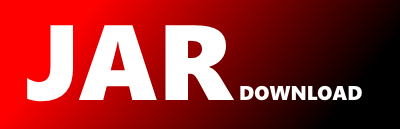
com.pulumi.gcp.datastream.kotlin.inputs.StreamSourceConfigSqlServerSourceConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.datastream.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.datastream.inputs.StreamSourceConfigSqlServerSourceConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property excludeObjects SQL Server objects to exclude from the stream.
* Structure is documented below.
* @property includeObjects SQL Server objects to retrieve from the source.
* Structure is documented below.
* @property maxConcurrentBackfillTasks Max concurrent backfill tasks.
* @property maxConcurrentCdcTasks Max concurrent CDC tasks.
*/
public data class StreamSourceConfigSqlServerSourceConfigArgs(
public val excludeObjects: Output? =
null,
public val includeObjects: Output? =
null,
public val maxConcurrentBackfillTasks: Output? = null,
public val maxConcurrentCdcTasks: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.datastream.inputs.StreamSourceConfigSqlServerSourceConfigArgs =
com.pulumi.gcp.datastream.inputs.StreamSourceConfigSqlServerSourceConfigArgs.builder()
.excludeObjects(excludeObjects?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.includeObjects(includeObjects?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maxConcurrentBackfillTasks(maxConcurrentBackfillTasks?.applyValue({ args0 -> args0 }))
.maxConcurrentCdcTasks(maxConcurrentCdcTasks?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [StreamSourceConfigSqlServerSourceConfigArgs].
*/
@PulumiTagMarker
public class StreamSourceConfigSqlServerSourceConfigArgsBuilder internal constructor() {
private var excludeObjects: Output? =
null
private var includeObjects: Output? =
null
private var maxConcurrentBackfillTasks: Output? = null
private var maxConcurrentCdcTasks: Output? = null
/**
* @param value SQL Server objects to exclude from the stream.
* Structure is documented below.
*/
@JvmName("fedisxuiqvwjlflc")
public suspend fun excludeObjects(`value`: Output) {
this.excludeObjects = value
}
/**
* @param value SQL Server objects to retrieve from the source.
* Structure is documented below.
*/
@JvmName("hwvbgodxqrciysps")
public suspend fun includeObjects(`value`: Output) {
this.includeObjects = value
}
/**
* @param value Max concurrent backfill tasks.
*/
@JvmName("usuqartxavhpqqtr")
public suspend fun maxConcurrentBackfillTasks(`value`: Output) {
this.maxConcurrentBackfillTasks = value
}
/**
* @param value Max concurrent CDC tasks.
*/
@JvmName("ondgxsuqyjjafuim")
public suspend fun maxConcurrentCdcTasks(`value`: Output) {
this.maxConcurrentCdcTasks = value
}
/**
* @param value SQL Server objects to exclude from the stream.
* Structure is documented below.
*/
@JvmName("crnasetrrpoonnsv")
public suspend fun excludeObjects(`value`: StreamSourceConfigSqlServerSourceConfigExcludeObjectsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.excludeObjects = mapped
}
/**
* @param argument SQL Server objects to exclude from the stream.
* Structure is documented below.
*/
@JvmName("ibufiyyogbwgvtbt")
public suspend fun excludeObjects(argument: suspend StreamSourceConfigSqlServerSourceConfigExcludeObjectsArgsBuilder.() -> Unit) {
val toBeMapped =
StreamSourceConfigSqlServerSourceConfigExcludeObjectsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.excludeObjects = mapped
}
/**
* @param value SQL Server objects to retrieve from the source.
* Structure is documented below.
*/
@JvmName("vqkwvyagmbymohnv")
public suspend fun includeObjects(`value`: StreamSourceConfigSqlServerSourceConfigIncludeObjectsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeObjects = mapped
}
/**
* @param argument SQL Server objects to retrieve from the source.
* Structure is documented below.
*/
@JvmName("vreulagmgtrwaixn")
public suspend fun includeObjects(argument: suspend StreamSourceConfigSqlServerSourceConfigIncludeObjectsArgsBuilder.() -> Unit) {
val toBeMapped =
StreamSourceConfigSqlServerSourceConfigIncludeObjectsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.includeObjects = mapped
}
/**
* @param value Max concurrent backfill tasks.
*/
@JvmName("thichyeaangpogmt")
public suspend fun maxConcurrentBackfillTasks(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConcurrentBackfillTasks = mapped
}
/**
* @param value Max concurrent CDC tasks.
*/
@JvmName("feiujsjaometsujr")
public suspend fun maxConcurrentCdcTasks(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConcurrentCdcTasks = mapped
}
internal fun build(): StreamSourceConfigSqlServerSourceConfigArgs =
StreamSourceConfigSqlServerSourceConfigArgs(
excludeObjects = excludeObjects,
includeObjects = includeObjects,
maxConcurrentBackfillTasks = maxConcurrentBackfillTasks,
maxConcurrentCdcTasks = maxConcurrentCdcTasks,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy