
serviceAccountScopes = javaType.serviceAccountScopes().map({ args0 -> args0 }),
shieldedInstanceConfig = javaType.shieldedInstanceConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.ClusterClusterConfigGceClusterConfigShieldedInstanceConfig.Companion.toKotlin(args0)
})
}).orElse(null),
subnetwork = javaType.subnetwork().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 -> args0 }),
zone = javaType.zone().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy