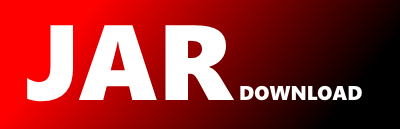
com.pulumi.gcp.datastream.kotlin.inputs.StreamDestinationConfigBigqueryDestinationConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.datastream.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.datastream.inputs.StreamDestinationConfigBigqueryDestinationConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property dataFreshness The guaranteed data freshness (in seconds) when querying tables created by the stream.
* Editing this field will only affect new tables created in the future, but existing tables
* will not be impacted. Lower values mean that queries will return fresher data, but may result in higher cost.
* A duration in seconds with up to nine fractional digits, terminated by 's'. Example: "3.5s". Defaults to 900s.
* @property singleTargetDataset A single target dataset to which all data will be streamed.
* Structure is documented below.
* @property sourceHierarchyDatasets Destination datasets are created so that hierarchy of the destination data objects matches the source hierarchy.
* Structure is documented below.
*/
public data class StreamDestinationConfigBigqueryDestinationConfigArgs(
public val dataFreshness: Output? = null,
public val singleTargetDataset: Output? = null,
public val sourceHierarchyDatasets: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.datastream.inputs.StreamDestinationConfigBigqueryDestinationConfigArgs =
com.pulumi.gcp.datastream.inputs.StreamDestinationConfigBigqueryDestinationConfigArgs.builder()
.dataFreshness(dataFreshness?.applyValue({ args0 -> args0 }))
.singleTargetDataset(
singleTargetDataset?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sourceHierarchyDatasets(
sourceHierarchyDatasets?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [StreamDestinationConfigBigqueryDestinationConfigArgs].
*/
@PulumiTagMarker
public class StreamDestinationConfigBigqueryDestinationConfigArgsBuilder internal constructor() {
private var dataFreshness: Output? = null
private var singleTargetDataset:
Output? = null
private var sourceHierarchyDatasets:
Output? = null
/**
* @param value The guaranteed data freshness (in seconds) when querying tables created by the stream.
* Editing this field will only affect new tables created in the future, but existing tables
* will not be impacted. Lower values mean that queries will return fresher data, but may result in higher cost.
* A duration in seconds with up to nine fractional digits, terminated by 's'. Example: "3.5s". Defaults to 900s.
*/
@JvmName("wfuxiqaikacfercu")
public suspend fun dataFreshness(`value`: Output) {
this.dataFreshness = value
}
/**
* @param value A single target dataset to which all data will be streamed.
* Structure is documented below.
*/
@JvmName("ktuhcotuxniagvvh")
public suspend fun singleTargetDataset(`value`: Output) {
this.singleTargetDataset = value
}
/**
* @param value Destination datasets are created so that hierarchy of the destination data objects matches the source hierarchy.
* Structure is documented below.
*/
@JvmName("oibjogstimrcxruc")
public suspend fun sourceHierarchyDatasets(`value`: Output) {
this.sourceHierarchyDatasets = value
}
/**
* @param value The guaranteed data freshness (in seconds) when querying tables created by the stream.
* Editing this field will only affect new tables created in the future, but existing tables
* will not be impacted. Lower values mean that queries will return fresher data, but may result in higher cost.
* A duration in seconds with up to nine fractional digits, terminated by 's'. Example: "3.5s". Defaults to 900s.
*/
@JvmName("ysmwmkhdjutitioq")
public suspend fun dataFreshness(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataFreshness = mapped
}
/**
* @param value A single target dataset to which all data will be streamed.
* Structure is documented below.
*/
@JvmName("odsxhxmcxsodwcqm")
public suspend fun singleTargetDataset(`value`: StreamDestinationConfigBigqueryDestinationConfigSingleTargetDatasetArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.singleTargetDataset = mapped
}
/**
* @param argument A single target dataset to which all data will be streamed.
* Structure is documented below.
*/
@JvmName("ulhymijiwofyyyot")
public suspend fun singleTargetDataset(argument: suspend StreamDestinationConfigBigqueryDestinationConfigSingleTargetDatasetArgsBuilder.() -> Unit) {
val toBeMapped =
StreamDestinationConfigBigqueryDestinationConfigSingleTargetDatasetArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.singleTargetDataset = mapped
}
/**
* @param value Destination datasets are created so that hierarchy of the destination data objects matches the source hierarchy.
* Structure is documented below.
*/
@JvmName("plxyjbwsrdtiynwc")
public suspend fun sourceHierarchyDatasets(`value`: StreamDestinationConfigBigqueryDestinationConfigSourceHierarchyDatasetsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceHierarchyDatasets = mapped
}
/**
* @param argument Destination datasets are created so that hierarchy of the destination data objects matches the source hierarchy.
* Structure is documented below.
*/
@JvmName("flqvyuygxhwxyfyl")
public suspend fun sourceHierarchyDatasets(argument: suspend StreamDestinationConfigBigqueryDestinationConfigSourceHierarchyDatasetsArgsBuilder.() -> Unit) {
val toBeMapped =
StreamDestinationConfigBigqueryDestinationConfigSourceHierarchyDatasetsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sourceHierarchyDatasets = mapped
}
internal fun build(): StreamDestinationConfigBigqueryDestinationConfigArgs =
StreamDestinationConfigBigqueryDestinationConfigArgs(
dataFreshness = dataFreshness,
singleTargetDataset = singleTargetDataset,
sourceHierarchyDatasets = sourceHierarchyDatasets,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy