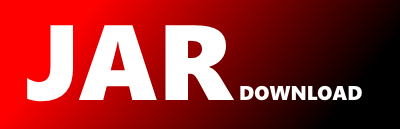
com.pulumi.gcp.dataproc.kotlin.outputs.GetMetastoreServiceResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getMetastoreService.
* @property artifactGcsUri
* @property databaseType
* @property effectiveLabels
* @property encryptionConfigs
* @property endpointUri
* @property hiveMetastoreConfigs
* @property id The provider-assigned unique ID for this managed resource.
* @property labels
* @property location
* @property maintenanceWindows
* @property metadataIntegrations
* @property name
* @property network
* @property networkConfigs
* @property port
* @property project
* @property pulumiLabels
* @property releaseChannel
* @property scalingConfigs
* @property scheduledBackups
* @property serviceId
* @property state
* @property stateMessage
* @property telemetryConfigs
* @property tier
* @property uid
*/
public data class GetMetastoreServiceResult(
public val artifactGcsUri: String,
public val databaseType: String,
public val effectiveLabels: Map,
public val encryptionConfigs: List,
public val endpointUri: String,
public val hiveMetastoreConfigs: List,
public val id: String,
public val labels: Map,
public val location: String,
public val maintenanceWindows: List,
public val metadataIntegrations: List,
public val name: String,
public val network: String,
public val networkConfigs: List,
public val port: Int,
public val project: String? = null,
public val pulumiLabels: Map,
public val releaseChannel: String,
public val scalingConfigs: List,
public val scheduledBackups: List,
public val serviceId: String,
public val state: String,
public val stateMessage: String,
public val telemetryConfigs: List,
public val tier: String,
public val uid: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.dataproc.outputs.GetMetastoreServiceResult): GetMetastoreServiceResult = GetMetastoreServiceResult(
artifactGcsUri = javaType.artifactGcsUri(),
databaseType = javaType.databaseType(),
effectiveLabels = javaType.effectiveLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
encryptionConfigs = javaType.encryptionConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.GetMetastoreServiceEncryptionConfig.Companion.toKotlin(args0)
})
}),
endpointUri = javaType.endpointUri(),
hiveMetastoreConfigs = javaType.hiveMetastoreConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.GetMetastoreServiceHiveMetastoreConfig.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
location = javaType.location(),
maintenanceWindows = javaType.maintenanceWindows().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.GetMetastoreServiceMaintenanceWindow.Companion.toKotlin(args0)
})
}),
metadataIntegrations = javaType.metadataIntegrations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.GetMetastoreServiceMetadataIntegration.Companion.toKotlin(args0)
})
}),
name = javaType.name(),
network = javaType.network(),
networkConfigs = javaType.networkConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.GetMetastoreServiceNetworkConfig.Companion.toKotlin(args0)
})
}),
port = javaType.port(),
project = javaType.project().map({ args0 -> args0 }).orElse(null),
pulumiLabels = javaType.pulumiLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
releaseChannel = javaType.releaseChannel(),
scalingConfigs = javaType.scalingConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.GetMetastoreServiceScalingConfig.Companion.toKotlin(args0)
})
}),
scheduledBackups = javaType.scheduledBackups().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.GetMetastoreServiceScheduledBackup.Companion.toKotlin(args0)
})
}),
serviceId = javaType.serviceId(),
state = javaType.state(),
stateMessage = javaType.stateMessage(),
telemetryConfigs = javaType.telemetryConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.GetMetastoreServiceTelemetryConfig.Companion.toKotlin(args0)
})
}),
tier = javaType.tier(),
uid = javaType.uid(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy