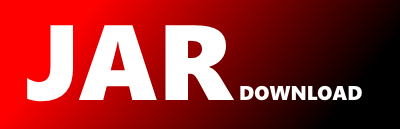
com.pulumi.gcp.datastream.kotlin.inputs.StreamSourceConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.datastream.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.datastream.inputs.StreamSourceConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property mysqlSourceConfig MySQL data source configuration.
* Structure is documented below.
* @property oracleSourceConfig MySQL data source configuration.
* Structure is documented below.
* @property postgresqlSourceConfig PostgreSQL data source configuration.
* Structure is documented below.
* @property sourceConnectionProfile Source connection profile resource. Format: projects/{project}/locations/{location}/connectionProfiles/{name}
* @property sqlServerSourceConfig SQL Server data source configuration.
* Structure is documented below.
*/
public data class StreamSourceConfigArgs(
public val mysqlSourceConfig: Output? = null,
public val oracleSourceConfig: Output? = null,
public val postgresqlSourceConfig: Output? = null,
public val sourceConnectionProfile: Output,
public val sqlServerSourceConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.datastream.inputs.StreamSourceConfigArgs =
com.pulumi.gcp.datastream.inputs.StreamSourceConfigArgs.builder()
.mysqlSourceConfig(mysqlSourceConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.oracleSourceConfig(
oracleSourceConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.postgresqlSourceConfig(
postgresqlSourceConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sourceConnectionProfile(sourceConnectionProfile.applyValue({ args0 -> args0 }))
.sqlServerSourceConfig(
sqlServerSourceConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [StreamSourceConfigArgs].
*/
@PulumiTagMarker
public class StreamSourceConfigArgsBuilder internal constructor() {
private var mysqlSourceConfig: Output? = null
private var oracleSourceConfig: Output? = null
private var postgresqlSourceConfig: Output? = null
private var sourceConnectionProfile: Output? = null
private var sqlServerSourceConfig: Output? = null
/**
* @param value MySQL data source configuration.
* Structure is documented below.
*/
@JvmName("oyvoxwlqdkalewbo")
public suspend fun mysqlSourceConfig(`value`: Output) {
this.mysqlSourceConfig = value
}
/**
* @param value MySQL data source configuration.
* Structure is documented below.
*/
@JvmName("qyqwteuubiibdolv")
public suspend fun oracleSourceConfig(`value`: Output) {
this.oracleSourceConfig = value
}
/**
* @param value PostgreSQL data source configuration.
* Structure is documented below.
*/
@JvmName("lhbmtxceinjexxev")
public suspend fun postgresqlSourceConfig(`value`: Output) {
this.postgresqlSourceConfig = value
}
/**
* @param value Source connection profile resource. Format: projects/{project}/locations/{location}/connectionProfiles/{name}
*/
@JvmName("chluewjxvhorsibk")
public suspend fun sourceConnectionProfile(`value`: Output) {
this.sourceConnectionProfile = value
}
/**
* @param value SQL Server data source configuration.
* Structure is documented below.
*/
@JvmName("bpcupcfxvbwlmtjh")
public suspend fun sqlServerSourceConfig(`value`: Output) {
this.sqlServerSourceConfig = value
}
/**
* @param value MySQL data source configuration.
* Structure is documented below.
*/
@JvmName("nxhwblecjpbkhcnf")
public suspend fun mysqlSourceConfig(`value`: StreamSourceConfigMysqlSourceConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mysqlSourceConfig = mapped
}
/**
* @param argument MySQL data source configuration.
* Structure is documented below.
*/
@JvmName("pomfhdingvclxcbr")
public suspend fun mysqlSourceConfig(argument: suspend StreamSourceConfigMysqlSourceConfigArgsBuilder.() -> Unit) {
val toBeMapped = StreamSourceConfigMysqlSourceConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.mysqlSourceConfig = mapped
}
/**
* @param value MySQL data source configuration.
* Structure is documented below.
*/
@JvmName("rskxjialsnrlsxsq")
public suspend fun oracleSourceConfig(`value`: StreamSourceConfigOracleSourceConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.oracleSourceConfig = mapped
}
/**
* @param argument MySQL data source configuration.
* Structure is documented below.
*/
@JvmName("pkewtlpsgedvrgkm")
public suspend fun oracleSourceConfig(argument: suspend StreamSourceConfigOracleSourceConfigArgsBuilder.() -> Unit) {
val toBeMapped = StreamSourceConfigOracleSourceConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.oracleSourceConfig = mapped
}
/**
* @param value PostgreSQL data source configuration.
* Structure is documented below.
*/
@JvmName("fvwkcotmquypiujg")
public suspend fun postgresqlSourceConfig(`value`: StreamSourceConfigPostgresqlSourceConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postgresqlSourceConfig = mapped
}
/**
* @param argument PostgreSQL data source configuration.
* Structure is documented below.
*/
@JvmName("nxbnpyblqxblcmca")
public suspend fun postgresqlSourceConfig(argument: suspend StreamSourceConfigPostgresqlSourceConfigArgsBuilder.() -> Unit) {
val toBeMapped = StreamSourceConfigPostgresqlSourceConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.postgresqlSourceConfig = mapped
}
/**
* @param value Source connection profile resource. Format: projects/{project}/locations/{location}/connectionProfiles/{name}
*/
@JvmName("blvtjcbokfiovfta")
public suspend fun sourceConnectionProfile(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceConnectionProfile = mapped
}
/**
* @param value SQL Server data source configuration.
* Structure is documented below.
*/
@JvmName("wfcgjowoxhhftrje")
public suspend fun sqlServerSourceConfig(`value`: StreamSourceConfigSqlServerSourceConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqlServerSourceConfig = mapped
}
/**
* @param argument SQL Server data source configuration.
* Structure is documented below.
*/
@JvmName("gjlwxtpchaqjhjrm")
public suspend fun sqlServerSourceConfig(argument: suspend StreamSourceConfigSqlServerSourceConfigArgsBuilder.() -> Unit) {
val toBeMapped = StreamSourceConfigSqlServerSourceConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sqlServerSourceConfig = mapped
}
internal fun build(): StreamSourceConfigArgs = StreamSourceConfigArgs(
mysqlSourceConfig = mysqlSourceConfig,
oracleSourceConfig = oracleSourceConfig,
postgresqlSourceConfig = postgresqlSourceConfig,
sourceConnectionProfile = sourceConnectionProfile ?: throw
PulumiNullFieldException("sourceConnectionProfile"),
sqlServerSourceConfig = sqlServerSourceConfig,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy