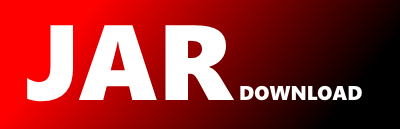
com.pulumi.gcp.diagflow.kotlin.Agent.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.diagflow.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [Agent].
*/
@PulumiTagMarker
public class AgentResourceBuilder internal constructor() {
public var name: String? = null
public var args: AgentArgs = AgentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AgentArgsBuilder.() -> Unit) {
val builder = AgentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Agent {
val builtJavaResource = com.pulumi.gcp.diagflow.Agent(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Agent(builtJavaResource)
}
}
/**
* A Dialogflow agent is a virtual agent that handles conversations with your end-users. It is a natural language
* understanding module that understands the nuances of human language. Dialogflow translates end-user text or audio
* during a conversation to structured data that your apps and services can understand. You design and build a Dialogflow
* agent to handle the types of conversations required for your system.
* To get more information about Agent, see:
* * [API documentation](https://cloud.google.com/dialogflow/docs/reference/rest/v2/projects/agent)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/dialogflow/docs/)
* ## Example Usage
* ### Dialogflow Agent Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const fullAgent = new gcp.diagflow.Agent("full_agent", {
* displayName: "dialogflow-agent",
* defaultLanguageCode: "en",
* supportedLanguageCodes: [
* "fr",
* "de",
* "es",
* ],
* timeZone: "America/New_York",
* description: "Example description.",
* avatarUri: "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enableLogging: true,
* matchMode: "MATCH_MODE_ML_ONLY",
* classificationThreshold: 0.3,
* apiVersion: "API_VERSION_V2_BETA_1",
* tier: "TIER_STANDARD",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* full_agent = gcp.diagflow.Agent("full_agent",
* display_name="dialogflow-agent",
* default_language_code="en",
* supported_language_codes=[
* "fr",
* "de",
* "es",
* ],
* time_zone="America/New_York",
* description="Example description.",
* avatar_uri="https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enable_logging=True,
* match_mode="MATCH_MODE_ML_ONLY",
* classification_threshold=0.3,
* api_version="API_VERSION_V2_BETA_1",
* tier="TIER_STANDARD")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var fullAgent = new Gcp.Diagflow.Agent("full_agent", new()
* {
* DisplayName = "dialogflow-agent",
* DefaultLanguageCode = "en",
* SupportedLanguageCodes = new[]
* {
* "fr",
* "de",
* "es",
* },
* TimeZone = "America/New_York",
* Description = "Example description.",
* AvatarUri = "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* EnableLogging = true,
* MatchMode = "MATCH_MODE_ML_ONLY",
* ClassificationThreshold = 0.3,
* ApiVersion = "API_VERSION_V2_BETA_1",
* Tier = "TIER_STANDARD",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/diagflow"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := diagflow.NewAgent(ctx, "full_agent", &diagflow.AgentArgs{
* DisplayName: pulumi.String("dialogflow-agent"),
* DefaultLanguageCode: pulumi.String("en"),
* SupportedLanguageCodes: pulumi.StringArray{
* pulumi.String("fr"),
* pulumi.String("de"),
* pulumi.String("es"),
* },
* TimeZone: pulumi.String("America/New_York"),
* Description: pulumi.String("Example description."),
* AvatarUri: pulumi.String("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png"),
* EnableLogging: pulumi.Bool(true),
* MatchMode: pulumi.String("MATCH_MODE_ML_ONLY"),
* ClassificationThreshold: pulumi.Float64(0.3),
* ApiVersion: pulumi.String("API_VERSION_V2_BETA_1"),
* Tier: pulumi.String("TIER_STANDARD"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.diagflow.Agent;
* import com.pulumi.gcp.diagflow.AgentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var fullAgent = new Agent("fullAgent", AgentArgs.builder()
* .displayName("dialogflow-agent")
* .defaultLanguageCode("en")
* .supportedLanguageCodes(
* "fr",
* "de",
* "es")
* .timeZone("America/New_York")
* .description("Example description.")
* .avatarUri("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png")
* .enableLogging(true)
* .matchMode("MATCH_MODE_ML_ONLY")
* .classificationThreshold(0.3)
* .apiVersion("API_VERSION_V2_BETA_1")
* .tier("TIER_STANDARD")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* fullAgent:
* type: gcp:diagflow:Agent
* name: full_agent
* properties:
* displayName: dialogflow-agent
* defaultLanguageCode: en
* supportedLanguageCodes:
* - fr
* - de
* - es
* timeZone: America/New_York
* description: Example description.
* avatarUri: https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png
* enableLogging: true
* matchMode: MATCH_MODE_ML_ONLY
* classificationThreshold: 0.3
* apiVersion: API_VERSION_V2_BETA_1
* tier: TIER_STANDARD
* ```
*
* ## Import
* Agent can be imported using any of these accepted formats:
* * `{{project}}`
* When using the `pulumi import` command, Agent can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:diagflow/agent:Agent default {{project}}
* ```
*/
public class Agent internal constructor(
override val javaResource: com.pulumi.gcp.diagflow.Agent,
) : KotlinCustomResource(javaResource, AgentMapper) {
/**
* API version displayed in Dialogflow console. If not specified, V2 API is assumed. Clients are free to query
* different service endpoints for different API versions. However, bots connectors and webhook calls will follow
* the specified API version.
* * API_VERSION_V1: Legacy V1 API.
* * API_VERSION_V2: V2 API.
* * API_VERSION_V2_BETA_1: V2beta1 API.
* Possible values are: `API_VERSION_V1`, `API_VERSION_V2`, `API_VERSION_V2_BETA_1`.
*/
public val apiVersion: Output
get() = javaResource.apiVersion().applyValue({ args0 -> args0 })
/**
* The URI of the agent's avatar, which are used throughout the Dialogflow console. When an image URL is entered
* into this field, the Dialogflow will save the image in the backend. The address of the backend image returned
* from the API will be shown in the [avatarUriBackend] field.
*/
public val avatarUri: Output?
get() = javaResource.avatarUri().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The URI of the agent's avatar as returned from the API. Output only. To provide an image URL for the agent avatar,
* the [avatarUri] field can be used.
*/
public val avatarUriBackend: Output
get() = javaResource.avatarUriBackend().applyValue({ args0 -> args0 })
/**
* To filter out false positive results and still get variety in matched natural language inputs for your agent,
* you can tune the machine learning classification threshold. If the returned score value is less than the threshold
* value, then a fallback intent will be triggered or, if there are no fallback intents defined, no intent will be
* triggered. The score values range from 0.0 (completely uncertain) to 1.0 (completely certain). If set to 0.0, the
* default of 0.3 is used.
*/
public val classificationThreshold: Output?
get() = javaResource.classificationThreshold().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The default language of the agent as a language tag. [See Language Support](https://cloud.google.com/dialogflow/docs/reference/language)
* for a list of the currently supported language codes. This field cannot be updated after creation.
*/
public val defaultLanguageCode: Output
get() = javaResource.defaultLanguageCode().applyValue({ args0 -> args0 })
/**
* The description of this agent. The maximum length is 500 characters. If exceeded, the request is rejected.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of this agent.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* Determines whether this agent should log conversation queries.
*/
public val enableLogging: Output?
get() = javaResource.enableLogging().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Determines how intents are detected from user queries.
* * MATCH_MODE_HYBRID: Best for agents with a small number of examples in intents and/or wide use of templates
* syntax and composite entities.
* * MATCH_MODE_ML_ONLY: Can be used for agents with a large number of examples in intents, especially the ones
* using @sys.any or very large developer entities.
* Possible values are: `MATCH_MODE_HYBRID`, `MATCH_MODE_ML_ONLY`.
*/
public val matchMode: Output
get() = javaResource.matchMode().applyValue({ args0 -> args0 })
/**
* The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The list of all languages supported by this agent (except for the defaultLanguageCode).
*/
public val supportedLanguageCodes: Output>?
get() = javaResource.supportedLanguageCodes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The agent tier. If not specified, TIER_STANDARD is assumed.
* * TIER_STANDARD: Standard tier.
* * TIER_ENTERPRISE: Enterprise tier (Essentials).
* * TIER_ENTERPRISE_PLUS: Enterprise tier (Plus).
* NOTE: Due to consistency issues, the provider will not read this field from the API. Drift is possible between
* the the provider state and Dialogflow if the agent tier is changed outside of the provider.
*/
public val tier: Output?
get() = javaResource.tier().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The time zone of this agent from the [time zone database](https://www.iana.org/time-zones), e.g., America/New_York,
* Europe/Paris.
* - - -
*/
public val timeZone: Output
get() = javaResource.timeZone().applyValue({ args0 -> args0 })
}
public object AgentMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.diagflow.Agent::class == javaResource::class
override fun map(javaResource: Resource): Agent = Agent(
javaResource as
com.pulumi.gcp.diagflow.Agent,
)
}
/**
* @see [Agent].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Agent].
*/
public suspend fun agent(name: String, block: suspend AgentResourceBuilder.() -> Unit): Agent {
val builder = AgentResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Agent].
* @param name The _unique_ name of the resulting resource.
*/
public fun agent(name: String): Agent {
val builder = AgentResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy