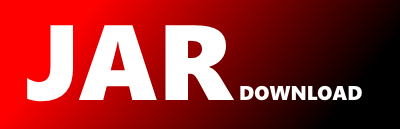
com.pulumi.gcp.datastream.kotlin.inputs.StreamSourceConfigPostgresqlSourceConfigIncludeObjectsPostgresqlSchemaPostgresqlTablePostgresqlColumnArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.datastream.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.datastream.inputs.StreamSourceConfigPostgresqlSourceConfigIncludeObjectsPostgresqlSchemaPostgresqlTablePostgresqlColumnArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property column Column name.
* @property dataType The PostgreSQL data type. Full data types list can be found here:
* https://www.postgresql.org/docs/current/datatype.html
* @property length (Output)
* Column length.
* @property nullable Whether or not the column can accept a null value.
* @property ordinalPosition The ordinal position of the column in the table.
* @property precision (Output)
* Column precision.
* @property primaryKey Whether or not the column represents a primary key.
* @property scale (Output)
* Column scale.
*/
public data class
StreamSourceConfigPostgresqlSourceConfigIncludeObjectsPostgresqlSchemaPostgresqlTablePostgresqlColumnArgs(
public val column: Output? = null,
public val dataType: Output? = null,
public val length: Output? = null,
public val nullable: Output? = null,
public val ordinalPosition: Output? = null,
public val precision: Output? = null,
public val primaryKey: Output? = null,
public val scale: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.datastream.inputs.StreamSourceConfigPostgresqlSourceConfigIncludeObjectsPostgresqlSchemaPostgresqlTablePostgresqlColumnArgs =
com.pulumi.gcp.datastream.inputs.StreamSourceConfigPostgresqlSourceConfigIncludeObjectsPostgresqlSchemaPostgresqlTablePostgresqlColumnArgs.builder()
.column(column?.applyValue({ args0 -> args0 }))
.dataType(dataType?.applyValue({ args0 -> args0 }))
.length(length?.applyValue({ args0 -> args0 }))
.nullable(nullable?.applyValue({ args0 -> args0 }))
.ordinalPosition(ordinalPosition?.applyValue({ args0 -> args0 }))
.precision(precision?.applyValue({ args0 -> args0 }))
.primaryKey(primaryKey?.applyValue({ args0 -> args0 }))
.scale(scale?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [StreamSourceConfigPostgresqlSourceConfigIncludeObjectsPostgresqlSchemaPostgresqlTablePostgresqlColumnArgs].
*/
@PulumiTagMarker
public class
StreamSourceConfigPostgresqlSourceConfigIncludeObjectsPostgresqlSchemaPostgresqlTablePostgresqlColumnArgsBuilder
internal constructor() {
private var column: Output? = null
private var dataType: Output? = null
private var length: Output? = null
private var nullable: Output? = null
private var ordinalPosition: Output? = null
private var precision: Output? = null
private var primaryKey: Output? = null
private var scale: Output? = null
/**
* @param value Column name.
*/
@JvmName("odjddcywxxnpkixo")
public suspend fun column(`value`: Output) {
this.column = value
}
/**
* @param value The PostgreSQL data type. Full data types list can be found here:
* https://www.postgresql.org/docs/current/datatype.html
*/
@JvmName("pypjkfwaoeedefpy")
public suspend fun dataType(`value`: Output) {
this.dataType = value
}
/**
* @param value (Output)
* Column length.
*/
@JvmName("kxfnbvfjiuusqydj")
public suspend fun length(`value`: Output) {
this.length = value
}
/**
* @param value Whether or not the column can accept a null value.
*/
@JvmName("celqriaogxnnqsfs")
public suspend fun nullable(`value`: Output) {
this.nullable = value
}
/**
* @param value The ordinal position of the column in the table.
*/
@JvmName("bewidvgtkuveonjl")
public suspend fun ordinalPosition(`value`: Output) {
this.ordinalPosition = value
}
/**
* @param value (Output)
* Column precision.
*/
@JvmName("brqugiukrixlujtx")
public suspend fun precision(`value`: Output) {
this.precision = value
}
/**
* @param value Whether or not the column represents a primary key.
*/
@JvmName("pdhkcgenbsgcyaje")
public suspend fun primaryKey(`value`: Output) {
this.primaryKey = value
}
/**
* @param value (Output)
* Column scale.
*/
@JvmName("bvflivgmotuwklgc")
public suspend fun scale(`value`: Output) {
this.scale = value
}
/**
* @param value Column name.
*/
@JvmName("aivoknemrouodiwe")
public suspend fun column(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.column = mapped
}
/**
* @param value The PostgreSQL data type. Full data types list can be found here:
* https://www.postgresql.org/docs/current/datatype.html
*/
@JvmName("gejwtabgvoiaeajw")
public suspend fun dataType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataType = mapped
}
/**
* @param value (Output)
* Column length.
*/
@JvmName("onmdojivdhtmhorj")
public suspend fun length(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.length = mapped
}
/**
* @param value Whether or not the column can accept a null value.
*/
@JvmName("polmqokwolclkaah")
public suspend fun nullable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nullable = mapped
}
/**
* @param value The ordinal position of the column in the table.
*/
@JvmName("jbdeevvthlpfhfbc")
public suspend fun ordinalPosition(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ordinalPosition = mapped
}
/**
* @param value (Output)
* Column precision.
*/
@JvmName("gbcshogbygsawpgu")
public suspend fun precision(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.precision = mapped
}
/**
* @param value Whether or not the column represents a primary key.
*/
@JvmName("lthkaeflnhmveofk")
public suspend fun primaryKey(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.primaryKey = mapped
}
/**
* @param value (Output)
* Column scale.
*/
@JvmName("bfbgovpxoknhgsuu")
public suspend fun scale(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scale = mapped
}
internal fun build(): StreamSourceConfigPostgresqlSourceConfigIncludeObjectsPostgresqlSchemaPostgresqlTablePostgresqlColumnArgs =
StreamSourceConfigPostgresqlSourceConfigIncludeObjectsPostgresqlSchemaPostgresqlTablePostgresqlColumnArgs(
column = column,
dataType = dataType,
length = length,
nullable = nullable,
ordinalPosition = ordinalPosition,
precision = precision,
primaryKey = primaryKey,
scale = scale,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy