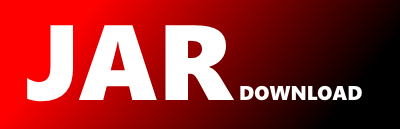
com.pulumi.gcp.datastream.kotlin.inputs.ConnectionProfileMysqlProfileSslConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.datastream.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.datastream.inputs.ConnectionProfileMysqlProfileSslConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property caCertificate PEM-encoded certificate of the CA that signed the source database
* server's certificate.
* **Note**: This property is sensitive and will not be displayed in the plan.
* @property caCertificateSet (Output)
* Indicates whether the clientKey field is set.
* @property clientCertificate PEM-encoded certificate that will be used by the replica to
* authenticate against the source database server. If this field
* is used then the 'clientKey' and the 'caCertificate' fields are
* mandatory.
* **Note**: This property is sensitive and will not be displayed in the plan.
* @property clientCertificateSet (Output)
* Indicates whether the clientCertificate field is set.
* @property clientKey PEM-encoded private key associated with the Client Certificate.
* If this field is used then the 'client_certificate' and the
* 'ca_certificate' fields are mandatory.
* **Note**: This property is sensitive and will not be displayed in the plan.
* @property clientKeySet (Output)
* Indicates whether the clientKey field is set.
*/
public data class ConnectionProfileMysqlProfileSslConfigArgs(
public val caCertificate: Output? = null,
public val caCertificateSet: Output? = null,
public val clientCertificate: Output? = null,
public val clientCertificateSet: Output? = null,
public val clientKey: Output? = null,
public val clientKeySet: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.datastream.inputs.ConnectionProfileMysqlProfileSslConfigArgs = com.pulumi.gcp.datastream.inputs.ConnectionProfileMysqlProfileSslConfigArgs.builder()
.caCertificate(caCertificate?.applyValue({ args0 -> args0 }))
.caCertificateSet(caCertificateSet?.applyValue({ args0 -> args0 }))
.clientCertificate(clientCertificate?.applyValue({ args0 -> args0 }))
.clientCertificateSet(clientCertificateSet?.applyValue({ args0 -> args0 }))
.clientKey(clientKey?.applyValue({ args0 -> args0 }))
.clientKeySet(clientKeySet?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConnectionProfileMysqlProfileSslConfigArgs].
*/
@PulumiTagMarker
public class ConnectionProfileMysqlProfileSslConfigArgsBuilder internal constructor() {
private var caCertificate: Output? = null
private var caCertificateSet: Output? = null
private var clientCertificate: Output? = null
private var clientCertificateSet: Output? = null
private var clientKey: Output? = null
private var clientKeySet: Output? = null
/**
* @param value PEM-encoded certificate of the CA that signed the source database
* server's certificate.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("qmwtqjfvucoxikpx")
public suspend fun caCertificate(`value`: Output) {
this.caCertificate = value
}
/**
* @param value (Output)
* Indicates whether the clientKey field is set.
*/
@JvmName("pkfvnatrtqiwctmu")
public suspend fun caCertificateSet(`value`: Output) {
this.caCertificateSet = value
}
/**
* @param value PEM-encoded certificate that will be used by the replica to
* authenticate against the source database server. If this field
* is used then the 'clientKey' and the 'caCertificate' fields are
* mandatory.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("lsryqtcucpxgdwyu")
public suspend fun clientCertificate(`value`: Output) {
this.clientCertificate = value
}
/**
* @param value (Output)
* Indicates whether the clientCertificate field is set.
*/
@JvmName("dvemenmcpesgdwsn")
public suspend fun clientCertificateSet(`value`: Output) {
this.clientCertificateSet = value
}
/**
* @param value PEM-encoded private key associated with the Client Certificate.
* If this field is used then the 'client_certificate' and the
* 'ca_certificate' fields are mandatory.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("lwhwofqsrhtqfokt")
public suspend fun clientKey(`value`: Output) {
this.clientKey = value
}
/**
* @param value (Output)
* Indicates whether the clientKey field is set.
*/
@JvmName("jlgqfajjonhuboyx")
public suspend fun clientKeySet(`value`: Output) {
this.clientKeySet = value
}
/**
* @param value PEM-encoded certificate of the CA that signed the source database
* server's certificate.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("rikhwtemibvdyeqd")
public suspend fun caCertificate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caCertificate = mapped
}
/**
* @param value (Output)
* Indicates whether the clientKey field is set.
*/
@JvmName("ovbjwqmhuinywhpd")
public suspend fun caCertificateSet(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caCertificateSet = mapped
}
/**
* @param value PEM-encoded certificate that will be used by the replica to
* authenticate against the source database server. If this field
* is used then the 'clientKey' and the 'caCertificate' fields are
* mandatory.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("mtelgtxilrcmvfki")
public suspend fun clientCertificate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientCertificate = mapped
}
/**
* @param value (Output)
* Indicates whether the clientCertificate field is set.
*/
@JvmName("ysgwljdslyfihttf")
public suspend fun clientCertificateSet(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientCertificateSet = mapped
}
/**
* @param value PEM-encoded private key associated with the Client Certificate.
* If this field is used then the 'client_certificate' and the
* 'ca_certificate' fields are mandatory.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("yodcmkjospjreaur")
public suspend fun clientKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientKey = mapped
}
/**
* @param value (Output)
* Indicates whether the clientKey field is set.
*/
@JvmName("mkykwejlwetejpyx")
public suspend fun clientKeySet(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientKeySet = mapped
}
internal fun build(): ConnectionProfileMysqlProfileSslConfigArgs =
ConnectionProfileMysqlProfileSslConfigArgs(
caCertificate = caCertificate,
caCertificateSet = caCertificateSet,
clientCertificate = clientCertificate,
clientCertificateSet = clientCertificateSet,
clientKey = clientKey,
clientKeySet = clientKeySet,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy