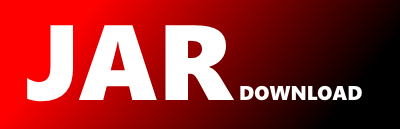
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataproc.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property autoscalingConfig Autoscaling config for the policy associated with the cluster. Cluster does not autoscale if this field is unset.
* @property encryptionConfig Encryption settings for the cluster.
* @property endpointConfig Port/endpoint configuration for this cluster
* @property gceClusterConfig The shared Compute Engine config settings for all instances in a cluster.
* @property gkeClusterConfig The Kubernetes Engine config for Dataproc clusters deployed to Kubernetes. Setting this is considered mutually exclusive with Compute Engine-based options such as `gce_cluster_config`, `master_config`, `worker_config`, `secondary_worker_config`, and `autoscaling_config`.
* @property initializationActions Commands to execute on each node after config is completed. By default, executables are run on master and all worker nodes. You can test a node's `role` metadata to run an executable on a master or worker node, as shown below using `curl` (you can also use `wget`): ROLE=$(curl -H Metadata-Flavor:Google http://metadata/computeMetadata/v1/instance/attributes/dataproc-role) if ; then ... master specific actions ... else ... worker specific actions ... fi
* @property lifecycleConfig Lifecycle setting for the cluster.
* @property masterConfig The Compute Engine config settings for additional worker instances in a cluster.
* @property metastoreConfig Metastore configuration.
* @property secondaryWorkerConfig The Compute Engine config settings for additional worker instances in a cluster.
* @property securityConfig Security settings for the cluster.
* @property softwareConfig The config settings for software inside the cluster.
* @property stagingBucket A Cloud Storage bucket used to stage job dependencies, config files, and job driver console output. If you do not specify a staging bucket, Cloud Dataproc will determine a Cloud Storage location (US, ASIA, or EU) for your cluster's staging bucket according to the Compute Engine zone where your cluster is deployed, and then create and manage this project-level, per-location bucket (see (https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* @property tempBucket A Cloud Storage bucket used to store ephemeral cluster and jobs data, such as Spark and MapReduce history files. If you do not specify a temp bucket, Dataproc will determine a Cloud Storage location (US, ASIA, or EU) for your cluster's temp bucket according to the Compute Engine zone where your cluster is deployed, and then create and manage this project-level, per-location bucket. The default bucket has a TTL of 90 days, but you can use any TTL (or none) if you specify a bucket.
* @property workerConfig The Compute Engine config settings for additional worker instances in a cluster.
* - - -
*/
public data class WorkflowTemplatePlacementManagedClusterConfig(
public val autoscalingConfig: WorkflowTemplatePlacementManagedClusterConfigAutoscalingConfig? =
null,
public val encryptionConfig: WorkflowTemplatePlacementManagedClusterConfigEncryptionConfig? =
null,
public val endpointConfig: WorkflowTemplatePlacementManagedClusterConfigEndpointConfig? = null,
public val gceClusterConfig: WorkflowTemplatePlacementManagedClusterConfigGceClusterConfig? =
null,
public val gkeClusterConfig: WorkflowTemplatePlacementManagedClusterConfigGkeClusterConfig? =
null,
public val initializationActions: List? = null,
public val lifecycleConfig: WorkflowTemplatePlacementManagedClusterConfigLifecycleConfig? = null,
public val masterConfig: WorkflowTemplatePlacementManagedClusterConfigMasterConfig? = null,
public val metastoreConfig: WorkflowTemplatePlacementManagedClusterConfigMetastoreConfig? = null,
public val secondaryWorkerConfig: WorkflowTemplatePlacementManagedClusterConfigSecondaryWorkerConfig? = null,
public val securityConfig: WorkflowTemplatePlacementManagedClusterConfigSecurityConfig? = null,
public val softwareConfig: WorkflowTemplatePlacementManagedClusterConfigSoftwareConfig? = null,
public val stagingBucket: String? = null,
public val tempBucket: String? = null,
public val workerConfig: WorkflowTemplatePlacementManagedClusterConfigWorkerConfig? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.dataproc.outputs.WorkflowTemplatePlacementManagedClusterConfig): WorkflowTemplatePlacementManagedClusterConfig =
WorkflowTemplatePlacementManagedClusterConfig(
autoscalingConfig = javaType.autoscalingConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigAutoscalingConfig.Companion.toKotlin(args0)
})
}).orElse(null),
encryptionConfig = javaType.encryptionConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigEncryptionConfig.Companion.toKotlin(args0)
})
}).orElse(null),
endpointConfig = javaType.endpointConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigEndpointConfig.Companion.toKotlin(args0)
})
}).orElse(null),
gceClusterConfig = javaType.gceClusterConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigGceClusterConfig.Companion.toKotlin(args0)
})
}).orElse(null),
gkeClusterConfig = javaType.gkeClusterConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigGkeClusterConfig.Companion.toKotlin(args0)
})
}).orElse(null),
initializationActions = javaType.initializationActions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigInitializationAction.Companion.toKotlin(args0)
})
}),
lifecycleConfig = javaType.lifecycleConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigLifecycleConfig.Companion.toKotlin(args0)
})
}).orElse(null),
masterConfig = javaType.masterConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigMasterConfig.Companion.toKotlin(args0)
})
}).orElse(null),
metastoreConfig = javaType.metastoreConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigMetastoreConfig.Companion.toKotlin(args0)
})
}).orElse(null),
secondaryWorkerConfig = javaType.secondaryWorkerConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigSecondaryWorkerConfig.Companion.toKotlin(args0)
})
}).orElse(null),
securityConfig = javaType.securityConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigSecurityConfig.Companion.toKotlin(args0)
})
}).orElse(null),
softwareConfig = javaType.softwareConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigSoftwareConfig.Companion.toKotlin(args0)
})
}).orElse(null),
stagingBucket = javaType.stagingBucket().map({ args0 -> args0 }).orElse(null),
tempBucket = javaType.tempBucket().map({ args0 -> args0 }).orElse(null),
workerConfig = javaType.workerConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.dataproc.kotlin.outputs.WorkflowTemplatePlacementManagedClusterConfigWorkerConfig.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy